Mastering Fundamentals: C++ and Data Structures
A Detailed Overview of Macros in C++
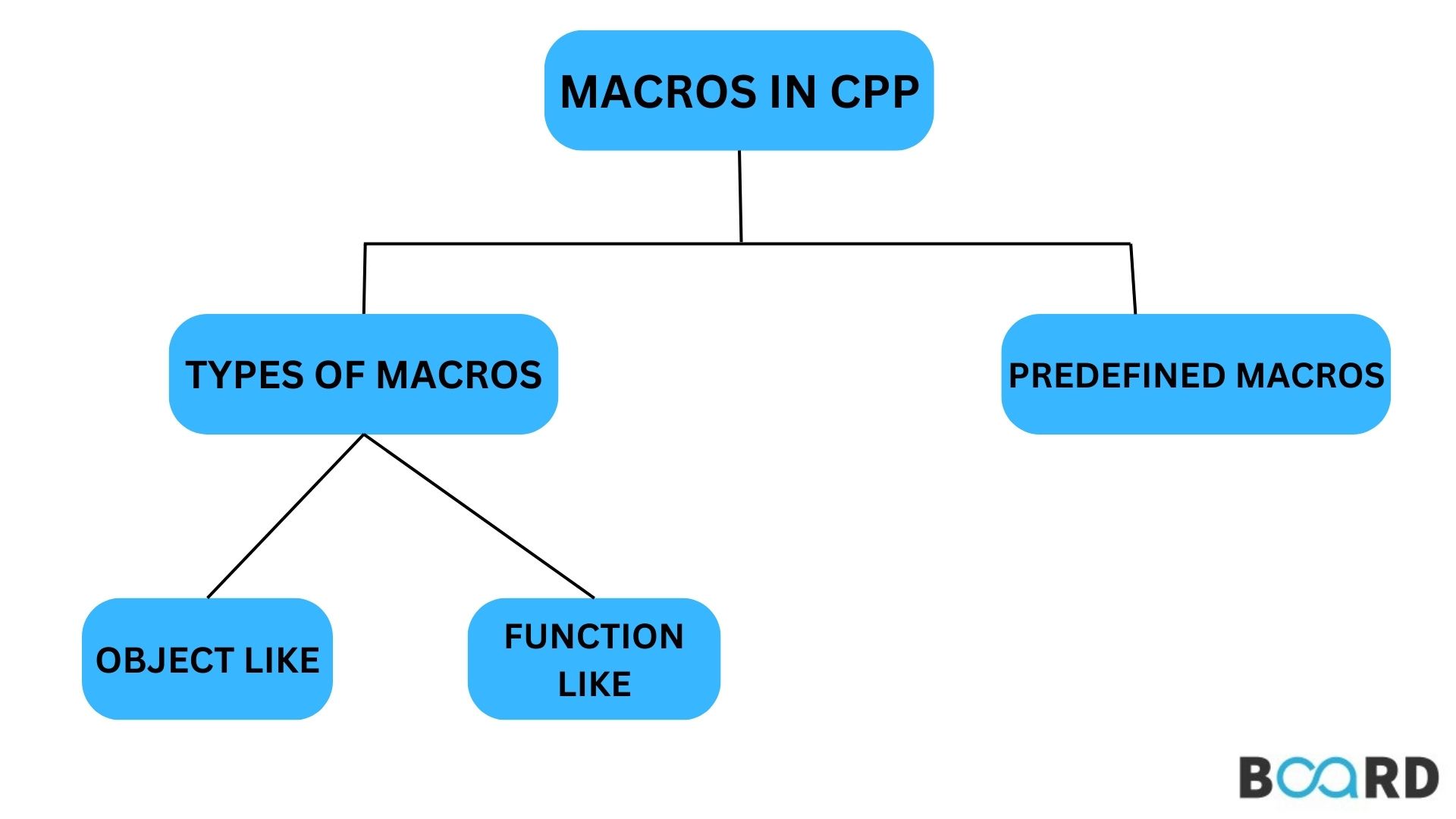
Introduction
A macro is described as the section of code that the macro's value replaces in a program. Using the #define directive, we can define the macro. A compiler substitutes the macro name with the macro definition whenever it comes across it. There is no requirement to use a semicolon to end the macro definition (;). Let's talk about the simple illustration to comprehend this idea:
Example
Explanation
We specify the macro's value. We create the driver software for the main. This displays the defined macro's value.
Types of macros
Four different types of macros exist:
- Chain macros
- Object-like macros
- Function-like macros
- Multi-line macros
Chain macros
The definition of a chain macro is a macro inside a macro. First, the child macro is expanded, and then the parent macro is expanded.
Example
Explanation
The parent and child macros are defined. The vehicle is enlarged in this case to create CARS. The macro is then enlarged to create the result.
Object-like macros
A basic identifier that is substituted by a code snippet is what is referred to as an object-like macro. In programming, it resembles an object. As a result, it is referred to as an object-like macro.
Example
Explanation
We specify the macro's value. The driver code for the main is being written. This displays the macro's specified value.
Function-like macros
Function calls and function-like macros have the same functionality. Instead of just changing the function name, the complete code is changed.
Example
Explanation
The function-like macros are defined. The primary driver of the software is being written. This executes the function and outputs the defined macro's value.
Multi-line macros
Multiple lines can make up an object-line macro. Consequently, we must use backslash-newline to generate multi-line macros.
Example
Explanation
The multi-line macros are defined. Multi-line macros are called inside of an array that we create. Then, we print the array's elements.
Predefined Macros in C++
There are several predefined macros in C++, including the following:
- __DATE__: Current date in the following format - MMM DD YYYY.
- __TIME__: The time is shown as HH:MM:SS.
- __FILE__ : Current filename
- __LINE__: Deconotes current line number
Conclusion
In this article you understood macros, its 4 types and some predefined macros provided by the C++ libraries along with examples and code snippets.