Numpy in Python
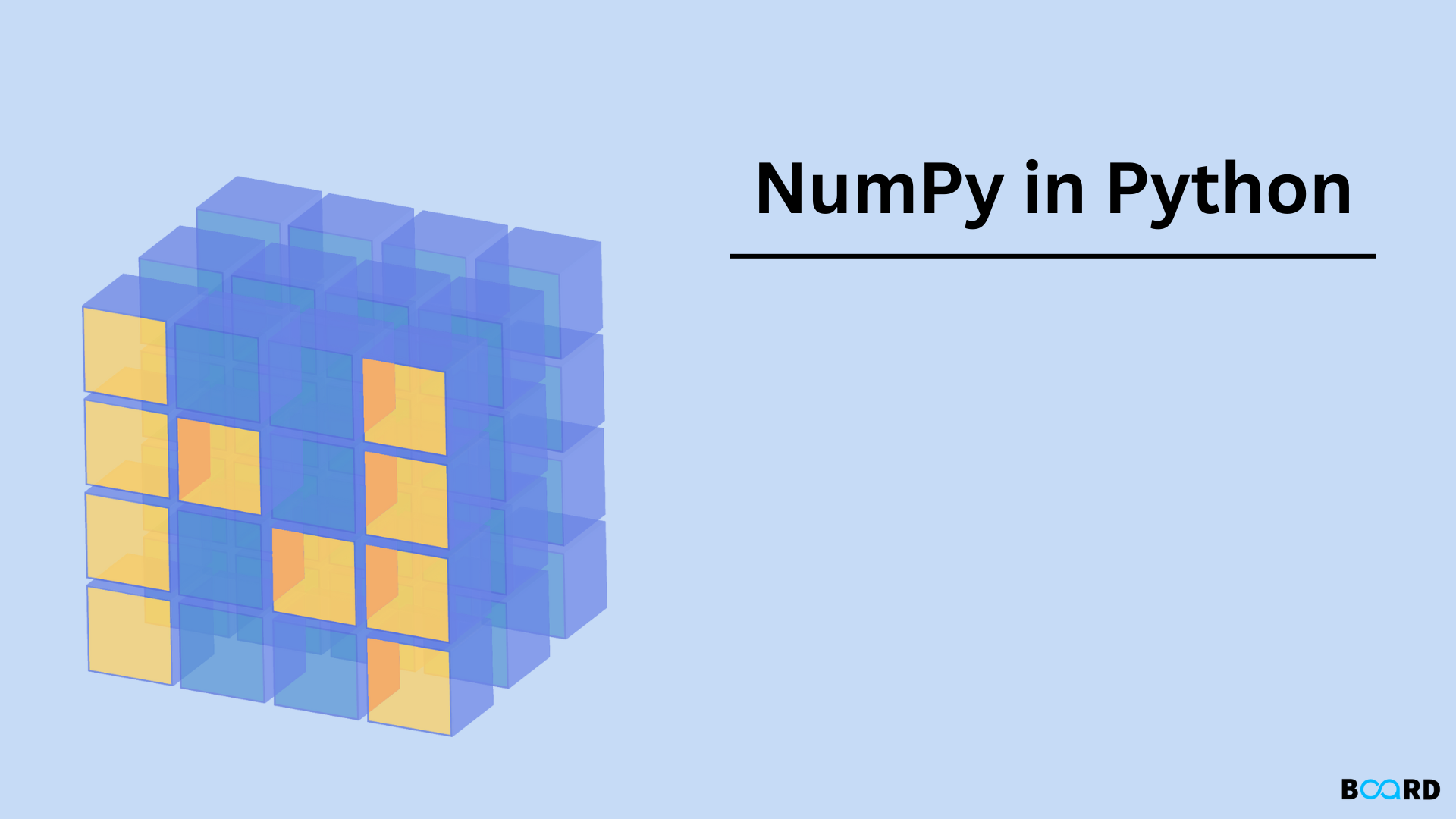
Introduction
NumPy is a popular Python library for numerical and scientific computing. It provides a powerful array object, which can hold and manipulate large arrays of homogeneous data. NumPy provides fast, efficient array operations and a wide range of mathematical functions that can be applied to these arrays.
Key Features of Numpy
Some of the key features of Numpy in Python are :
- N-Dimensional Array: The core data structure in NumPy is the ndarray, which is a multidimensional array of homogeneous data. Ndarrays are fast and efficient, and can be used to perform a wide variety of operations.
- Broadcasting : NumPy allows for broadcasting, which is a powerful way to perform operations on arrays with different shapes and sizes.
- Mathematical Functions : NumPy provides a wide range of mathematical functions, including trigonometric, logarithmic, and exponential functions, as well as functions for linear algebra, Fourier analysis, and more.
- Input/Output : NumPy provides functions for reading and writing data to and from files, as well as functions for interacting with databases and other data sources.
In this article, we will understand the basics of numpy and apply it on different types of arrays. The topics covered in this article are: Array Creation, Indexing of arrays and Basic Operations on Arrays using the numpy module in Python.
Let’s start with installing the Numpy module in Python. We will use the “pip” command to install the package and import it.
Numpy Arrays
The primary objective of Numpy in python is to help create multi-dimensional arrays.
Example:
This is a 2-Dimensional array which has 3 rows and 3 columns, i.e. a 3 X 3 matrix.
Creating Arrays in Numpy Python
NumPy provides various functions for creating arrays in Python. Here are some of the commonly used functions to create arrays in NumPy:
We will import the installed numpy module using the import command.
np.array():
This function creates a NumPy array from a Python list or tuple.
np.zeros():
This function creates an array of all zeros.
np.ones():
This function creates an array of all ones.
Complex Array
You can create a complex array in NumPy using the np.array() function and the dtype parameter set to complex
These are just a few examples of the functions available in NumPy for creating arrays. NumPy provides many other functions for creating arrays with different shapes and values.
Indexing Arrays using Numpy in Python
Indexing in NumPy arrays works similarly to indexing in Python lists. You can access elements in a NumPy array by specifying the indices of the elements you want to access inside square brackets [].
Example:
Output
In the above example, we create a two-dimensional NumPy array a with three rows and three columns. We then access the element at row 1, column 2 using a[1, 2], which returns the value 6. We also access the first two rows and all columns using a[:2, :], which returns the values [[1, 2, 3], [4, 5, 6]]. Note that the : symbol means "all indices" in that dimension.
Basic Operation On Arrays in Python
In this section, we will cover the basic operations that can be performed on arrays. Here are some of the most common operations.
Element-wise arithmetic operations: You can perform arithmetic operations on NumPy arrays element-wise using operators like +, -, *, /, and ** (exponent)
Output:
Mathematical functions: You can also apply various mathematical functions to NumPy arrays using functions like np.sin(), np.cos(), np.exp(), np.log(), and more
Output
Aggregate Functions: You can also apply various aggregation functions to NumPy arrays to compute statistics like mean, median, maximum, and more.
Output:
These are just some of the many basic operations that you can perform on NumPy arrays. NumPy also provides many more advanced functions for linear algebra, Fourier transforms, and more.
Conclusion
In this article, we have seen the basics of Numpy module in Python and covered the important topics in Numpy arrays. We have covered array creation, indexing, basic operations, and mathematical and aggregation functions, and illustrated using various examples