Dictionary to JSON: Conversion in Python
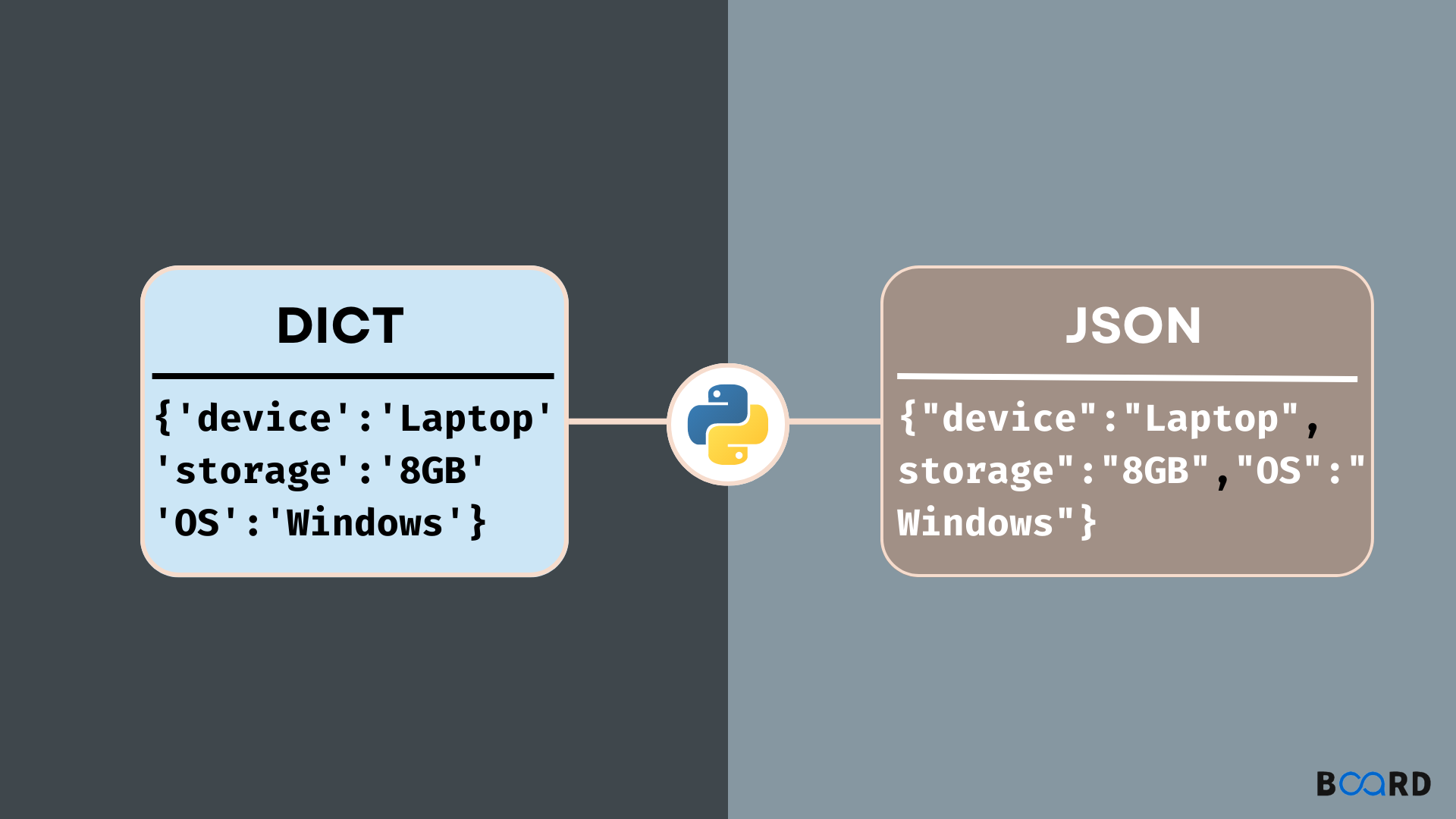
Introduction
Do you know how we can convert Python dict to JSON? If not, then you are at the right place. Board infinity got your back.
In this article, we will discuss Python dict or Python dictionary conversion to JSON. We will also discuss its implementation in Python. Most programs require data to function in anything. This information is either given to the software while it runs or has always been a part of it. One method for organizing and handling this data is to save it in JSON. A Python dictionary can store a series of elements concurrently in a well-formatted manner, just like JSON.
Let us understand what JSON is in Python.
JSON in Python
JSON (Javascript Object Notation) is a commonly used format for transferring data as text over a network. Data can be exchanged and stored using the JSON syntax over a network. It extensively uses databases and APIs that are simple to read and understand by humans and machines. To work with JSON data, you can use the 'json' package with Python. You must import the JSON package in Python programming to use this feature.
Python JSON is similar to a python dictionary in that it stores data in key-value pairs enclosed in curly brackets ({}). However, double quotation marks around the JSON key are required in this case.
Let us take an example to understand JSON in Python.
Output:
Now let us understand what a dictionary is in Python.
Dictionary in Python
Python uses the dictionary data type to store the data sequence in a single variable. Because no other data type supports holding more than one value as an element per element, the Python dictionary helps store data values like a map. A dictionary is a list of data elements that can be changed and is stored as key-value pairs inside curly brackets ({}). The key associated with each value is represented by the colon (:) in this case.
While dictionary keys are unique and unchangeable, the values of a dictionary can be of any data type and allow for duplicate values.
Let us understand the dictionary in Python with the help of an example.
Output:
Now let us discuss how to convert Python dict to JSON.
Python dict to JSON
We will discuss the conversion of Python dict to JSON using the Python dumps() method.
By importing the "json" module, the dictionary can be transformed into a JSON object using the built-in dumps() function of Python's default module, "json." It is simple to parse JSON strings that contain JSON objects thanks to the "json" module. A Python dictionary is transformed into a JSON object in the example below. Here is the implementation of it.
Output:
Conclusion
In this article, we have discussed Python dict to JSON conversion. We have discussed what JSON in Python is. We also discussed what a dictionary is in Python with the help of examples. We have also seen the implementation of Python dict to JSON conversion with the help of the dumps() method.