Mastering Fundamentals: C++ and Data Structures
How File Handling Works in C++
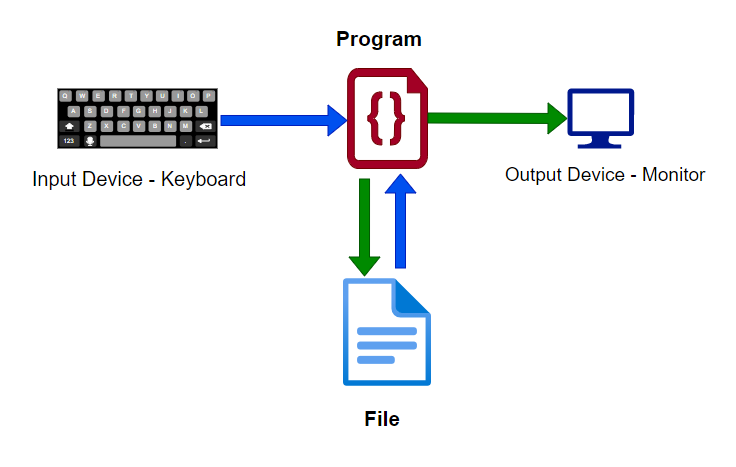
Introduction
C++ provides us with various inbuilt functions using which we can easily deal with files. File handling refers to storing some information permanently on the disk space (or Hard disk). File handling can be achieved in any programming language using the following steps:
- Name a file
- Open a file
- Write the required data into the file
- Read data from a file
- Close a file after the required operation
Streams in C++
Stream is defined as the sequence of bytes passed as input to a program that is being executed and then it returns again a sequence of bytes as an output. In simple words, we can say that a stream is a flow of information in the form of sequences.
The executing program interacts with the input/output devices and performs operations. Such operations are known as console I/O operations. Similarly, the executing program interacts with the files and performs input and output operations, and such operations are known as “disk I/O operations”.
Inbuilt classes for File stream operations
In C++, the input/output system provides us with a number of classes that contains file-handling methods. These classes are the listed below:
- ifstream
- ofstream
- Fstream
These classes are defined under the fstream header file. We use ifstream class to read data from files, ofstream class to write data into files, fstream class in order to perform both the operations, read from and write into files.
Now the primary steps before reading or writing into files is to open a file. A file can be opened using one of the following ways in C++:
- During object creating, give the file name to the constructor.
- Open file using open method.
All these methods have the following structure:
Constructor method to open a file:
Open method to open a file:
Parameters:
Here the nameOfFile denotes the name of the file to be opened and mode is one of the following:
The default file opening modes of ifstream, ofstream, and fstream are the following:
Now let us consider a program that illustrates the working of ifstream and ofstream class:
Source Code
Input:
Board Infinity
-1
Output:
“sample.txt”
Output Description:
As you can see in the output, We have input the string “Board Infinity” from the user and written the same in the “sample.txt” file.
Now let us consider a program that illustrates the working of fstream class:
Source Code
Input:
Board Infinity
-1
Output:
“sample.txt”
Output Description
As you can see in the output, We have input the string “Board Infinity” from the user and written the same in the “sample.txt” file.
Conclusion
In this article, we discussed how file handling can be done using objects in C++. We saw the working of the classes: fstream, ofstream, ifstream. We believe that this article has surely helped you to enhance your knowledge of file handling in C++.