Python Docstring
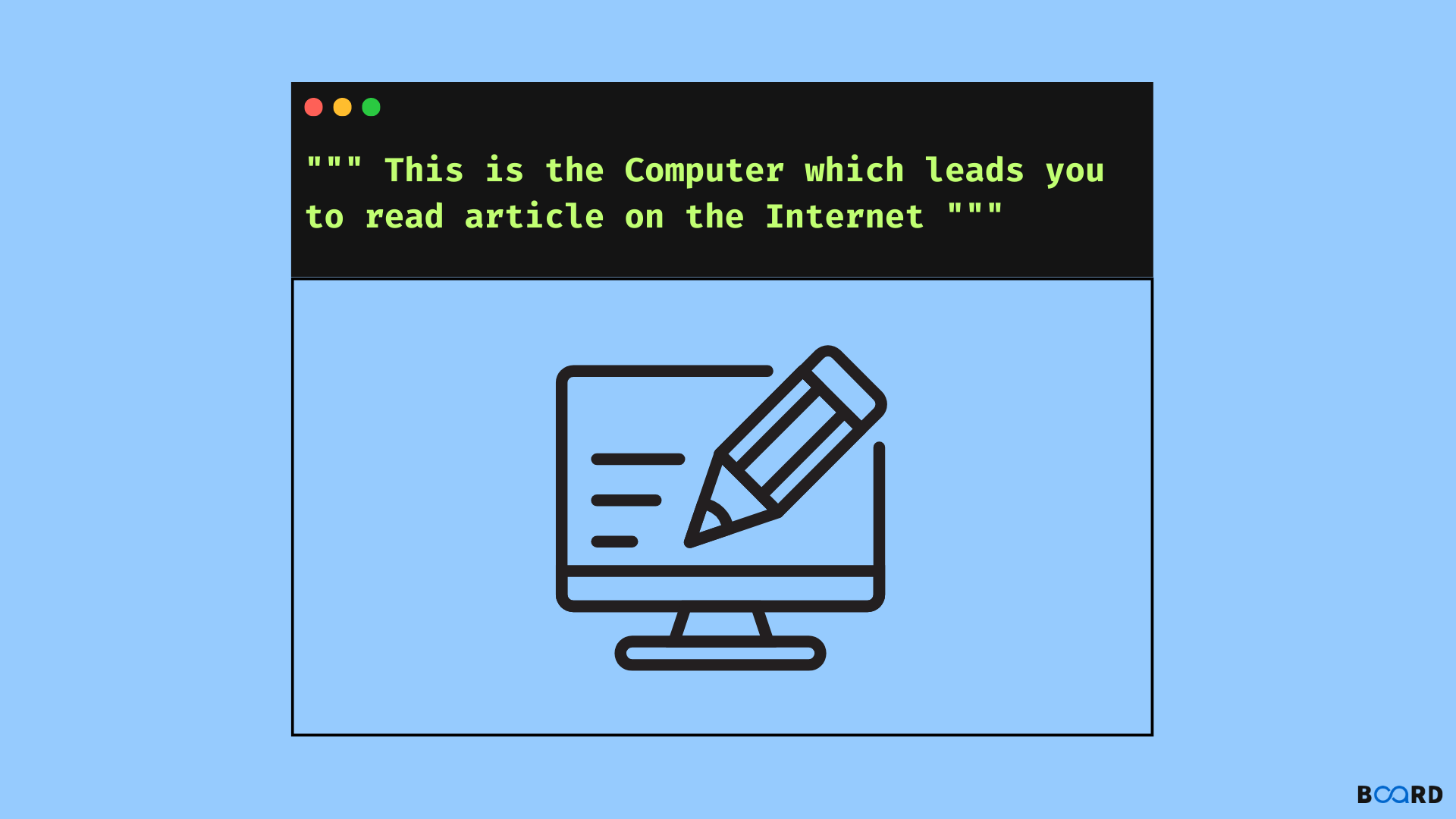
Introduction
Python docstring stands for Python documentation strings, it gives us a convenient path to associate documentation with python modules, methods, functions and classes.
Python docstrings are specified in the source code. It is used as a comment or also used to document a certain block of code. Not like the conventional code comments, Python docstring needs to describe not how the function works but what the function does.
Types of Docstrings
Declaring Docstrings
In Python, we can declare docstrings using triple quotes either single or double.
Python docstrings are declared just below the class, function or method declaration, mentioning what that class, method or function does. All functions should contain their docstring.
Accessing Docstrings
By using the “doc” method of the object, docstrings can be accessed in Python. The doc method can also be accessed using the help function.
- The example below shows how to declare and access a docstring using single quotes.
Code
Output
The example below shows how to declare and access a docstring using double quotes.
Code
Output
One-line Docstrings
Now in Python, when we declare docstrings in one line that docstring becomes a one-line docstring. They are used in obvious cases. The closing quotes are also on the same line as the opening quotes.
Code
Output
Multi-line Docstrings
In Python, there are multi-line docstrings similar to one-line docstrings which are used to write a more elaborate description or to write a summary, followed by a blank line. The code below demonstrates multi-line docstring.
Code
Output
Difference between comments and docstrings
In Python, comments are used to describe the code so that the user who reads the code understands the source code. It describes the logic or a block of code. It can be written by using a #, on the other hand, Python docstrings give us a convenient path to associate documentation with python modules, methods, functions and classes. Python docstrings are specified in the source code.