Mastering Fundamentals: C++ and Data Structures
Header in C++
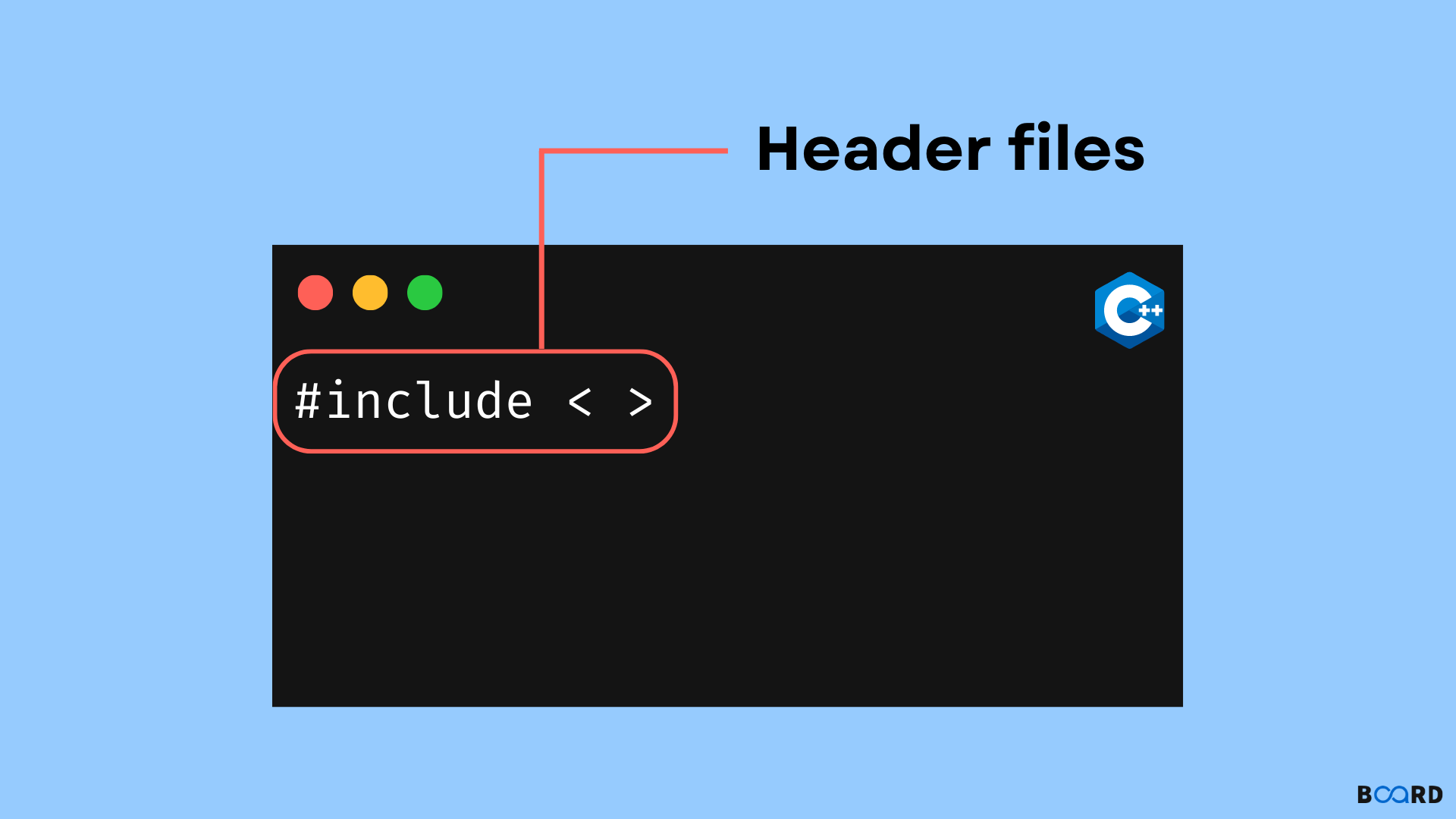
Introduction
One of the many features provided by the C/C++ to its users are header files. While all header files in C++ may or may not conclude with the.h extension, all header files in C are required to start with the.h extension.
