Iterators in Python
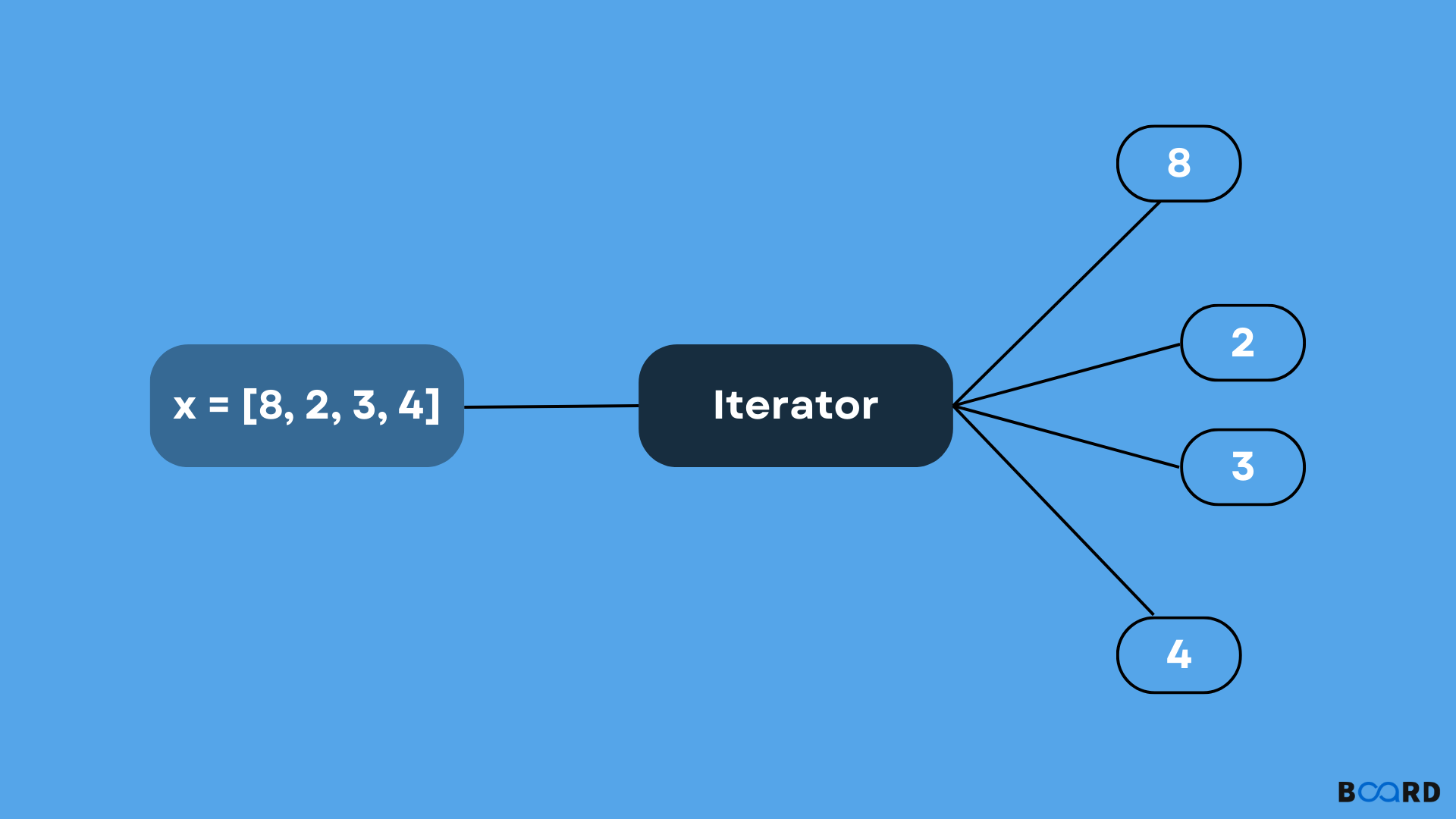
In python, an object called an iterator is used to iterate over iterable objects such as lists, tuples, dictionaries etc. To initialize this object we need to use the iter() method. For iteration, this object uses the next() method.
iter() method
This method is used for initializing the iterator. This method returns an iterator object.
next() method
For getting the next value of the iterator we use the next() method. While using a loop over an iterable object, it makes use of the iter() object to get an iterable object which after uses the next() function to get the next method to iterate over objects. To signal the end of the iteration it raises a StopIteration signal to do so.
Python iter() example
Code
Output
Examples
Creating and looping over an iterator using iter() and next()
In the code below, there is a custom iterator that will create an iterator type to iterate over a given limit (from 10 to a certain limit(). Suppose the limit is 13, then it will print 10, 11, 12, and 13. Also if the limit is less than 10, then it will print nothing.
Code
Output
Iterating over built-in iterables using the iter() method
In the code below, we manage the iterate state and iterator internally, that is we can not see it. We manage t using an object i.e. iterator to traverse over built-in iterable objects such as lists, tuples, dict and sets.
Code
Output
StopIteration Error
While iterating over an iterable object, we can iterate over multiple times, but when all items have iterated successfully it will raise a StopItertaion error.
Code
Output