JavaScript Fundamentals: Learning the Language of the Web
JavaScript Object.assign()
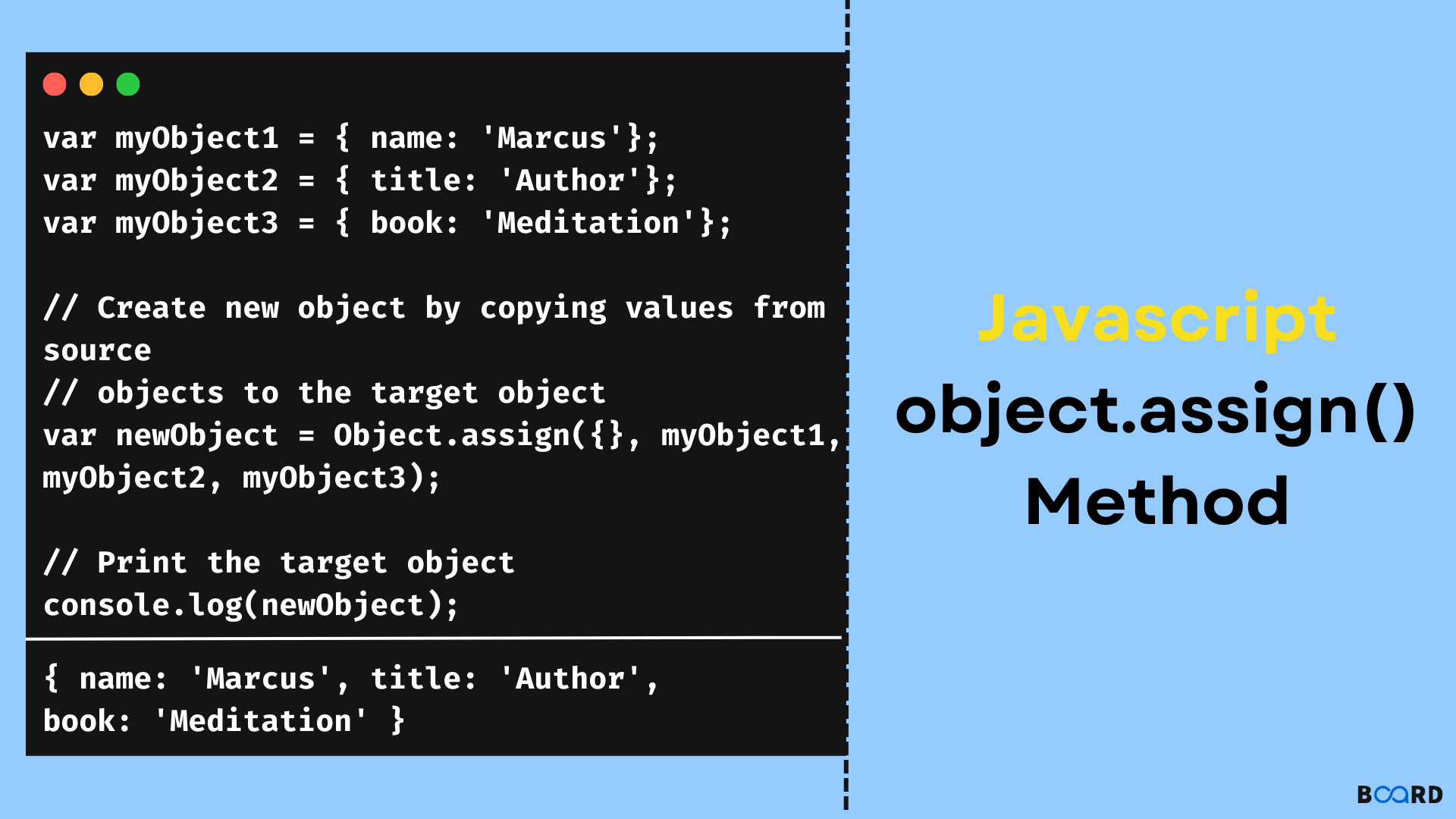
Introduction
In this article, we will discuss the object.assign() method in Javascript.
This method calls both: getter and setter as it makes use of both [[Get]] on the source and [[Set]] on the target. The return type of this method is the target object that contains properties and values obtained from the source object. This method doesn’t throw any null value.
Applications
Some of its applications are the following:
- We can clone objects using this function.
- We can merge those objects that share the same properties.
Syntax
Parameters
Return Type
Errors and exceptions
- If the property is non-writable then a TypeError is raised.
- Object.assign() doesn’t accept any null or undefined source values.
Let us consider the following program illustrating the working of this method:
Source Codes
Source Code 1
In the following program, we have created an object (myObject) having three properties as data1, data2, and data3. Using the assign method we are copying the source object into the target object.
Output:
Output Description:
As you can see in the output, the source with all the properties have been copied to the target object.
It is important to note here that we can copy multiple source objects into a single target object. For example,
Source Code 2
In the following program, we have created three objects: myObject1, myObject2, and myObject3. All these objects contain only one property. Using the assign method we are copying these source objects into the target object.
Output:
Output Description:
As you can see in the output, three source objects have been combined copied to the target object.
Now you must be wondering what happens if two objects have some properties in common. Let us consider the following program:
Source Code 3
In the following program, we have created three objects: myObject1 (having data1 as property), myObject2(having data1 and data2 as property), and myObject3(having data3 as property). Using the assign method we are copying these source objects into the target object.
Output:
Output Description:
As you can see in the output, the value of 100 was assigned to data1 firstly then it was overwritten to 300 while declaring object2.
Conclusion
In this article, we have discussed Object.assign() function and its working in Javascript. We hope this has strengthened your knowledge in the field of Javascript.