Java Advanced Topics and Techniques
Collectors toMap() in Java
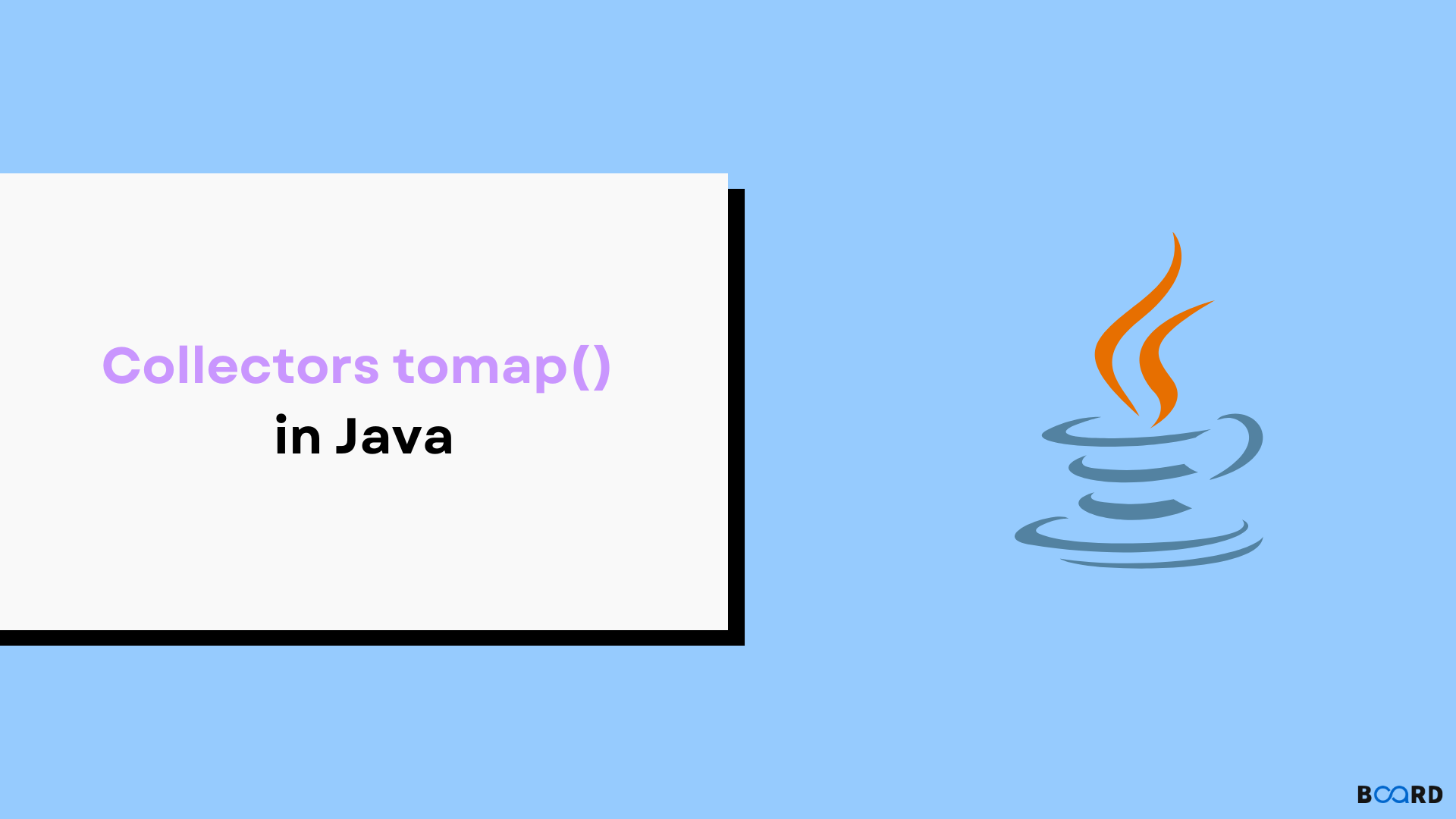
Introduction
Introduced in Java 8, collectors are final classes in Java that extend object classes. The class offers methods for reducing elements in collections based on various rules and criteria. Java collectors provide a static toMap method for producing new Map instances through the collector.
The keys in that newly created Map help calculate the values by applying provided mapping functions. Any repeating key would cause an IllegalStateException every time we try to execute a collection operation. Java Collectors toMap helps convert data structures (a list or an array) into maps by traversing all the elements of a list or array into a newly created Map.
Syntax
Here,
keyMapper: A key-producing or key-returning mapping function.
valueMapper: A value-producing mapping function.
Example
Collectors.toMap() with Mapper Functions
There are only two mapper functions required - a keyMapper and a valueMapper:
The keyMapper function outputs the key of the final Map.
ValueMapper returns the values of the final Map as its output.
The method returns a Collector, which collects elements into a Map, whose pair <K, V> represents the mapping functions previously applied.
Collectors.toMap() with Mapper and Merge Functions
We can also provide a Merge Function in addition to the two Mapper Functions:
Whenever there is a duplicate key element in the final Map, the mergeFunction gets called to merge their values and assign them to one unique key.
It takes two input values, i.e., the two keyMapper returned values, and combines them into one value.
Collectors.toMap() with Mapper, Merge, and Supplier Functions.
Developers can specify a Supplier function in the final overloaded version of the method - to implement a new Map interface for "packing in the result":
It specifies which implementation of Map we want to use for our final Map in the mapSupplier function. As a default, Java uses a HashMap to store the maps when we use Map to declare them.
Code Sample
The following code will help you understand how to use Collector Tomap() method in java.