JavaScript Fundamentals: Learning the Language of the Web
AddEventListener() in Javascript
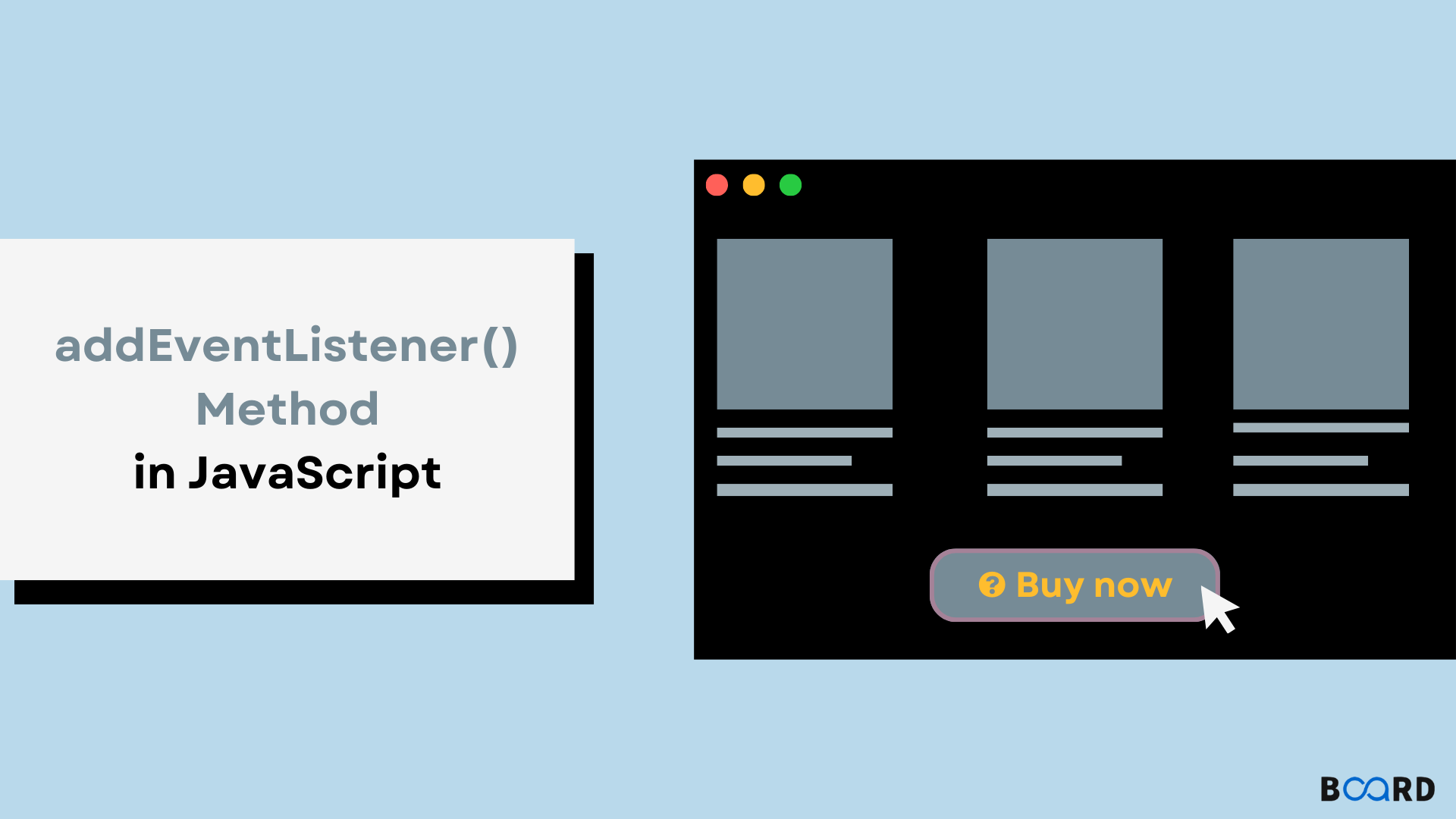
Introduction
An event handler may be attached to a specific element using the addEventListener() function. It does not take the place of the current event handlers. According to some, events are a crucial component of JavaScript. A web page reacts to an event in accordance with that occurrence. Events may be created by users or by APIs. A JavaScript process that watches for the occurrence of an event is known as an event listener.
JavaScript includes the addEventListener() method as a standard feature. A certain element can have numerous event handlers added to it without removing the previous ones.
Syntax
Parameter Values
- event: It is a necessary parameter, according to parameter values. It may be described as a string containing the event's name.
- function: This is a necessary input. It is a JavaScript function that reacts to the occurrence of the event.
- useCapture: This qualifier is optional. The value of the Boolean type indicates whether the event is carried out during the bubbling or capturing phase. True and false are the two possible values. The event handler runs in the capturing phase when it is set to true. The handler runs during the bubbling phase if it is set to false. False is its default value.
Return value
None (undefined)
Example
Output:
Code Explanation
When the button element in the example above is clicked, only secondFunction() will be called since assigning the second event handler replaces the first.
It is impossible to attach more than one event handler to a single event on a single element, which is the fundamental drawback of the traditional event paradigm. Event listeners, a more flexible event-model, was created by W3C to address this issue.
Conclusion
On the EventTarget on which it is called, the method addEventListener() adds a function or an object that implements EventListener to the list of event listeners for the provided event type. The function or object is not added a second time if it already exists in this target's list of event listeners.
You have control over the execution stage using addEventListener. With onClick, capturing and bubbling are difficult tasks. The addition of numerous events to an element is also possible using addEventListener. The addition of several handlers to an event is also possible. JavaScript modules and libraries both operate nicely with it.