Mastering Fundamentals: C++ and Data Structures
Inline Functions in C++
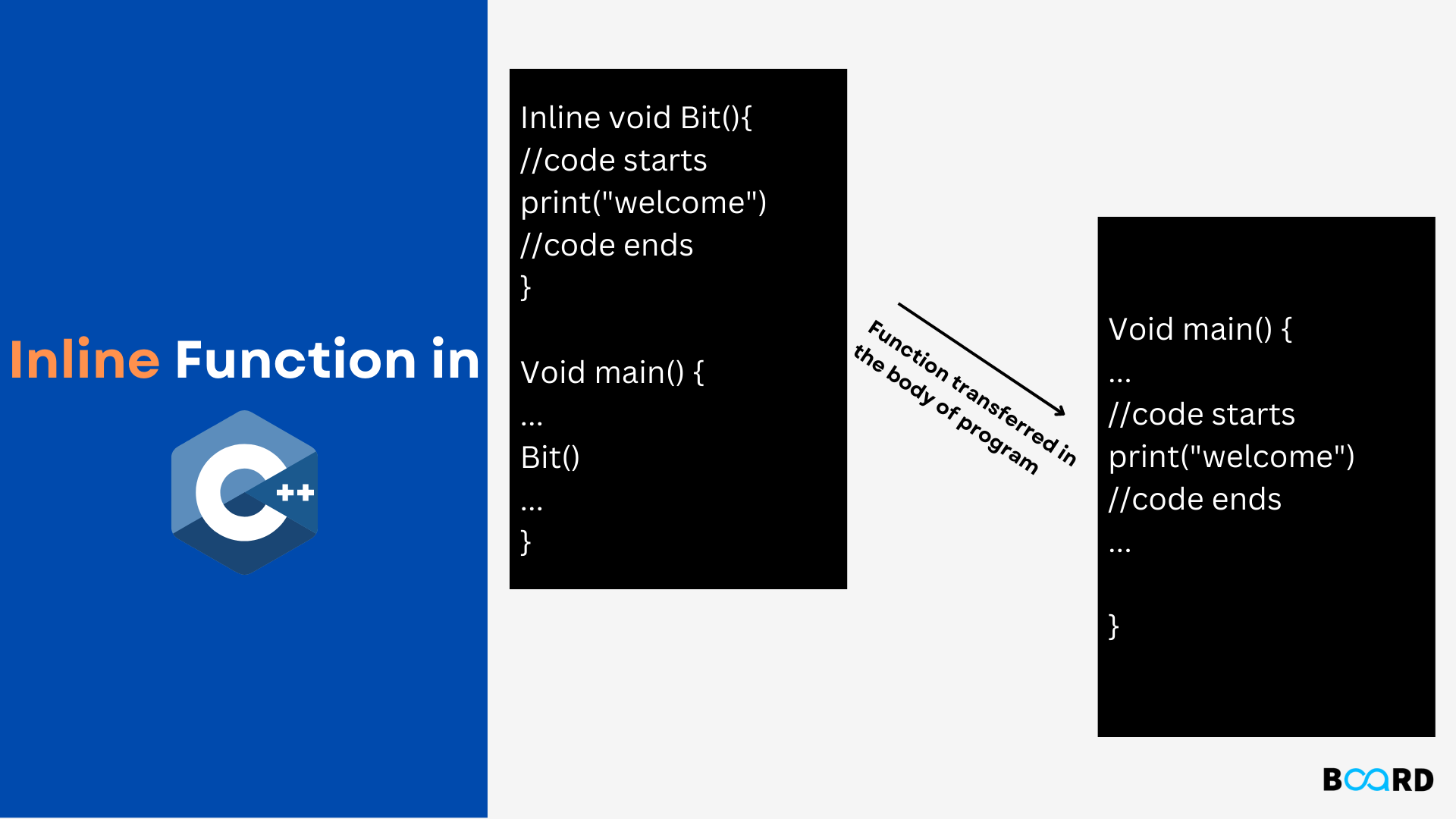
Introduction
In this article, we will discuss inline functions in C++. A function can be declared as inline in C++ which may speed up program execution by compile-time copying the function to the location of the function call. The inline functions are one of many fascinating C++ concepts that can be very helpful for a program's performance. It is crucial to understand how and what inline functions do before adding one to a function. Without further ado, let's get right to the point of our discussion!
Why Are They Required?
Similar to how macros in C reduce program execution time, inline functions are an optimization feature in C++. When handling classes in C++, this idea of inline functions is used. But it is also applied in other contexts. In other words, such a function could be described as being expanded in-line, hence the name inline function.
When a program calls a function multiple times and the definitions are brief, inline functions are commonly used. It takes less time to move program control to the function definition when inline functions are used. But rather than being a necessary command, inlining a function is merely a request made to the compiler. The compiler will decide whether to accept or reject this suggestion to inline a specific function.
Under some circumstances this request is rejected by the compiler:
- The compiler does not comply with the programmer's request when the function is not returning any value.
- For a function that has a loop (for, while, or do-while).
- Recursive functions are unable to be inlined.
- Functions involving static variables cannot be inlined
- If a function requests to be inlined but contains switch or go-to statements, the compiler rejects the request.
The following syntax has been provided to define an inline function:
Code
In C++, "inline" is a keyword that is used to make a function inline. Here is a sample of C++ code demonstrating how an inline function is implemented:
Code Explanation
- The main() function was written first in this case, and the inline function add(int a, int b) was then called within the main () function.
- The code from the add function definition is inserted there when the add function is invoked, or inlined at the time of compilation.
- It no longer incurs the cost of function calls and returns.
- Due to its numerous benefits, which are stated below, C++'s Inline function is a very valuable feature.
Advantages
Some notable benefits of inline functions are:
- Since there is no function call overhead, program speed is increased.
- It aids in reducing the overhead of a function's return call. helpful in reducing the overhead of variables being pushed and popped off the stack when invoking a function.
- The locality of reference is increased by using the instruction cache.
- For tiny embedded systems, inlining a function frequently results in less code than the preamble and return of the function call.
Summary
- Inline functions are not required; they are only recommendations. The compiler might not inline the routines that the programmer designated as inline.
- In addition, even if a function is not designated as inline, the compiler may nevertheless choose to inline it. Since they are already considered to be inline functions by default, functions that are declared and defined inside of a class do not need to be explicitly specified as such.
- Inline works as a compiler controlled copy and paste action and is not implemented forcefully, which makes it easier to debug than preprocessor macros and ensures that the namespaces and code are not corrupted.
- Virtual methods cannot be inline functions. A method or function is not immediately inlined only because it is used as a template in the header section.