Mastering Fundamentals: C++ and Data Structures
Constructor vs Destructor in C++
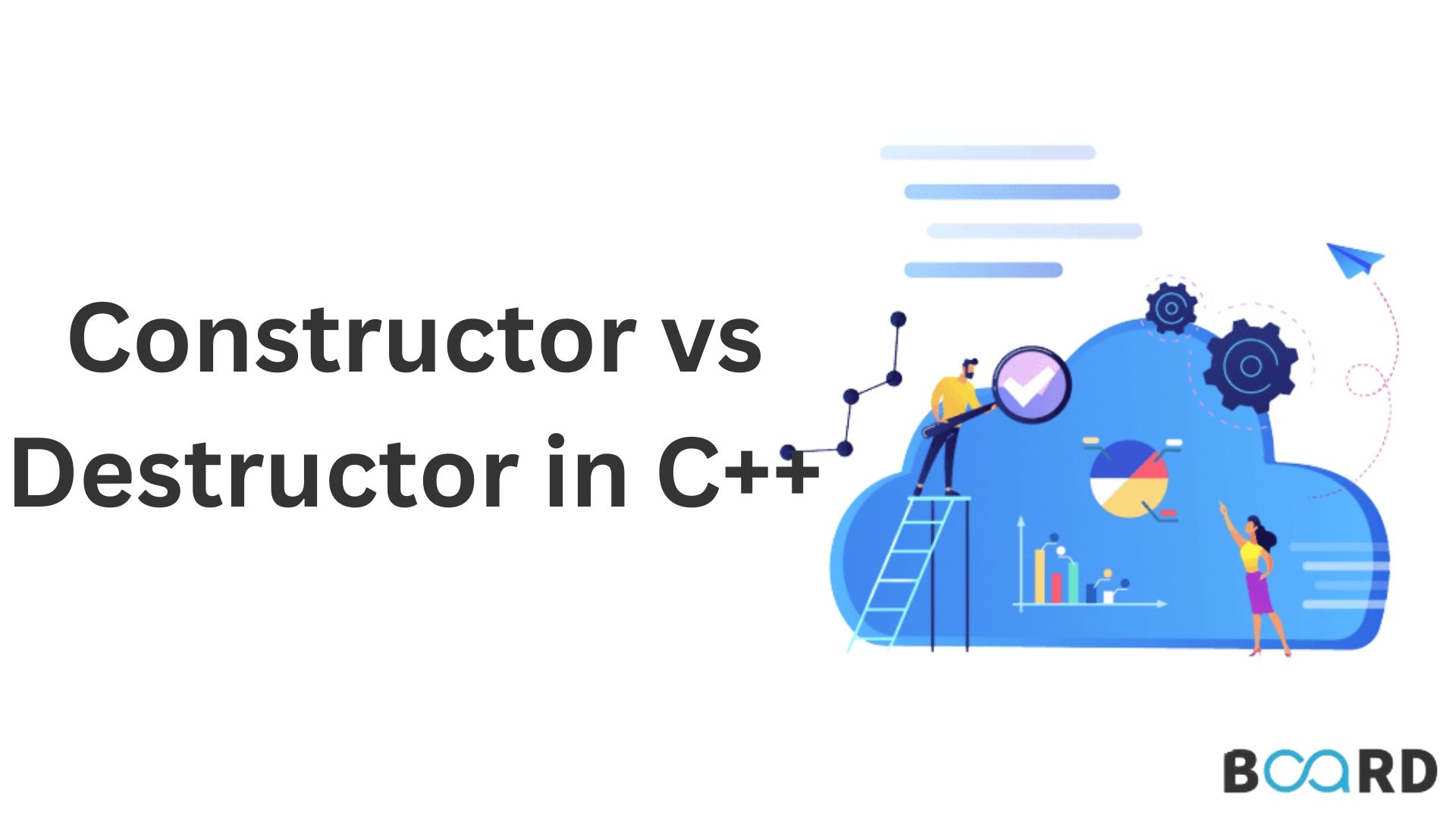
Introduction
One of the key methods for resolving issues using the concepts of classes and objects is OOPS or object-oriented programming. By combining the most well-structured programming features with a number of potent new notions, object-oriented programming (or OOP) is an approach to program organization and development that seeks to do away with some of the difficulties of traditional programming approaches. Data is considered the most important component of OOPS, and access to it is only permitted in certain circumstances and places. It strengthens the connection between data and the functions that use it and guards against unintentional alteration by external functions. One of the cornerstones of object-oriented programming is a function constructor and destructor.
Constructor
In essence, a constructor is a specific procedure that is automatically called when an object is created. The primary function of a constructor is to initialize class variables with values provided by the user or to provide default values in the event that the user chooses not to do so. The class name is also the name of the function constructor. The ability to return values is absent, though. As long as the signatures are distinct, a class may have more than one constructor. In other words, constructor overloading is supported, meaning that while the function constructor name can be used, the number, kind, and order of the parameters can vary from one signature to the next.
Syntax
Destructor
A destructor is a function of a class that, like a constructor, has the same name as the class name but comes before it with the tilde operator. It serves the opposite purpose from the function constructor and is used to reset the values of the variables. There is just one destructor available, and a class cannot overload it. Destructor calls are made in the opposite order of how function constructor calls are made. In the event of a parent class and child class, the destructor of the child class is called first, followed by the destructor of the parent class, as opposed to a function constructor, where the parent is called first and then the child.
Constructor vs Destructor
Conclusion
The concepts of a constructor, and a destructor, and the differences between them were dealt with in this exercise along with a code snippet to understand their working of them.