Java Advanced Topics and Techniques
Java Custom Exception
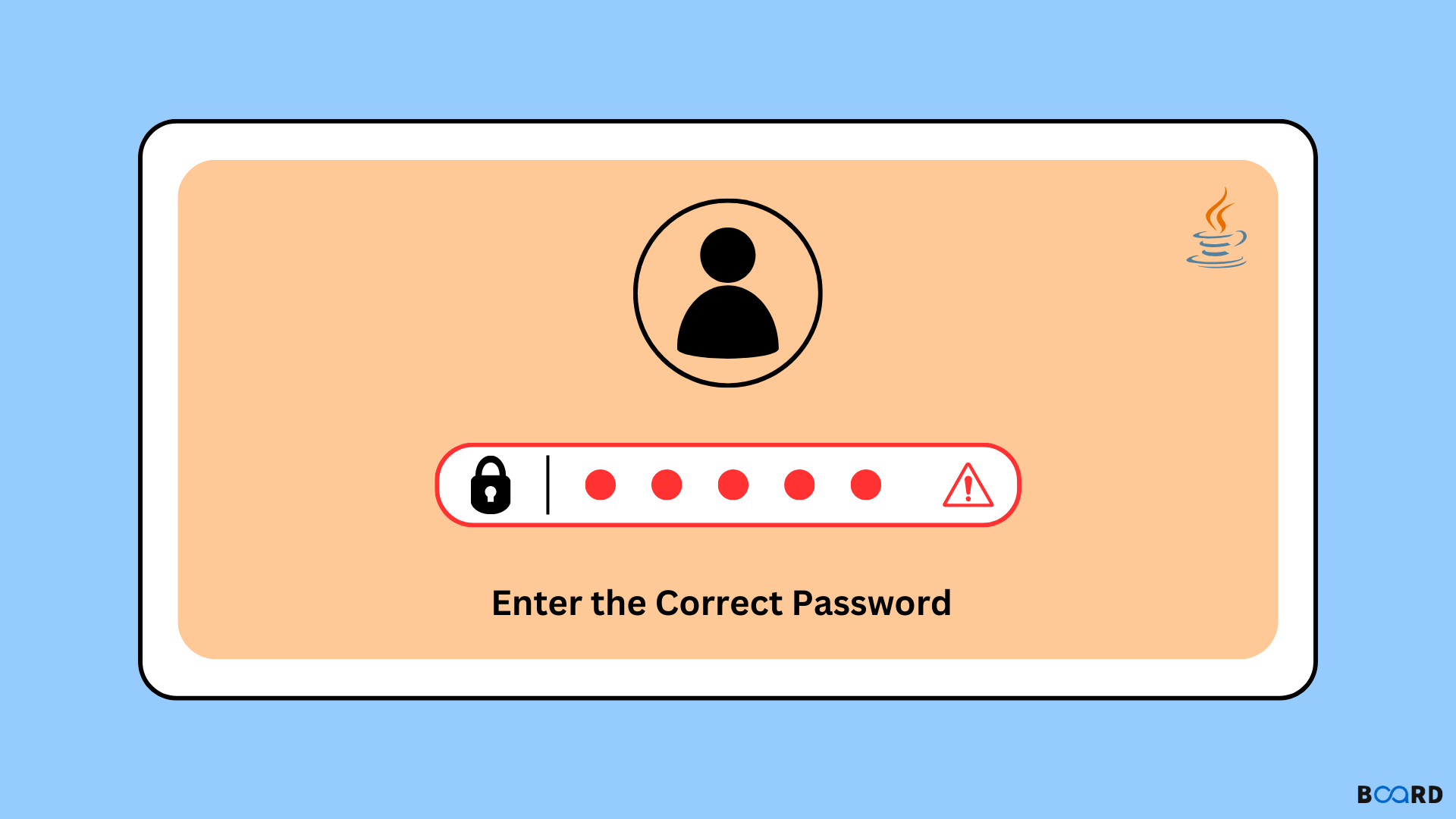
Introduction
In Java, custom exceptions can be created by deriving them from the Exception class. When a user creates a custom exception it is known as a user-defined exception. Generally, in Java User-defined customs are created to adjust exceptions according to a user’s needs. With the custom exception, we can make our exception and message. In this article, we will see how to create custom exceptions and how are they implemented.
Features of custom exceptions
All general types of exceptions that occur in programming almost get covered by Java exceptions. But sometimes there is a need to create custom exceptions. Some reasons to create custom exceptions are mentioned below.
- To a subset of some existing Java exceptions, to catch them and give them special treatment.
- Business logic exceptions: The exceptions that are related to business logic and their workflow. It can come in handy for developers and application users to know the exact problem.
Creating Custom Exceptions
Now to create a custom exception, the first thing we need to do is extend the Exception class which belongs to the “java.lang” package.
Let us have a look at the example below, in which we created a user-defined exception named “InvalidName”.
We have to create a constructor which takes the string i.e. the error message as a parameter and this constructor is called the parent class constructor.
Example 1
Let us try to understand a simple example of a Java User-defined exception. In the below code, the constructor of checkVote takes a string as the argument. This string then is passed to the constructor of the parent class Exception via the super() method. Calling a super() method is not necessary and also the constructor does not need to be always called with the parameter it can be called without parameters.
Code
Output
Example 2
In the code below there is no code written in the constructor of the parent exception class, also nothing is passed as a parameter, which is why it is printing null after catching the exception.
Code
Output