GroupBy Python Function & How To Use It!
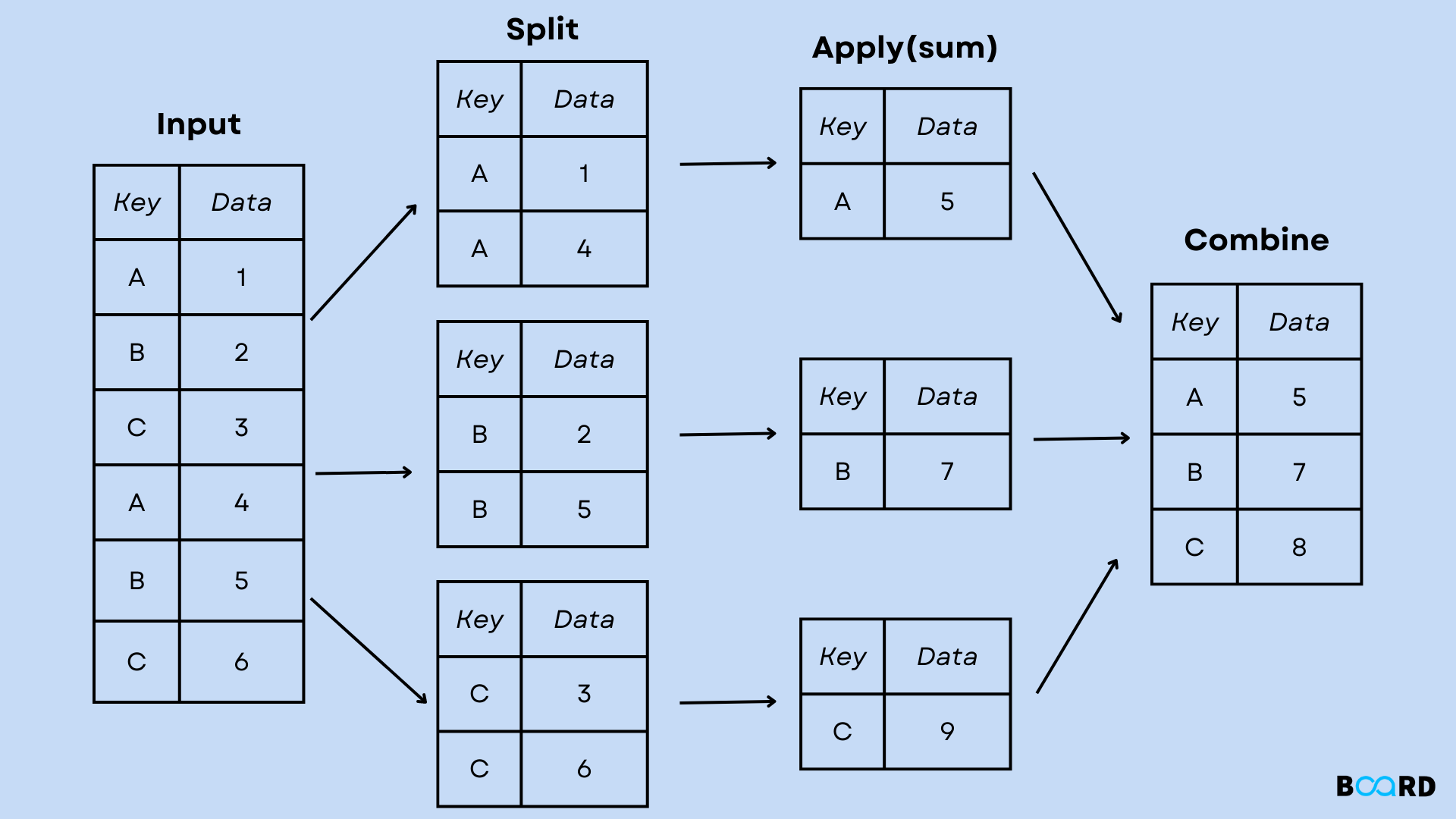
Introduction
One of the most well-liked Python libraries is Pandas. Data structures, a sizable number of built-in methods, and operations are all provided by Pandas for data analysis. It is primarily designed for intuitively and readily working with relational or labeled data. The pandas package has a wide number of in-built functions that let you operate on a huge dataset rapidly. In this post, we'll look at some built-in pandas library functions, along with an example and output, to show you how to count the number of rows in a pandas group effectively. So let's get going!
Pandas GroupBy Function
When working on data science projects, it's common to experiment with a lot of data and repeatedly test different procedures on datasets. The idea of groupby enters the picture at this point. By making your code more effective and efficient, groupby is the capacity to efficiently aggregate the provided data. Generally, groupby notion means:
- Splitting the dataset after performing various processes to create a group
- Applying the individually assigned function to each group
- Combining utilizing the groupby() function, each dataset's various results are combined into a data structure.
What if you want to count the number of rows in each of the groups that pandas groupby divides a given dataset into? It would be pretty difficult and impossible to count them by hand; therefore, let's look at some effective techniques that can assist you in this endeavor.
How Do I Count the Rows in Each Pandas Groupby?
The two techniques listed below may be used to determine how many items are present in groupby pandas:
1. groupby size()
Using the built-in pandas function called size is the most straightforward technique for pandas groupby count (). It gives back a pandas series with the overall number of rows for each group. The size() function is unaffected by NaN values in the dataset since its fundamental operation is the same as that of the len() method. Let's look at an example below for a better understanding: Take a look at the dataframe that contains the names of a group of students together with the topics they are taking.
Output:
Let's group the "Subjects" column in the dataframe mentioned above, and then use the groupby size() function to count the number of rows in each group.
For example:
Output:
As a consequence, the output for the aforementioned example shows the number of rows for each category in the dataframe according to the available subjects.
2. grouby count()
To count the values of each column in each group, you can alternatively use the pandas groupby count() function instead of the size() method. If there are no NaN values in the dataframe, take note that the number of counts is always close to the size of the rows. For a better understanding of the pandas grouby count() function, see the sample below:
For example:
Output:
In addition, if you are grouping the dataframe using a single column, you may use the value count() function.
For example:
Difference between Size() and Count() Methods
You must have decided after looking at the aforementioned examples to utilise the size() and count() methods interchangeably when dealing with pandas groupby. However, keep in mind that on its own, each of these approaches is fairly different. Since any NaN values detected by the count() method will be disregarded in this situation, the function returns the number of values in each group, which may or may not be equal to the number of rows. On the other hand, the size() function will, regardless of NaN values, return the real number of rows for each group in the dataframe. Let's use an illustration to clarify this:
For example:
Using the dataframe's "Students" column as an example, apply the count() function.
For example:
Output:
You must have realized from the example above that the size() method on groupby should be used to count all the rows in each dataframe, while the pandas groupby count() method should be used to count just the non-null values.
Conclusion
Python Pandas is an open-source package that offers powerful capabilities for data analysis and manipulation. However, to effectively use this capability of pandas, you must be familiar with a sizable number of its built-in libraries, which let you carry out certain operations on huge datasets. In this post, we looked at how to use built-in methods to count the number of rows in each group in a pandas group, making programming simple and effective even when dealing with large amounts of data.