Java Advanced Topics and Techniques
Constructor Chaining in Java
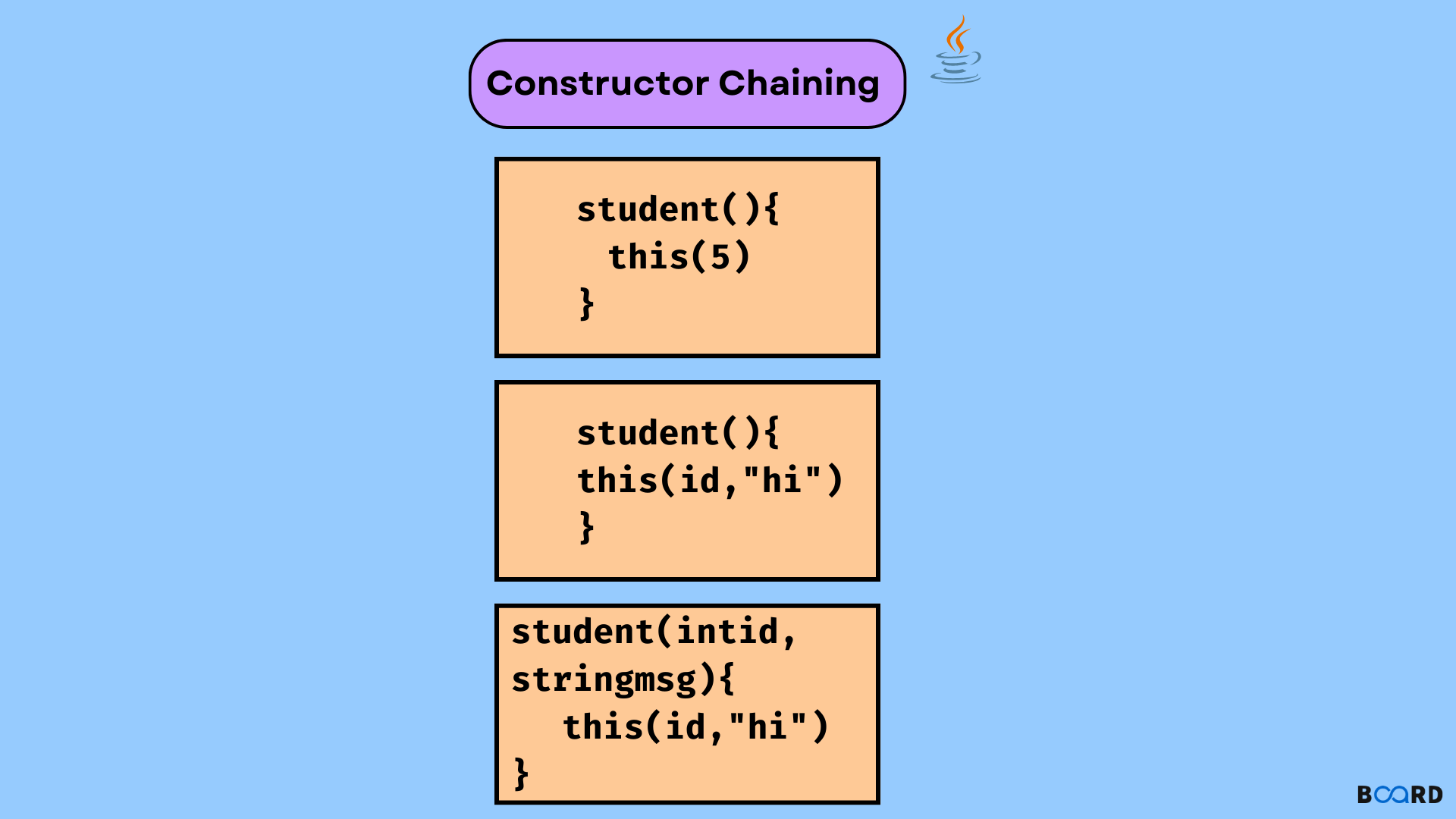
Introduction
In Java, constructor chaining involves invoking constructors repeatedly after creating an object. We can use this method when we want to invoke multiple constructors at once using only one instance.
This section will discuss Java constructor chaining in depth with appropriate examples.
Constructor Chaining
Constructor chaining is the method of invoking one constructor from another constructor in the same class. The process occurs through inheritance. Whenever we create an instance of a derived class, it invokes all constructors of the base class (inherited class), and then it invokes the constructor of the calling class (derived class).
There are two ways for constructor chaining :
Within the same class: When constructors are from the same class, we use this() method.
Syntax:
From the base class: When the constructors are from different classes (parent and child), we can use the super() keyword to invoke the base class constructor.
Syntax:
Need Constructor chaining in Java
Constructor chaining is a Java programming technique for performing multiple tasks and ordering them by having a separate constructor for each task. A developer can make the program easier to read and understand using this technique.
For example, If we do not use constructor chaining and they require a particular parameter, then we have to initialize it twice in each constructor.
Whenever developers change a parameter, they must make changes inside each constructor.
Note: In Java, one cannot call the constructor directly by name. So if we want to call a constructor, we need to use one of these two keywords mentioned above.