Count Rows and Column with Pandas
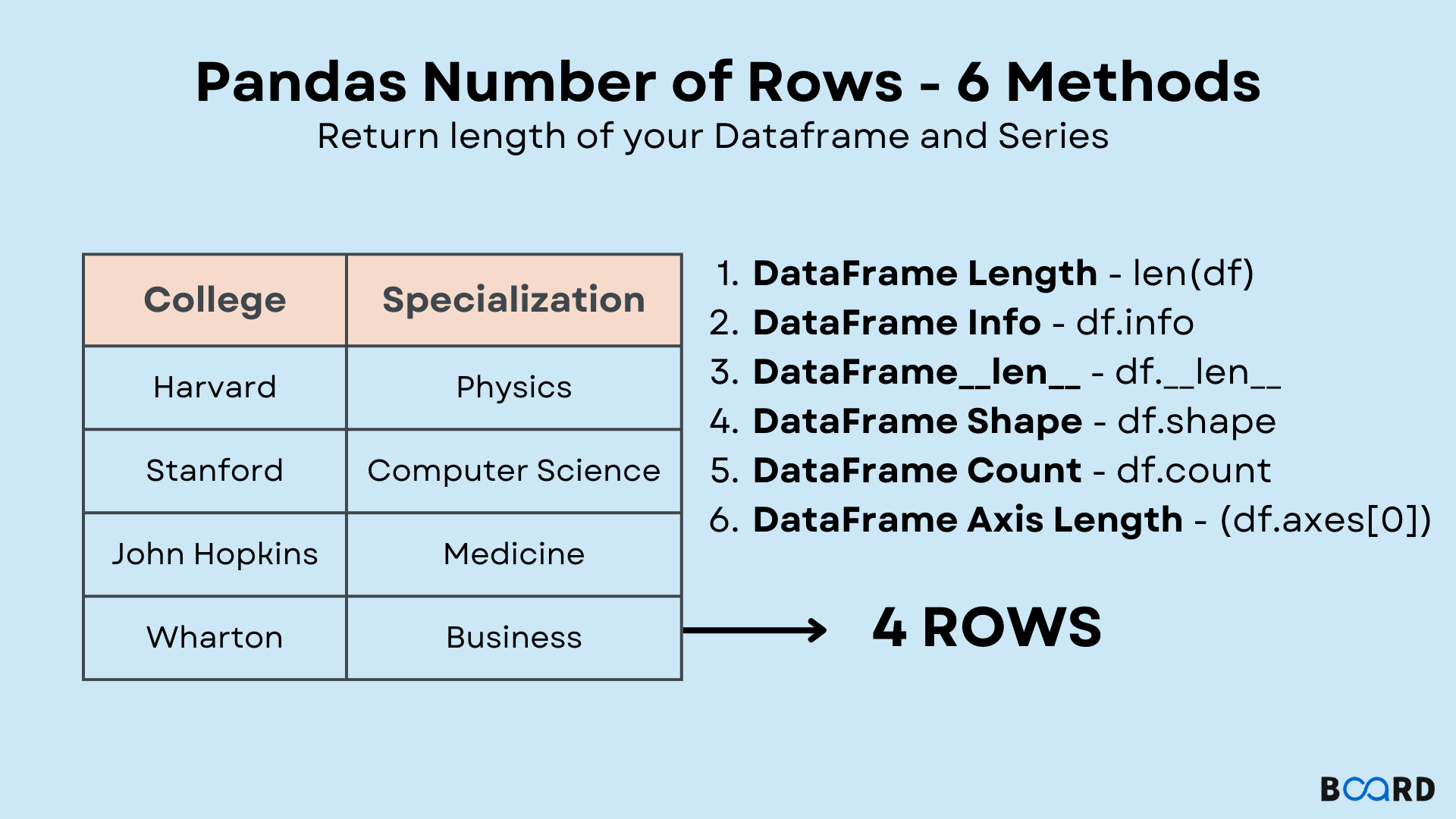
Introduction
In Pandas Library of Python, DataFrame is majorly used for loading huge datasets and manipulating them during Data Analysis operations. One of the common requirements while manipulating the data is to find the range of DataFrame, which means the number of rows and columns present in the DataFrame.
The Pandas Count Rows function is used for this purpose. There are also some other methods for counting the rows and columns in Pandas DataFrame, which will be discussed in this article. Let’s first see the python implementation of the Count Rows function in Pandas.
Counting the number of Rows in DataFrame
We have used an excel sheet to create a DataFrame. For simplicity, we have retrieved the first five rows of DataFrame using DataFrame.head() method in Pandas.
Following are the ways for Counting Rows in DataFrame:
- Using len() method: It takes the DataFrame index property and returns the number of rows.
- Using the axes() method: It returns the number of rows for argument ‘0’ and the number of columns with argument ‘1’ in Pandas DataFrame.
- Using the Shape() method: It returns the number of rows and columns in DataFrame in the form of a tuple.
- Using Pandas count() method: It returns the number of non-NA entries in the DataFrame. In our code, we have used Pandas Count Rows method as df[df.columns[0]].count(). This means that it will return the number of non-NA values in the first column.
Code Implementation
The implementation of the Python code is given below.
The output terminal of the Jupyter notebook shows the output.
Counting the Number of Columns in DataFrame
For Counting the columns in Pandas DataFrame, the following methods are used:
- Using the len() method: For counting the number of columns, the DataFrame column property is passed into the function.
- Using the Shape() method: Here, ‘1’ is passed to count the number of columns
- Using the axes() method: the argument ‘1’ is passed for counting the number of columns.
- Using the count() method: Here, the number of non-NA entries is returned for each row which signifies the number of columns. In other words, It returns the Series of non-NA entries for each row.
Code Implementation
The python implementation of the above approaches is given below:
The output of the above code in the Jupyter Notebook is given below:
Additional ways: Count Rows and Column
There is one more way of Counting the rows and columns in Pandas DataFrame which is using the DataFrame info() method provided by Pandas.
DataFrame info() is different from Pandas Count Row and Count Columns It prints a concise summary of the DataFrame in Pandas. As seen in the above output, the second line shows the number of rows in the DataFrame, and the third line shows the number of columns in the DataFrame.
Although these all methods are simple to use, the time taken for their execution is different from each other. You can see their runtime by using %timeit to see which method is faster and more efficient in a particular situation.
Similarly, for the Pandas Count Row functions, the time taken for methods is shown below.
Now, you can easily fetch the number of Rows and Columns of the DataFrame and use the functions as per the requirements.