In C/C++, a header file includes:
- Function definitions
- Data type definitions
- Macro
By importing these features into your program with the use of the preprocessor command #include, header files provide these functionalities. It is up to these preprocessor directives to inform the C/C++ compiler that certain files must be handled prior to compilation. The header file provides functionality of standard input and output and is used to accept input with the aid of the scanf() function and show the output with the help of the printf() function, must be included in every C program. C++ programs must include the header file, which is used to take input using the "cin>>" function and show output using the "cout" function.
Basically, header files are of 2 types:
- Standard library: These are the existing header files that the C/C++ compiler comes with by default.
- User-defined: The user can create header files that begin with #define.
Syntax of Header File in C/C++
There are two methods we may specify the header file's syntax:
#include<filename.h>
Angular brackets are used to surround the header file's name. It is the method used most frequently to define a header file. As was previously mentioned, all header files in C were required to start with the.h extension in order to avoid compilation errors, but this is not the case with C++.
Eg.
#include<string.h>
#include<vector>
#include"filename.h" / #include "filename"
Double-quotes are used to encapsulate the header file's name. Whenever defining user-defined header files, it is typically preferable.
Eg.
#include"stdlib.h"
#inlcude"iostream"
The main point is that we can not use the same header file twice in the same application.
Different C/C++ Header File Types
In C and C++, a lot of header files are included. We even have the ability to construct them based on our needs. Before developing the program's body, specific header files in C/C++ must be included in order to use the Standard Library functions. Here are some examples of C and C++ header files:
- #include<string.h> (String header) - Strcpy and other string manipulation techniques should be used.
- #include<conio.h> (Console input-output header) - Use console procedures such as clrscr() for screen clear and getch() to retrieve a character from the keyboard to input and output data to the console.
- #include<stdlib.h> (Standard library header) - Utilize common utility tools like malloc() and calloc() to carry out routine tasks like dynamic memory management
- #inlcude<iostream> (Input Output Stream) – used as an input and output stream.
- #include<iomanip.h> (Input-Output Manipulation) – Accessible through set() and setprecision ().
- #include<fstream.h> (File stream) – used to manage the data that is read from and written to files as input and output, respectively.
- #include<stdio.h> (Standard input-output header) - used to carry out operations on C's input and output, such as scanf() and printf ().
- #include<math.h> (Math header ) - Sqrt() and pow are examples of mathematical operations (). to determine a number's power and square root, respectively.
- #include<ctype.h>(Character type header) - Use character-type methods such as isaplha() and isdigit (). to determine whether the provided character is, respectively, an alphabet or a number.
- #include<setjmp.h> (Jump header) - execute jump operations.
- #include<stdarg.h> (Standard argument header) - Execute common argument functions, such as va start and va arg (). to specify the beginning of the program's variable-length argument list and to get the arguments from it, respectively.
- include<errno.h> (Error handling header) - Used to carry out tasks like errno for error handling (). By first setting val to 0, then afterwards modifying it to indicate mistakes, it is possible to highlight flaws in the programme.
- #include<time.h>(Time header) - Use date and time-related methods like setdate() and getdate (). to retrieve the CPU time and change the system date, respectively.
- #include<assert.h> (Assertion header) - It is utilized in assert and other software assertion routines (). To obtain an integer data type in C/C++ that only outputs to the standard error if the argument is 0.
- #include<locale.h> (Localization header) - Execute localization operations such as setlocale() and localeconv (). to, respectively, configure the locale and obtain the locale conventions.
- #include<signal.h> (Signal header) - Execute signal handling operations such as signal() and raise (). to install a signal handler and, correspondingly, to increase the signal in the programme