with statement in Python
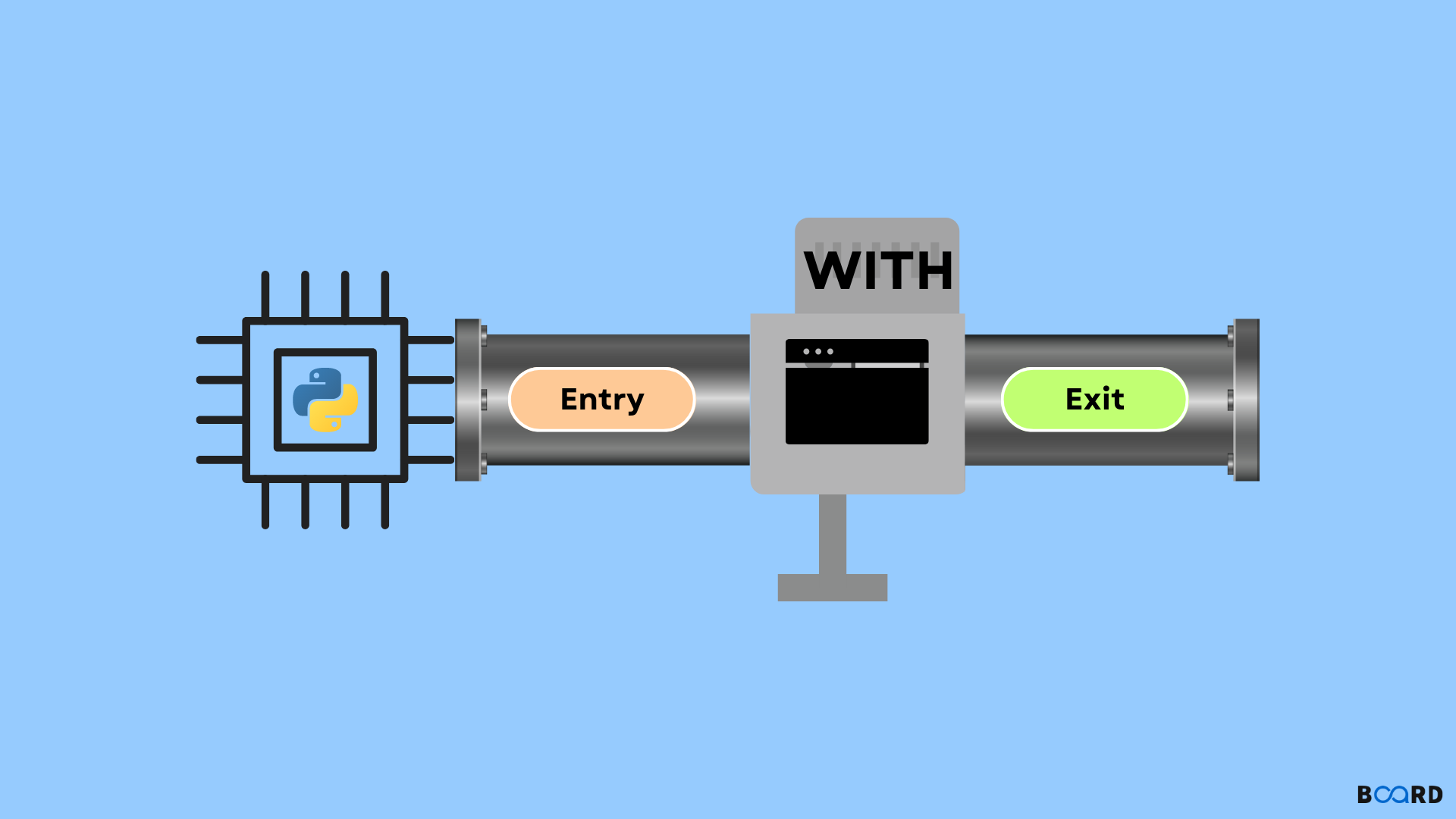
Introduction
For interacting with a file, the Python language includes several functions and statements. One of the statements and functions is the with clause, and another is the open() function. This article will teach you how to interact with files in Python using both the with statement and the open() method.
Understanding File Reading
In order to operate with a file in Python, you must first open the file. As a result, the open() method does what its name suggests by opening a file for you to use. You must first specify a variable for the open function before using it. The filename, mode, and encoding are the three arguments that the open() method can accept. The print function will then allow you to indicate what you wish to do with the file.
Not just that. You must additionally use the close() method to shut the file because the open() function does not do so. So, the open function should be used as follows:
Python's read mode is the default file mode, therefore the code above still functions properly even if you don't specify the mode:
How Does the With Statement Work in Python?
To open a file, the with statement collaborates with the open() function. Therefore, you can modify the code we used in the example of the open() function as follows:
The with statement automatically closes the file for you, unlike open(), which requires you to do it with the close() method. This is due to the with statement indirectly using the built-in functions __enter() and __exit(). After the specified operation is complete, the __exit()__ procedure closes the file. You may alternatively add to the file using the write() function, like I did below:
The text can also be printed line by line by iterating over the file:
Conclusion
You may be debating between using the open() function alone or the combination of with and open() to manipulate files. You should use the with statement in conjunction with open() because it automatically closes the file and requires less programming on your part.