Pandas append() in Python
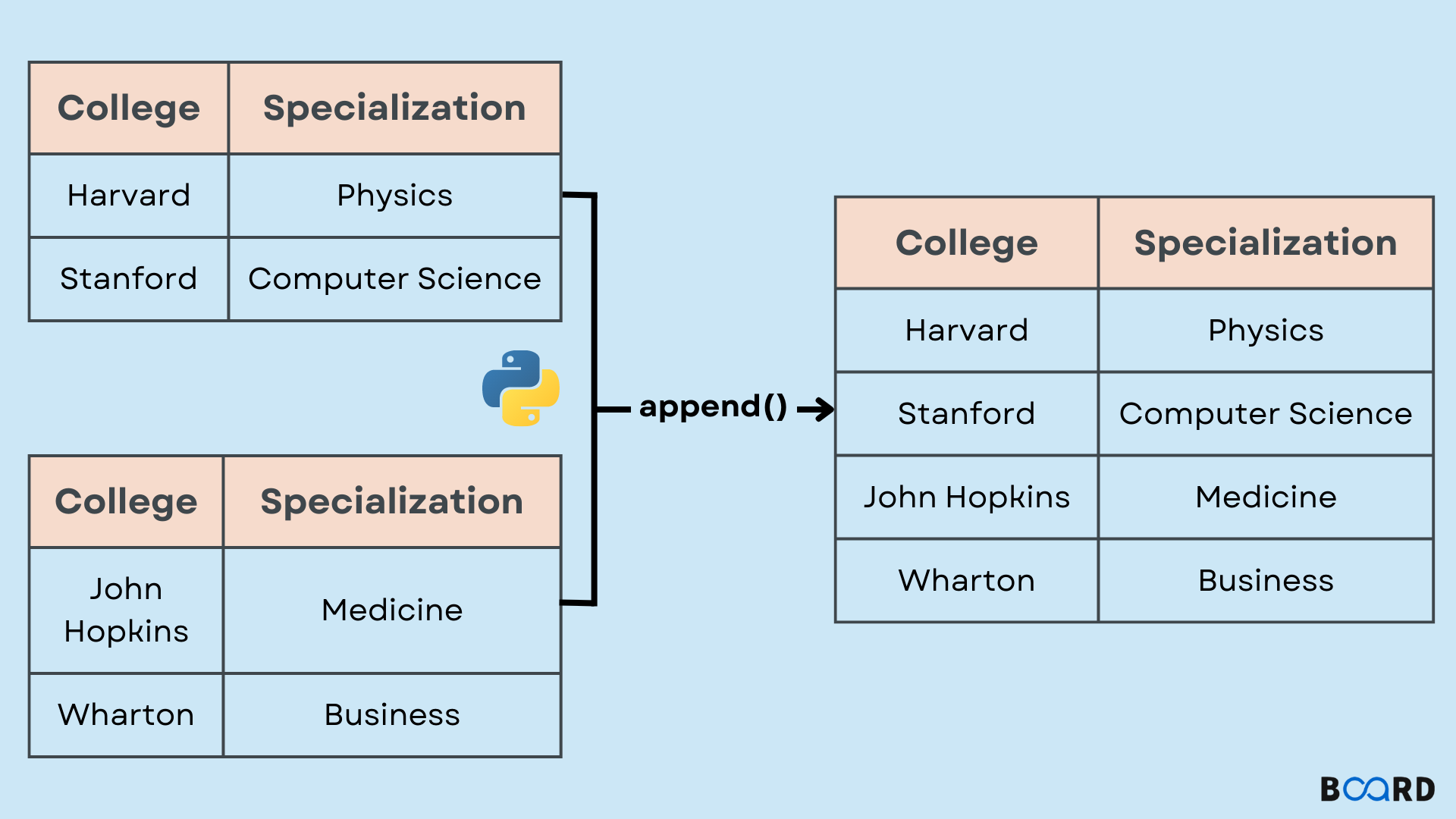
What is Pandas DataFrame append() method in Python?
Pandas package provides a wide variety of Tools for Data Analysis. One of the most popular Data Structures in Pandas is DataFrame, which has huge applications in handling datasets such as importing data from storage and files.
But, as per the varying business requirement, you may need to add some more rows and columns in the DataFrame so that it can store some more information. For this, Pandas DataFrame append() method is used.
The DataFrame append method appends the other DataFrame in the end and returns a new DataFrame object. Along with the DataFrame which you want to append, this method also has the following parameters:
- Ignore_index: If this is declared true, then the index labels of the resulting DataFrame are labeled as 0 to n-1, where n is the total number of indices in the resulting DataFrame.
- Sort: If this is declared true, the resulting DataFrame is returned in sorted order.
- Verify_integrity: If this is declared true, A ValueError will be raised if there are duplicated indexes in the DataFrame.
Code Implementation
Let’s see the implementation of the Pandas DataFrame append method with the help of an example. We have created two sample DataFrames which will be appended using this method. For dataframe1, we have used an excel file and DataFrame.head() method to get the first five rows of DataFrame.
The dataframe2 has been created using the dictionary data structure in Python.
We will also see the above-mentioned parameters while appending the second DataFrame to the first.
In the output shown above, there is no change in the index of labels but if you declare the ignore_index property, then you will get the following output in which the labels have been changed to a range of 0 to n-1.
Now, in our DataFrame, there are repeated index values in both the DataFrames. If the verify_integrity property is set to be true, ValueError is raised.
If you want to return the DataFrame in the sorted order, use the sort property and set it to true. In the following output, the resulting DataFrame is sorted.
How to append rows and columns to DataFrame?
If you want to add some specific rows or columns in the Pandas, then creating a separate DataFrame would not be an efficient solution. In such a case, you have to directly append the rows to DataFrame without using the Pandas DataFrame append method.
First, let’s see how you can append columns in Pandas DataFrame. You can simply append columns using the label and assign a list to them, as shown below.
Another method is using the Pandas assign() method. You just need to pass the column name and list to it and the assign method will add the column to it.
Now, let’s see how to append rows in the DataFrame. This can be done using the Pandas DataFrame append method.
Since the new row added should be part of the DataFrame, we have declared the ignore_index property as True.
To sum up, you can append a DataFrame to another DataFrame, a row to a DataFrame, or a column to a DataFrame as per the use case. If you want to add a column to a DataFrame, use DataFrame assign() method and if you want to add rows, use DataFrame append() method. Both methods return a new DataFrame object with the added fields.