Java Advanced Topics and Techniques
Abstract Class in Java
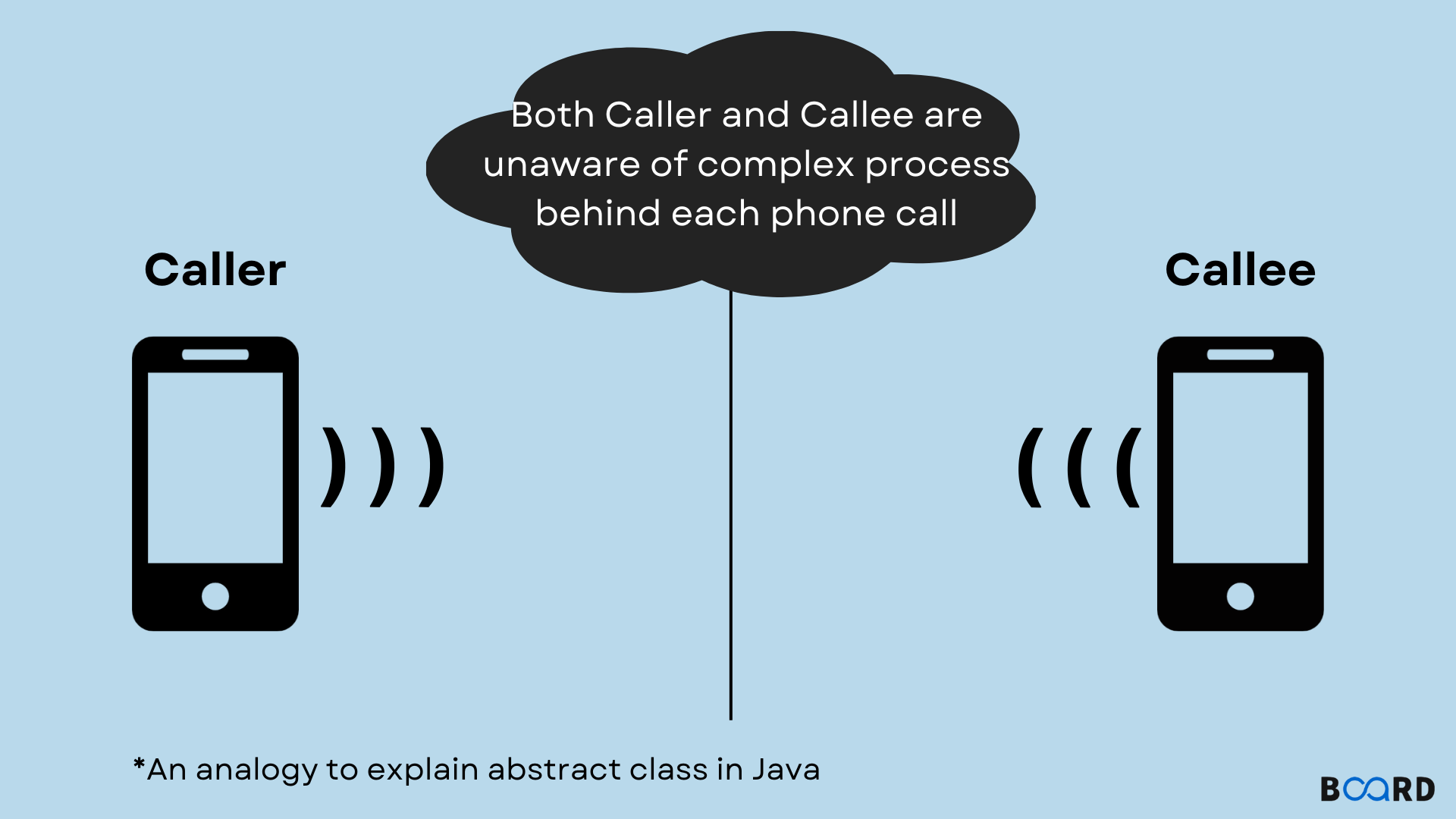
Introduction
When a class in java is declared with a keyword i.e. ‘abstract’, the class becomes an abstract class. It can contain non-abstract methods (methods with no body) and abstract. Before diving into the abstract classes let us have a look at the abstraction part of the java.
Abstraction
In Java, the process of only showing the functionality to the user and hiding the implementation part is called abstraction.
Another way to understand abstraction is taking the example of sending an email, you compose an email, attaching files etc then you send it. You are not aware of the processes occurring internally.
Mainly, abstraction lets the user only focus on the functionality of the object not how the object works.
What is Abstract class?
In java, the class which is declared with an abstract keyword is called the abstract keyword. It can contain non-abstract as well as abstract methods. Its methods need to be extended and then needs to be implemented. It can’t be instantiated.
Some important points about abstract class
An abstract class needs to be declared with an abstract keyword to be declared as an abstract class.
- It can contain non-abstract and abstract methods.
- It can’t be instantiated.
- It can also contain constructors and static methods.
- It can also contain final methods.
NOTE: Final Methods force the subclass so that it does not change the body of the method.
Syntax
Abstract Method in Java
When a method is declared with an abstract keyword and also does not contain implementation is an abstract method.
Syntax
Examples of abstract class in Java
The abstract class having abstract methods
Code
Output
Abstract class having constructor, data member and methods
Code
Output
Another example
Code