Mastering Fundamentals: C++ and Data Structures
How to Sort a Vector in C++
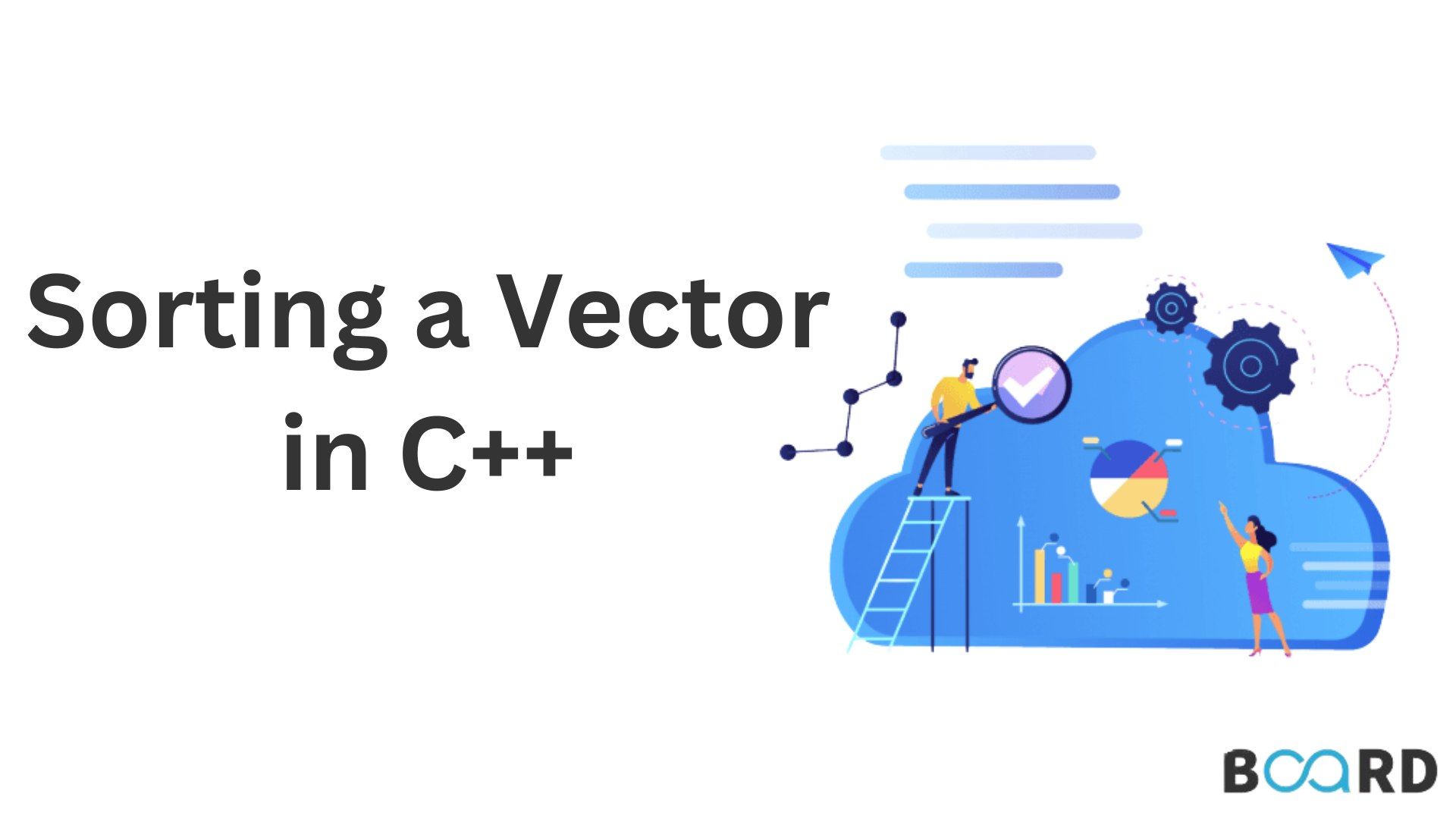
A vector in C++ is similar to dynamic arrays which are capable of resizing itself if required. The C++ stl provides us sort() inbuilt function to sort a vector
Sorting a vector in ascending order
The following syntax sorts a vector in ascending order:
Syntax
The above syntax sorts a vector in ascending order. Let us consider the following program,
Source Code
Output
Output Description
As you can see, the vector has been sorted in ascending order.
Sorting a vector in descending order
The sort() function accepts a third argument that sorts the vector in descending order. Its syntax is given below,
Syntax
Let us consider the following program:
Source Code
Output:
Output Description
As you can see in the output, the vector has been sorted in descending order.
Sorting a vector in a particular order
The sort() function also accepts a compare function using which we can sort a vector in any particular order. Its syntax is given below,
Syntax
sort(myVector.begin(), myVector.end(), compare)
The compare function should have bool return type and has the following structure:
Now let us consider the program that sorts a vector of pairs on the basis of first element:
Source Code
Output
Output description
As you can see in the output, elements have been sorted on the basis of first element of pairs in the vector
Conclusion
In this article, we discussed how can sort a vector in C++. In the end we also illustrated how we can sort a vector on the basis of a particular condition by passing a compare function to the sort() function. We believe that this article has surely helped you to enhance your knowledge.