Mastering Fundamentals: C++ and Data Structures
Becoming Friends with Friend Function in C++
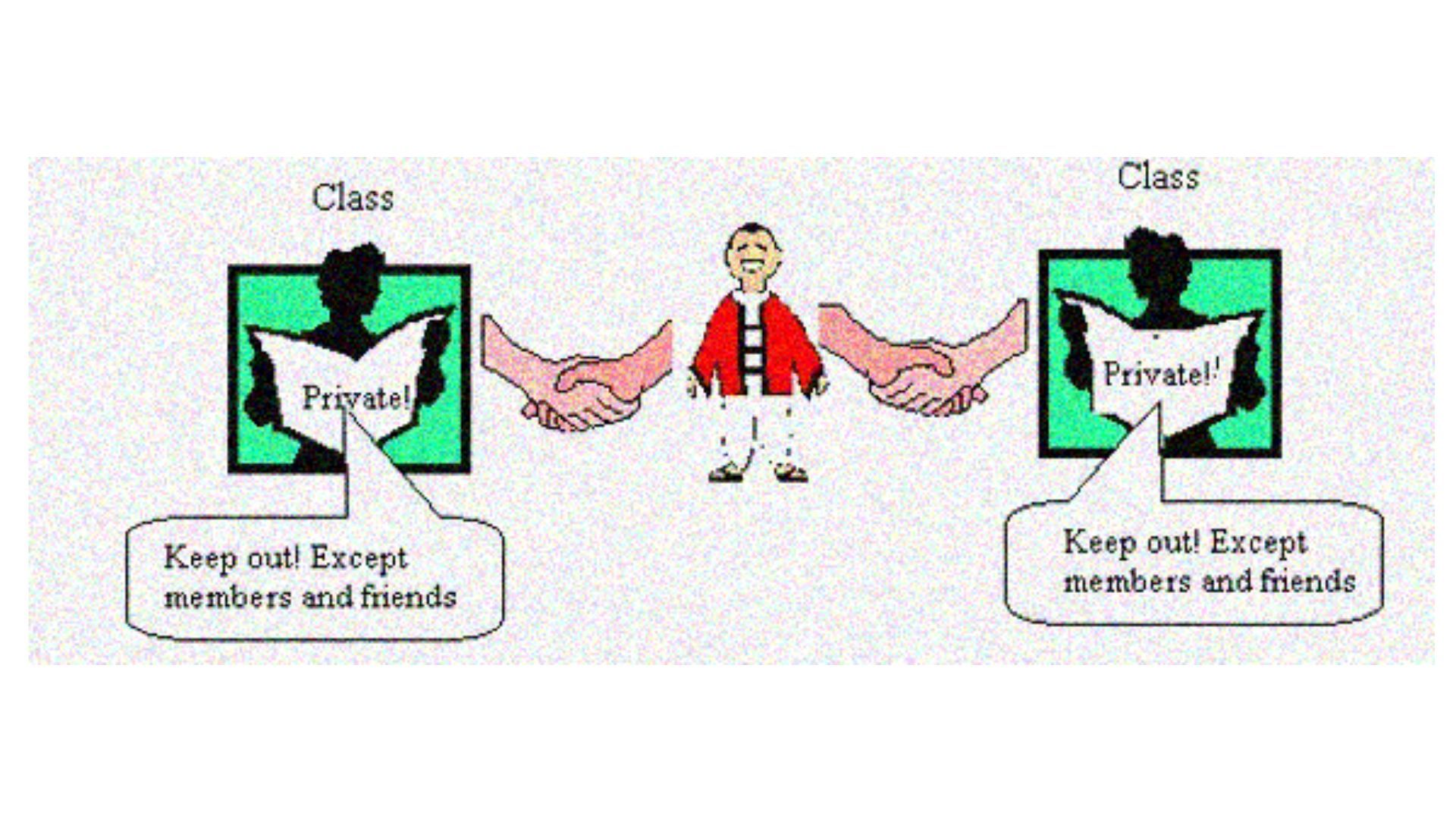
Introduction
With the aid of examples, we will learn how to create friend classes and functions in C++ in this article. An essential idea in object-oriented programming is data hiding. Private members from outside the class are not allowed access. Similar to protected members, which are unavailable to outsiders and only accessible via derived classes. In C++, the friend function is defined outside of the class's boundaries. It has the right to access both private and protected members of the class. Although friend functions are not member functions, only class functions include prototypes for friend functions. It addresses class templates, classes, functions, functions, and member functions if the class's whole membership is a friend.
Why do we need Friend Functions?
Let us take this example for instance,
Friend functions, a C++ feature, allow us to circumvent this restriction and reach data and functions from outside of the class.
A function can access the private as well as the private data of a class if it is written in C++ as a friend function. Compiler recognizes the supplied function as a friend function via the keyword friend. The declaration of such a friend function must be made inside the class body that begins with the term "friend" in order to access the data.
Characteristics of Friend Functions
- It must use an object identifier and dot membership operator in conjunction with the member name because it cannot retrieve the member names directly.
- The class that it has been designated as a friend does not cover it.
- It is beyond the scope of that class, thus it cannot be invoked using the object.
- Without utilizing the object, it may be called as you would any other function.
- Either the private or the public component may make the declaration.
- In the class's body, we use the friend keyword to declare a friend function.
Syntax
Code
Since the method printLength() in the preceding example is a friend function of the class Box, we can see from the output that it is successfully able to access and alter the class Box's data members even though it is defined outside the scope of that class.
Like friend functions, we can also create friend classes.
Advantages
- The friend function enables the programmer to create a program that are more effective.
- It enables a non-member function to share confidential class information.
- It makes it simple to access a class's private members.
- It is frequently used when several classes include members that are connected to other programme elements.
- It enables extra functionality that isn't frequently utilized by the class.