Mastering Fundamentals: C++ and Data Structures
List in C++
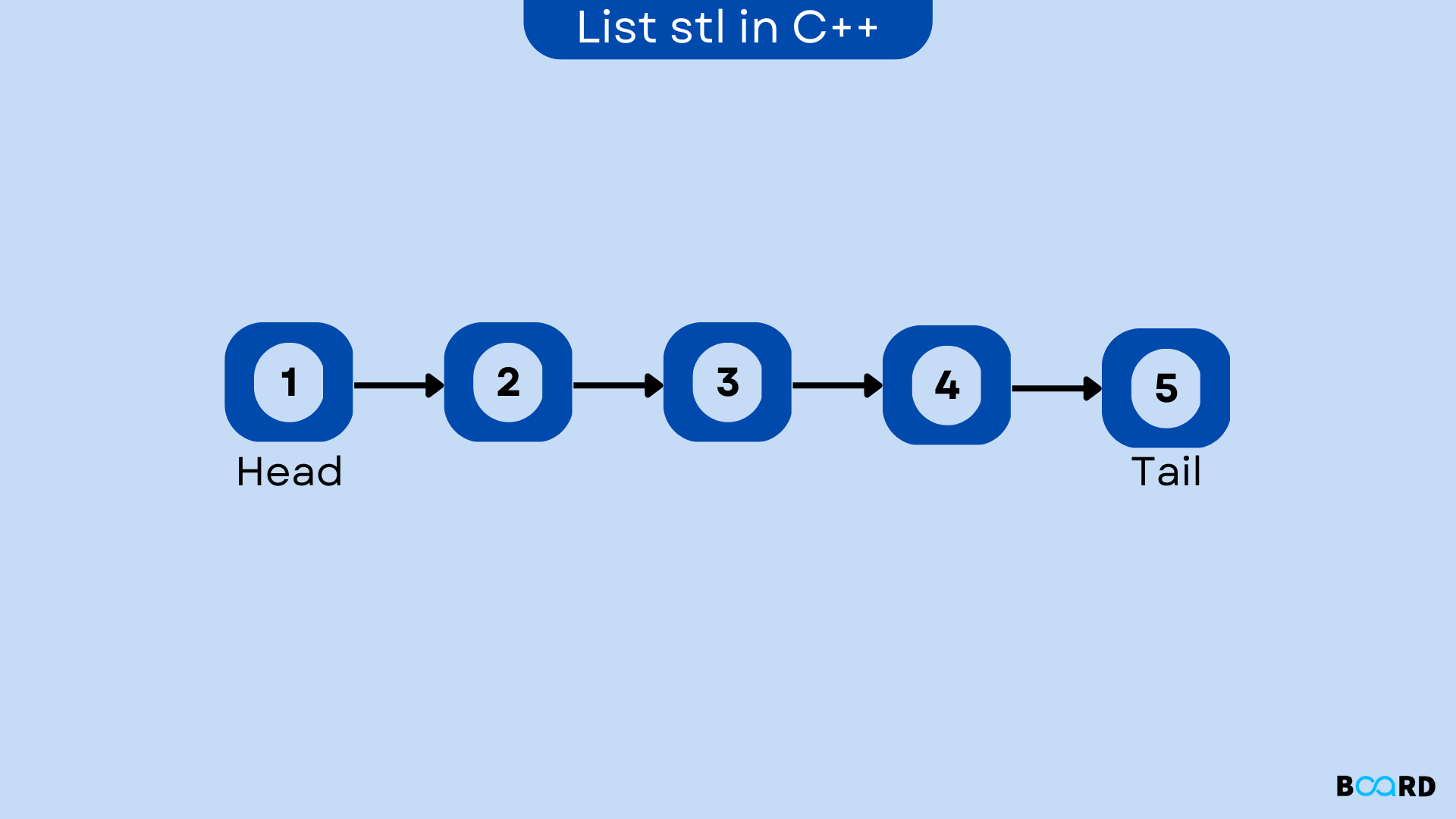
Introduction
The term "storage container" in C++ refers to the std::list. You can add and remove things from the std:list from any location. A doubly-linked list is used to implement standard::list. This indicates that bi-directional and sequential access to list data is possible. The STL (Standard Template Library) provides sequential access in all directions but not fast random access.
List components can be dispersed among various memory blocks. A container stores the data that is required for sequential access. During runtime, the std::list might grow and contract from both ends as necessary. The storage needs are automatically met by an internal allocator.
The best use cases for lists in C++ are:
- In comparison to array and vector, the std::list performs better.
- In terms of inserting, moving, and withdrawing pieces from any position, they perform better.
- Algorithms that heavily rely on these operations also perform better when used with the std::list.
Syntax
We must import the list> header file in order to define the std::list.
Here is a description of the above parameters:
- T – Defines the type of element contained. You can substitute "T" by any data type, even user-defined types.
- Alloc – establishes the allocator object's type. By default, this uses the allocator class template. It uses a straightforward memory allocation paradigm and is value-dependent.
Code Example
Code Example for Deletion of Elements
Summary of the List Data Type in C++
- The storage container is known as std::list.
- It permits the constant deletion of things as well as the insertion of things from any location.
- The system is set up as a doubly-linked architecture.
- Both sequential and bidirectional access is possible to the std::list data. Fast random access is not supported by std::list.
- It does, however, permit sequential access in every direction.
- List components from std::list can be dispersed across many memory blocks.
- Std::list can be shrunk or expanded from both ends as necessary during runtime.
- The insert() function is used to add entries to a std::list.
- Use of the erase() method is required to remove entries from the std::list.