Java Advanced Topics and Techniques
String Array in Java
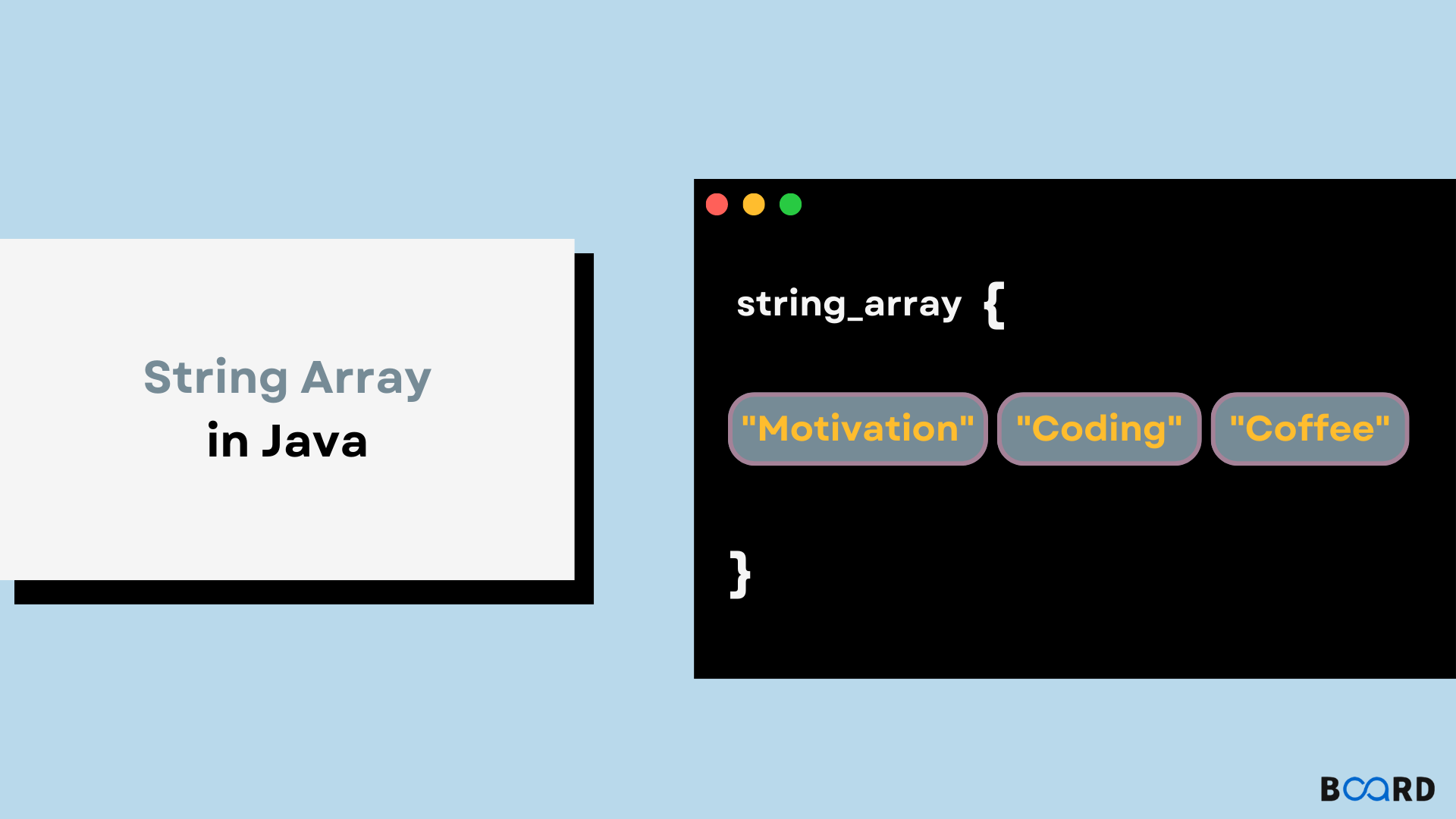
Introduction
An array is a collection of homogeneous forms of data that are stored in a series of memory locations and can individually be retrieved using their respective indexes in programming.
We have a String data type in the Java programming language. The string is only an object that represents a list of char values. In Java, strings are immutable. In Java, immutable refers to strings that cannot be changed.
It is known as a String Array in Java when a String array is created.
A String array must first be declared and initialized before it can be used. There are several options available for doing this.
Declaration
String arrays can be declared in programs either with or without size. Here is the equivalent code:
One String array (String0) without size and another (String1) with a size of four have been declared in the code above. Both of these approaches can be used to declare a String array in Java.
Initialization
In the first approach, the values are declared on the same line. The last method first creates the String array with the size before we store data in it, and the second method is a condensed version of the first method.
Iteration
A looping statement can be used to iterate through a String array.
Output:
Therefore, there are generally three ways to iterate across a string array. Utilizing a for-each loop is the first approach. The second approach uses a straightforward for loop, and the third approach makes use of a while loop. Iterating over Arrays in Java has more information about iterating over arrays.
Searching
A straightforward linear search technique can be used to locate an element in a string array. Here is how to put that into practice:
Output:
The String array in the code above has five elements: Apple, Banana, Orange, Grapes, and Pineapple. We are currently looking for a grapes. Our output is Grapes, which is present at index point 3.
Sorting
Sorting a String array involves placing the components in either ascending or downward lexical order.
To do this, we can either develop our own sorting algorithm from scratch or use the built-in sort() method; however, for the sake of simplicity in this post, we will utilize the built-in way.
Output:
Our String array, in this case, is not sorted, therefore after the sort operation the array is sorted in lexicographic order, which is the way we used to see it on dictionaries.
String array to String
The function toString() method can be used to convert a String array to a String.
Output:
Here, the String array is transformed into a string and saved in a string type variable, but one thing to observe is that the string also contains brackets and commas (,). Use the code line below to produce a string from a string array without them.
Output:
A StringBuilder class object is present in the code above. For each component of the string array, we are adding that (array). The function toString() method is then used to save the data contained in the StringBuilder object as a string.
Conclusion
A definite number of items of the string type make up a string array. Three methods exist for increasing the number of elements in a string array: pre-allocation, in which the array's size is increased so that values can be added later; construction; and use of the Java ArrayList class of the Collections Framework.