Java Advanced Topics and Techniques
Equals and == in Java: Differences
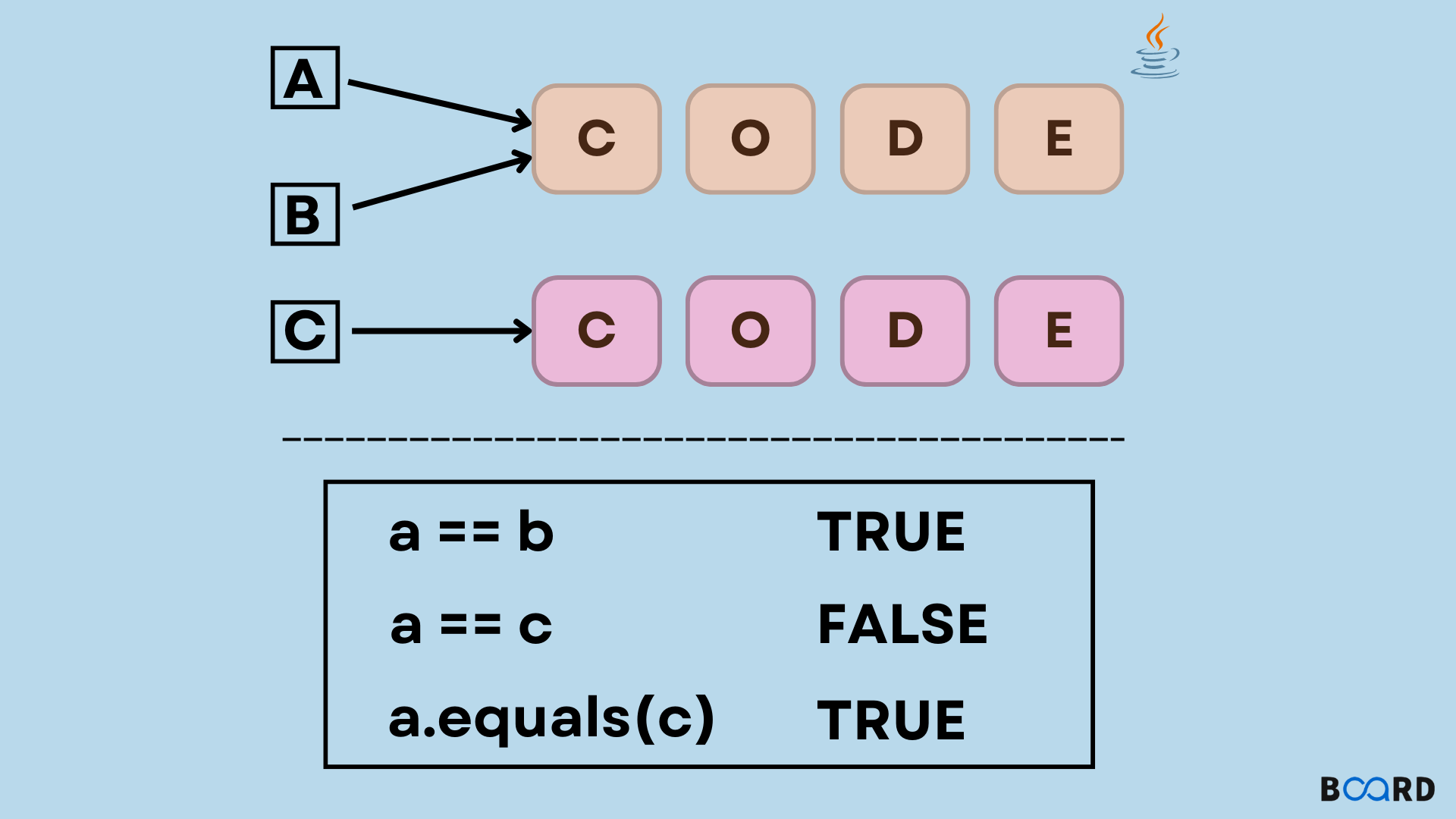
Introduction
You will learn about Java String comparisons in this post, including what they are, how to use them, and what it implies for you. Additionally, with an emphasis on equality comparisons, you will learn about the various comparison kinds. You'll see some code samples of the syntax and application of comparisons for a variety of tasks before the end.
String Equals() method
There are many ways to compare things in Java, but when doing so, you always need to take the value and data type into account. This can help you plan your software better and make the pseudocode much cleaner, which supports better coding techniques.
The equals() function and "==" operator are two of the numerous ways to compare strings to one another. These methods are intended to compare two strings to one another, which is their common objective. Each method, nevertheless, contrasts certain features of the strings. There are several aspects of strings to consider due to the nature of Java Strings. To compare a string's value or type, for instance, Java treats strings as objects rather than primitives. You may even determine if the string instance is the same as another one. Let's examine a few instances of string value comparison to see how it functions.
Code Implementation
Let's have a look at some sample code that demonstrates string value comparison using the equals() function.
Explanation
The equals() technique is used in this code block to compare various string variables. Due to similar values, the first comparison yields a true result, but the second contrasts different values and yields a false result. The third comparison, on the other hand, returns true since the two variables' values are identical. It's important to realize that the equals() function simply examines the string's actual value, oblivious to its other properties. The memory address and string type are two examples of elements that aren't taken into consideration. Let's now have a look at a double equal operator example of a string comparison.
Java String Equals vs. Equals-Equals
Because it additionally examines the string's memory address, the "==" is a little different. The condition returns true if the result and memory location for the compared lines are the same; else, it returns false. Other notable differences are covered in the image below.
No string produced using the new keyword will, in this regard, return true when compared to another string since it will already have a memory location.
Conclusion
You are now prepared to begin doing string evaluations in your programme code because this post covers a lot of ground. There are still other ways to exercise, hone, and expand your comprehension of the string comparison procedure. One last thing to keep in mind is that the methods covered in this piece may be applied to various kinds of data. Primitives, objects, and a lot more are included. You can keep drawing parallels of various types to enhance your comprehension of these strategies.