Mastering Fundamentals: C++ and Data Structures
Working of Jump Statements in C++
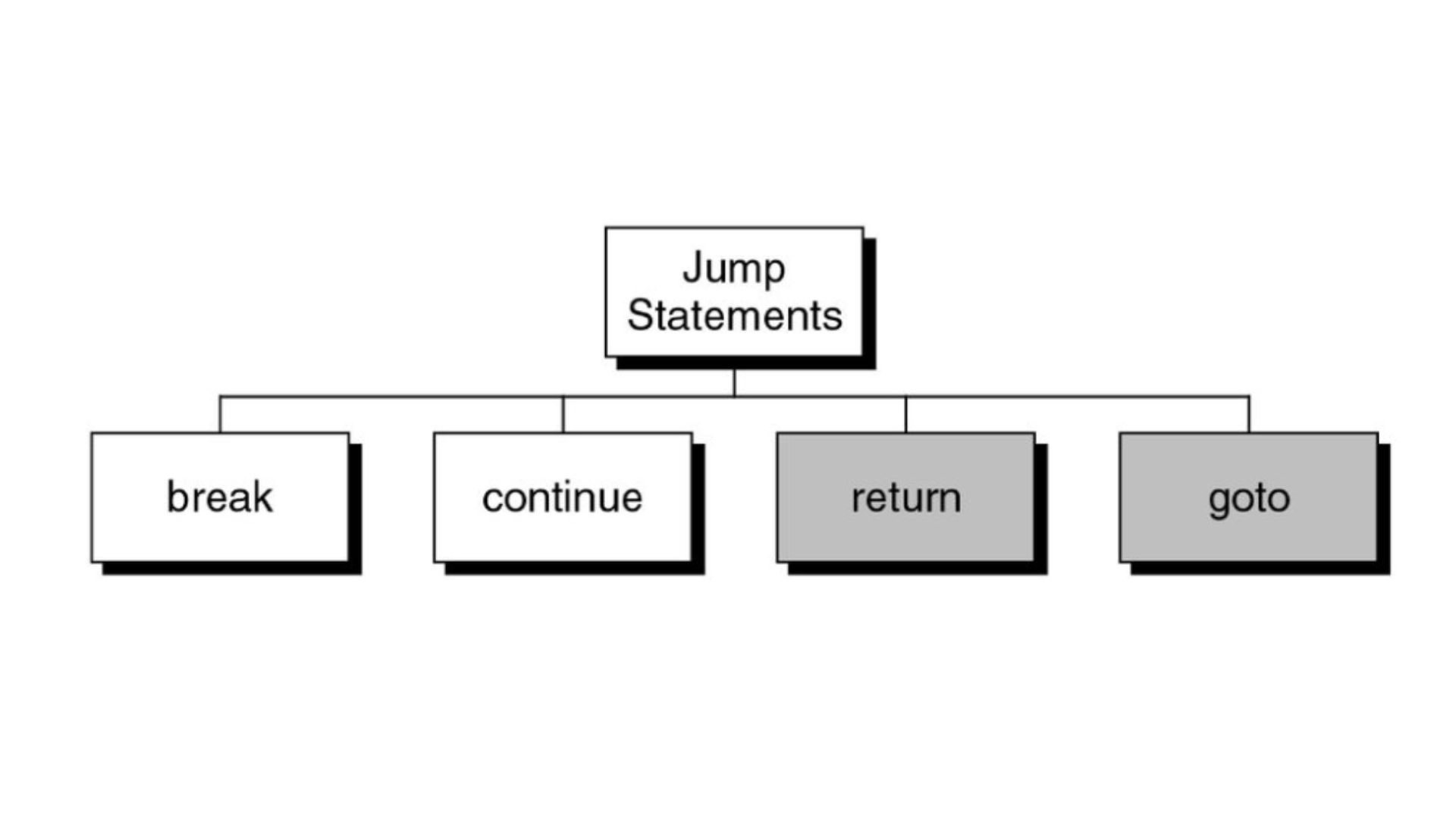
Introduction
To stop a program from running normally, use a jump statement. 4 Types of Jump statements are:
- goto
- break
- continue
- return
Let's examine each of them individually.
goto statement
A control statement called a "goto" is used to move the focus of a program's attention from one location to another without imposing any conditions. The goto statement's syntax is as follows:
goto label;
..
label: statement;
An identifier is a label in the previous syntax. The program's control goes to label: and runs the code there when the goto label; is encountered
C++ script that uses the goto instruction to compute the average of two values.
The average of the user-inputted integers is determined in the above code snippet. The average of the entered numbers is disregarded if the user submits a negative value. up until a calculation of that moment.
Break statement
A break statement, also known as a jump statement, stops the execution of a loop and transfers control so that the loop's body may continue normally.
N number count in C++ using break statement
In the aforementioned example, the loop will cycle 20 times under the while condition, but the break statement will force the loop to end once the count hits 5.
Continue statement
Similar to how the break statement functions, the continue statement also does. The continue statement compels the loop to continue or carry out the subsequent iteration as opposed to ending it (the break statement does that). The code inside the loop that comes after the continue statement is skipped whenever the continue statement is used in a loop, and the subsequent iteration of the loop then starts.
C++ script using continue statement to display numbers upto 10 except 6
The loop iterates 10 times in the example above, but due to the continue expression, if i reaches 6, control is passed to the for loop.
To check out similar topics and questions, do visit our Discussion Forum.