Mastering Fundamentals: C++ and Data Structures
Understanding Substr() Function in C++
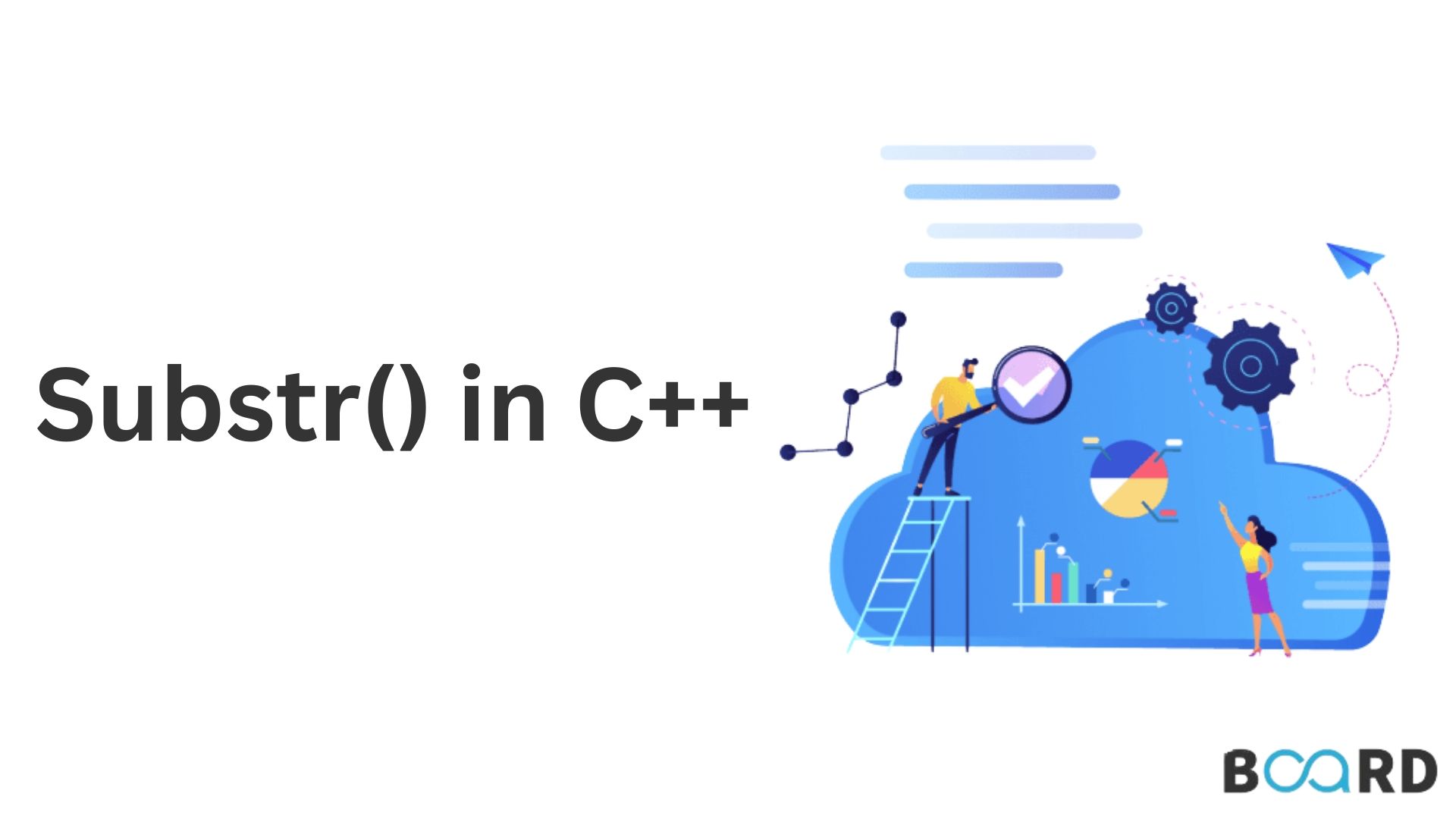
Introduction
You may use the substr() function to discover the substring inside a given string, or use a looping method to find the substring on your own. In this article, we'll go through a few methods for locating a string's substring given its length, beginning position, or ending position.
Syntax of the substr () function
A member method of the string class is called substr(). The syntax for the substr() function is as follows.
substr (pos, len)
- A string object calls the substr() method.
- Pos is the substring's beginning index or location within this string.
- Len is the substring's length in characters.
- Pos has a default value of zero.
- The entire text is returned as a substring if pos is not supplied. Len can be omitted. The substring up until the end of this text is returned if len is not given.
- A substring constructed using this string, pos, and len is represented by a freshly created string object that substr() returns.
Example #1:
Get a substring with a start point and length using substr(). In this example, we'll get a string with an initial value and look for the portion of it that begins at a specific position and ends at a particular length.
Code
Note: Len will be modified such that the substring terminates at the end of the main string even if you supply a length that exceeds the main string's maximum length.
Example #2:
Find Substring with just Start Position using substr() In this example, we'll take a text and search for the substring that begins at a specific beginning point. The length of the substring is not provided. The supplied start point through the string's conclusion should be covered by the returned substring.
Code
Example #3:
Finding a substring without any start point using substr() as an example In this illustration, we'll take a string with an initial value and identify its substring. The start location of the substring is not provided. The string that is given back must be a duplicate of the original string.
Code
Example #4:
Find Substring with End and Start Positions using substr() In this example, we'll take a string and search for the portion of it that starts at a specific starting position and ends at a specific finishing position. The length, not the end point, is the argument that the substr() method takes. As a result, we will modify the len input to create a length value by utilizing the start and end places of the original string's substring.
Code
Example #5:
Using a for loop to find a substring In this example, we'll use a C++ For Loop to locate the substring of a text given its start position and length.
Code
For the same purpose, you may discover the substring using a Do While or While loop in C++ as well.
Conclusion
This C++ tutorial showed us how to use example scripts to understand how to determine a string's substring in C++.