Mastering Fundamentals: C++ and Data Structures
Understanding Copy Constructor in C++
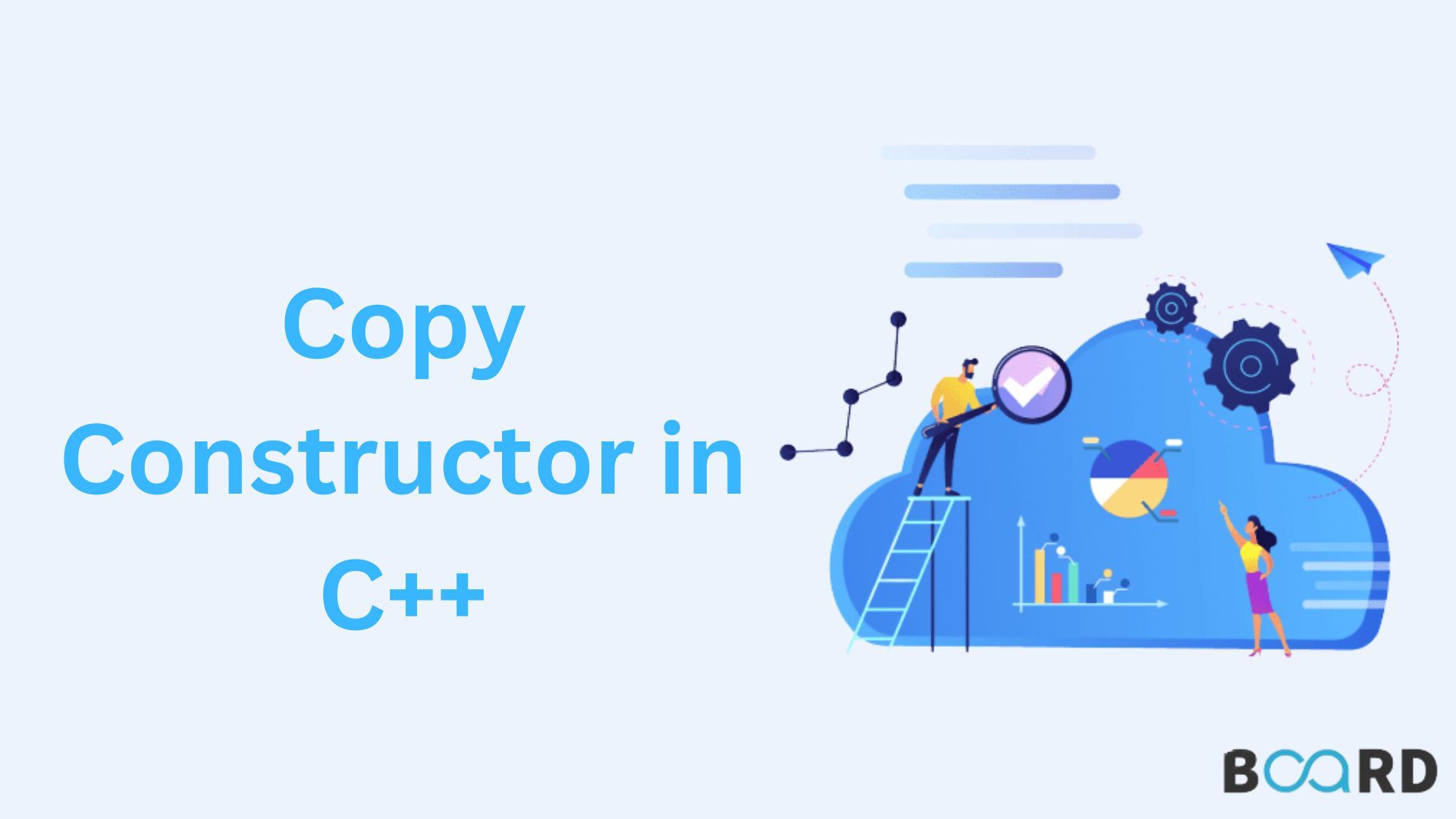
Introduction
Through the use of examples in this lesson, we will learn about the C++ copy constructor and its type. To make a duplicate of an existing object of a class type, a constructor type called a copy constructor is employed. Usually, it has the shape X (X&), where X stands for the class name. To all the classes, the compiler adds a default Copy Constructor.
Syntax of Copy Constructor
It is referred to as a constructor since it is used to generate objects. And because it makes an identical copy of the current copy when a new object is created, it is known as a copy function ..
Copy construction of objects (CODE)
Shallow Copy Constructor
Through the use of an example, the shallow copy constructor idea is demonstrated. From two distinct PCs connected by a network, two students are simultaneously inputting their information in an excel spreadsheet. The excel sheet will reflect any changes that they both make. when the exact same Excel document is open in both places. In the shallow copy constructor, this occurs. Each item will point to the same location in memory. reference to the original items is copied in shallow copies. There is a default copy constructor provided by the compiler. Shallow copies are offered by the default copy constructor, as seen in the example below. It is an object's bitwise copy.
When a class is not working with any dynamically allocated memory, a shallow copy constructor is utilized.
You can see that both objects (C1 and C2) in the example below link to the same memory address. When the c1.concatenate() method is used, c2 is likewise impacted. Therefore, the result from c1.display() and c2.display() will be the same.
Deep Copy Constructor
Let's use an illustration to demonstrate how a deep copy constructor works. Because you don't have enough time and have an assignment due tomorrow, you stole a copy from a buddy. You and your friend now have different copies of the same assignment. As a result, any changes you make to your copy of the assignment won't appear in your friend's copy. In the deep copy constructor , this occurs. Deep copies set aside distinct memory for duplicated data. The source and copy are therefore distinct. Any modifications performed in one area of memory have no impact on the duplicate there. We require a user-defined copy constructor when utilizing pointers to allocate dynamic memory. The two objects will each point to separate parts of memory.
Requirements for deep copy
- A standard constructor.
- A destructor that deletes the dynamically allocated memory.
- A copy constructor for making copies of dynamically allocated memory.
- Overloaded assignment operator.
Because both c1 and c2 are looking to the same memory address, you can see in the preceding example that when c1 is called concatenate(), changes happen in both c1 and c2. You can see a user-defined copy constructor, or deep copy constructor, in the example below. Here, c1 and c2 each point to an alternative memory address. Therefore, modifications done in one place won't have an impact on the other.