Mastering Fundamentals: C++ and Data Structures
A Quick Guide to Circular Linked List in C++
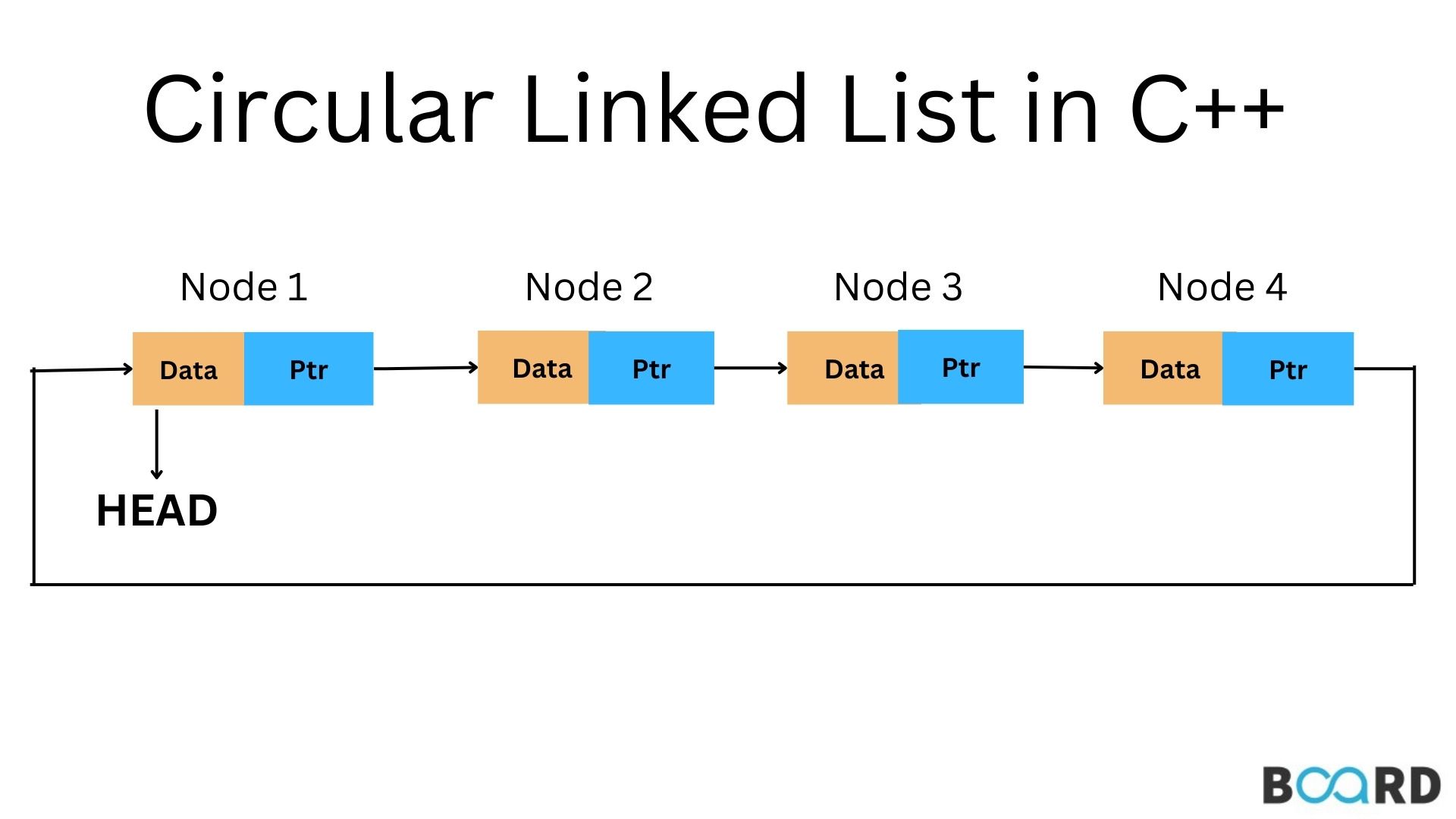
What is a circular linked list in C++?
Another data structure that improves on the linked list data structure is the circular linked list in C++. As we have learned, a linked list is a type of data structure in which each node has a link to the node after it, with the last node referring to null. Similar to a linked list, a circular linked list includes links connecting each node to the one after it. Unlike a linked list, the final node in a circular linked list connects to the list's head rather than null.
Why Circular Linked List?
Moving through the list is simple.
Since the list has a loop-like structure, the entire list may be explored from any node. Because of this, moving from the final node to the first node just requires one step.
Application of a cutting-edge data structure
Modern data structures like queues and others are implemented using circular linked lists.
Useful in applications that loop back on themselves
Circular linked lists make it simple to create applications that loop endlessly or never stop.
Structure of the circular linked list
There are two types of circular links: single and double. The following collection of code may be used to define them in a program.
The individually circular linked list includes:-
This is a doubly circular linked list:-
Operation on the circular linked list in C++
- Inserting at the list's beginning.
- At the end of the list, insert.
- Inserting at a Particular Place in the List
- Removing from the list's beginning.
- Removing from the list's end.
- Removal of a Particular Node from the List
- Dividing a list into two equal parts
- List insertion with sorting
Code Example
Applications of Circular Linked List in C++
The circular linked list has numerous practical uses, and it is used to build a variety of intricate data structures. Here are a few of them:
- The operating system uses circular linked lists to manage computer resources. Additionally, linked lists are frequently used in scheduling circulars.
- Token scheduling in computer networks uses circular linked lists.
- Circular linked lists are used to build a variety of sophisticated data structures, including the Fibonacci Heap.
- It may also be used to implement the data structures queue and stack.