Dictionary of Lists
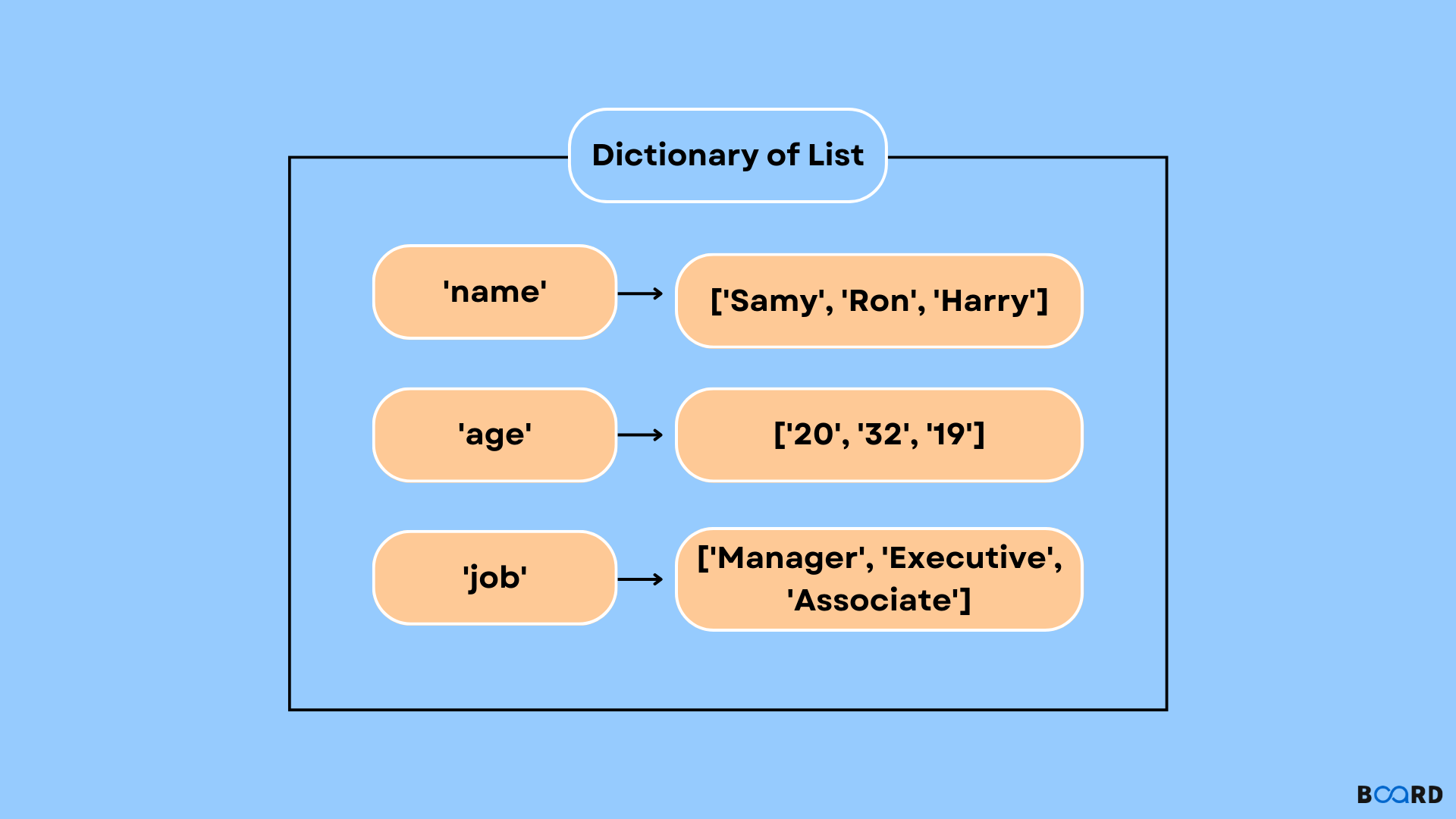
Introduction
A dictionary of lists is a data structure that consists of a collection of lists, where each list is associated with a key. The key serves as an identifier for the list, allowing you to access the list by specifying the key.
For example, you might have a dictionary of lists that stores a list of names for each year. The keys in the dictionary would be the years, and the values would be the lists of names. You could then access the list of names for a particular year by specifying the year as the key.
Example
Here is an example of how you might create a dictionary of lists in Python:
In this example, the dictionary names_by_year has three keys: 1900, 1910, and 1920. Each key is associated with a list of names. You can access the list of names for a particular year by specifying the year as the key in square brackets (e.g., names_by_year[1900]).
Dictionaries of lists are useful for storing and organising data into groups or categories. They allow you to quickly and easily access the data for a particular group or category by using the key as an identifier.
Key points
There are a few other things you might want to know about dictionaries of lists:
- You can use any data type as a key in a dictionary as long as the data type is immutable (e.g., strings, integers, tuples). Mutable data types (e.g., lists and dictionaries) cannot be used as keys.
- You can use any data type as the values in a dictionary, including lists, dictionaries, and other data structures.
- You can add, modify, and remove elements from the lists in a dictionary of lists like you would with a regular list. For example, you can use the append() method to add an element to the end of a list or the insert() method to insert an element at a specific position.
- You can iterate over the keys, values, or both keys and values in a dictionary of lists using a for a loop. For example, you can use the items() method to iterate over both the keys and values in the dictionary:
This would output the following:
You can use the len() function to determine the number of keys in a dictionary of lists. You can also use the in operator to check if a particular key is in the dictionary.
Extras
Here are a few more things you might want to know about dictionaries of lists:
- You can create an empty dictionary of lists using the dict() function:
empty_dict = dict()
- You can also create an empty dictionary of lists using curly braces ({}):
empty_dict = {}
- To add a new list to a dictionary of lists, you can assign a value to a new key:
names_by_year[1930] = ['John', 'Karen', 'Lisa']
- To modify an existing list in a dictionary of lists, you can simply reassign the value for that key:
names_by_year[1900] = ['Alice', 'Bob', 'Charlie', 'Daniel']
- To remove a list from a dictionary of lists, you can use the del statement:
del names_by_year[1900]
- You can use the clear() method to remove all the lists from a dictionary of lists:
names_by_year.clear()
Conclusion
A dictionary of lists is a data structure that consists of a collection of lists, where each list is associated with a key. The key serves as an identifier for the list, allowing you to access the list by specifying the key. Dictionaries of lists are useful for storing and organising data into groups or categories. They allow you to quickly and easily access the data for a particular group or category by using the key as an identifier. You can use any data type as a key in a dictionary as long as the data type is immutable. You can use any data type as the values in a dictionary, including lists, dictionaries, and other data structures. You can add, modify, and remove elements from the lists in a dictionary of lists like you would with a regular list, and you can iterate over the keys, values, or both keys and values in a dictionary of lists using a for a loop.