Java Advanced Topics and Techniques
Try, Catch and Finally in Java
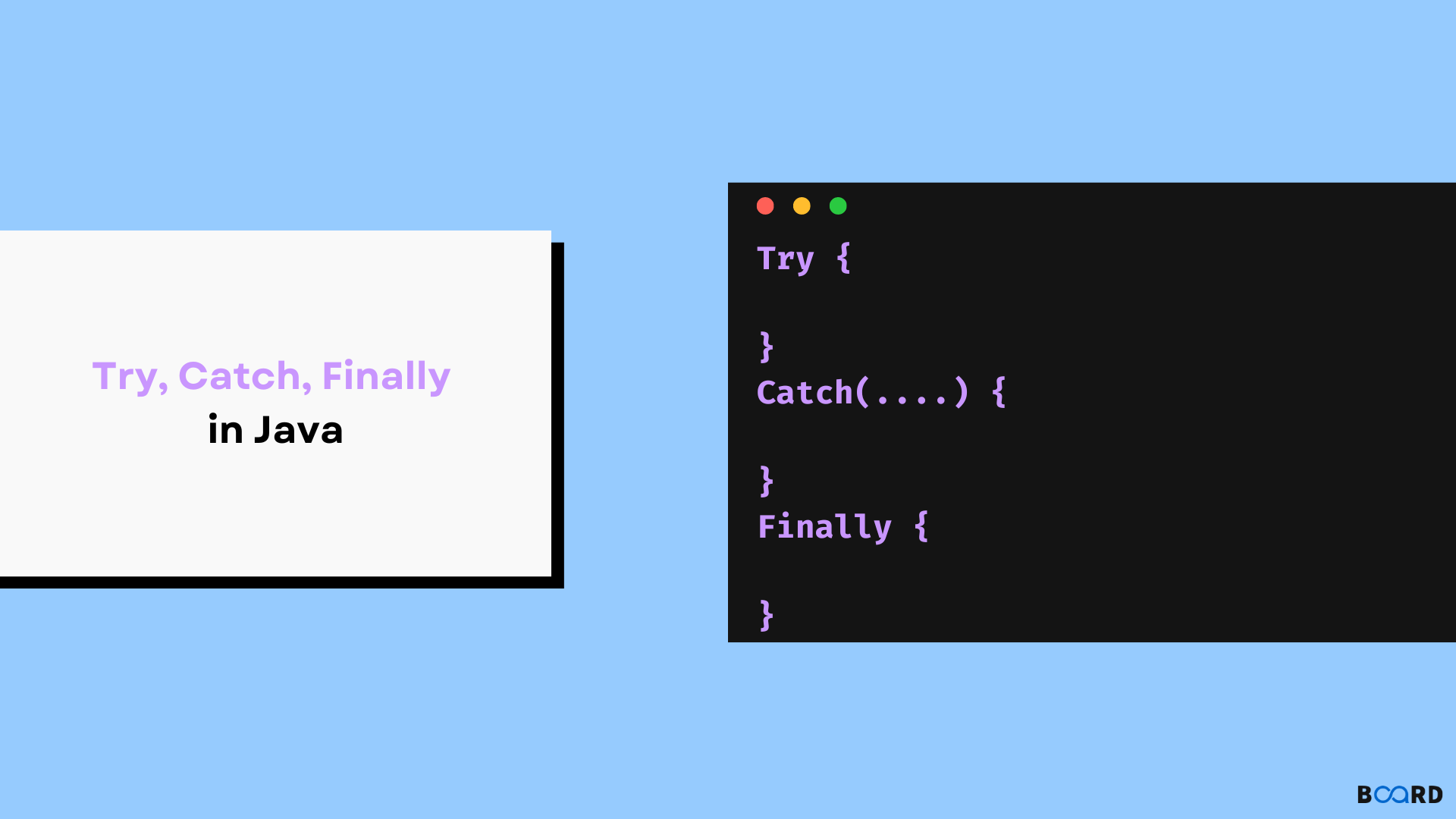
Introduction
While executing a Code of any programming language, there can be factors that obstruct the code execution. These are called exceptions. Thus, an exception is an anomaly or run-time error that hinders the normal flow of the program. In this article, we will see how we can handle the exception and maintain the flow control of java programs using the concept of Try Catch Finally in Java.
In normal conditions, the compiler executes the code in a top-down manner. But, whenever an exception is raised in any piece of code, the flow of the program is controlled by using the Try, Catch and Finally Block so that the program can be executed successfully. Exception Handling is one of the most important features of Java Programming which allows the developers to prevent the abrupt or abnormal termination of the program due to exceptions.
For example, suppose you take a number and try to divide it by zero.
You will encounter the following Arithmetic Exception:
Thus, the Try Catch Finally in Java is used to handle the piece of code which may cause an exception. For example in the above case, the try-catch in java can be used as:
Output:
Let’s see the various use cases of flow control using the Try, Catch and Finally block in Java code.
The Try Catch and Finally can be used in the following ways:
- Try-Catch Block: A try block is used with one or multiple catch blocks.
- Try-Catch Finally Block: A try block is used with the Catch and Finally blocks.
- Try-Finally Block: A Try block is used with the Finally block.
Following are the important points about the try-catch-finally blocks:
- There can be only one Finally Block.
- Try block cannot be used alone. It must have either a Catch block or a Finally block.
- You cannot have multiple try blocks with a single catch block in Java.
- One try block can be followed by multiple catch blocks.
Now, let’s see the various cases of control flow using the Try Catch Finally in Java.
Control Flow using Try-Catch or Try-Catch-Finally Block
Case 1
Exception is raised inside the try block and handled in the catch block.
In this case, if the exception occurs in the try block, the control immediately passes to the catch block without the execution of the remaining try block. If there is a finally block, then control passes to the finally block after the catch block. The implementation of this case of try catch finally in java is given below:
Output:
Case 2
Exception is raised inside the try block and not handled in the catch block.
In this case, if the exception occurred in the try block is not handled in the catch block, then the default handling mechanism handles it and the rest of the program executes as it is. This is shown by the following code:
Output:
Case 3
Exception is not raised in the try block.
Here, the catch block will never be executed as it can be executed only if an exception occurs. The try catch finally in java without any exception is demonstrated below:
Output:
Control Flow using Try-Finally Block
Here, the only possibility is that the exception is raised in Try Block and it may not be raised. If there is no exception in Try Block, then finally block will be executed after the try block exits. Then, the rest of the program will be executed. This is demonstrated in the code given below:
Output:
On other hand, if an exception occurs in the try block, then the finally block executes after which the default handling mechanism executes. Its implementation is given below:
Output:
This is how you can handle the exception and maintain the control flow using the concept of try catch finally in Java.