Java Advanced Topics and Techniques
ListSize() in Java
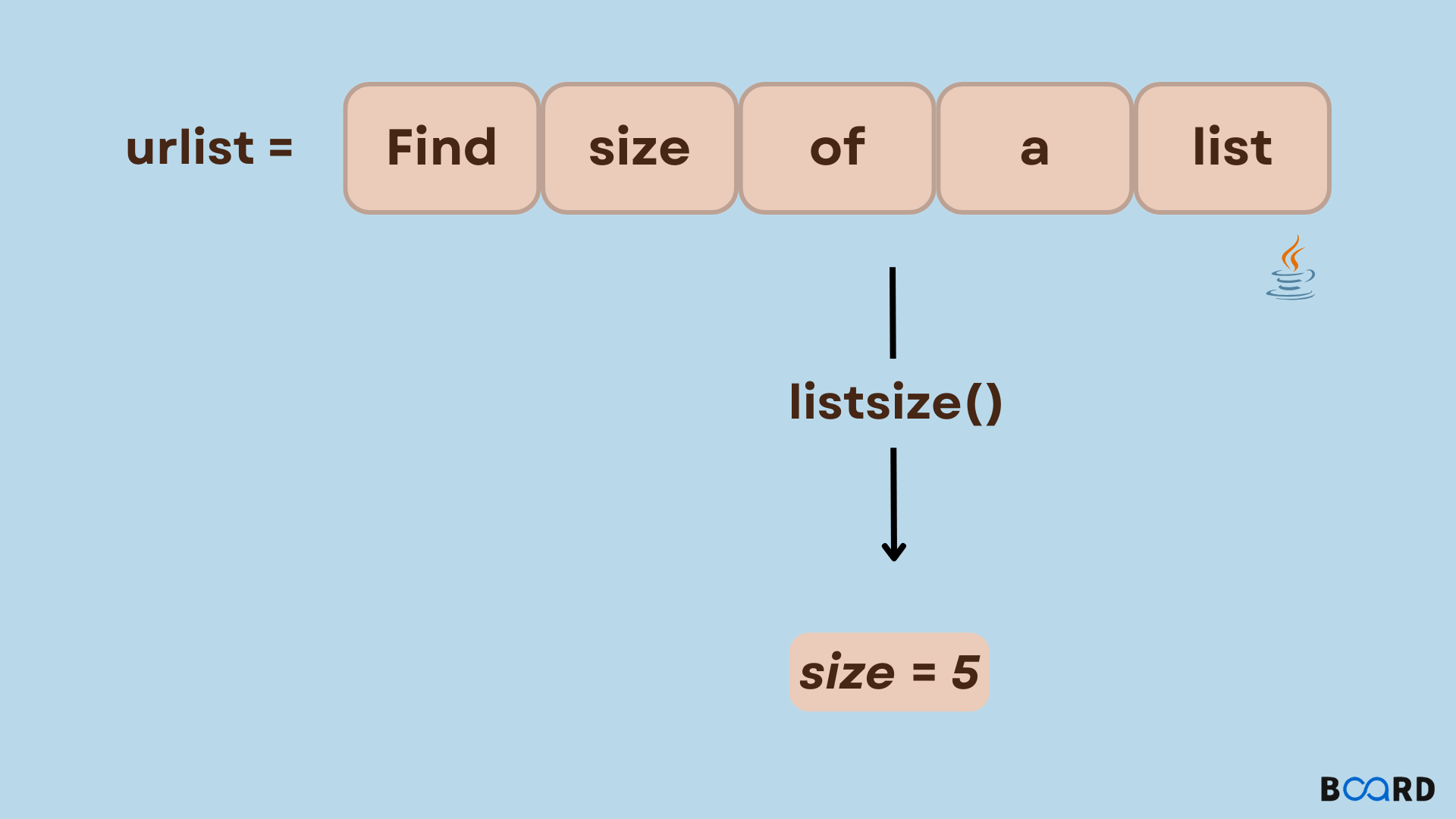
Introduction
In Java, the ArrayList class has a predefined method called size(). It is used to figure out how many items are in an array list. When the ArrayList uses this function, it does not require any input parameters and always returns an integer that represents the number of elements in the ArrayList. This method has an O(n) time complexity, where n is the total number of elements in the ArrayList object.
Syntax:
The declaration syntax for the java.util.ArrayList.size() function is shown in the sample below.
Parameters:
There is no input argument required for Java's List size() function.
Return values:
The ArrayList's element count is returned via the Java List size() function. For instance, the size method will return five if an ArrayList contains five string objects stored in it.
Exception for Java ListSize()
When the application tries to determine the size of a null ArrayList, NullPointerException is produced. To avoid it, you can decide whether to verify if the list is empty or not.
Example: Java list size():
Assume you wish to use the size() method to determine the size of an ArrayList that contains some names. The Java code that follows shows how to build an ArrayList of Strings, add two string objects to it using the add() function, then get the number of entries in the list using the size() method. We added a few additional details, adjusted the size, and decorated it.
Output:
Java's internal implementation of ArrayList.size()
When we call the size() function, it simply returns the value stored in the size variable, which is a constant-time operation. This is how Java implements ArrayList such that it always maintains track of the current size of the list in a separate variable named size.
Let's now examine how the size() method operates in the context of the previous example.
- MyList starts out empty, and the size variable holds 0 in it. After we added two values, the size variable now holds 2. The list's size() method then yields the same result (2).
- Once more, we added two values, and the size was changed to 4 when we called the myList's size() method.
- The size() method will be a constant-time operation because all it does is return the value contained in the size variable.
More Example
The same size() method can be used in Java to determine how many elements are kept in a HashSet. The implementation for the same is demonstrated in the example below.
Output:
Conclusion
- Java's List size() function can be used to determine a list's size; it returns an integer that indicates the list's size without asking for any additional parameters.
- The return value of the size() method can change because ArrayList has a dynamic size, as demonstrated in the example.
- Data with dynamic sizes, such as student records or information about the COVID-19 vaccine, can be stored in ArrayList.