Beginner's Guide to NumPy in Python
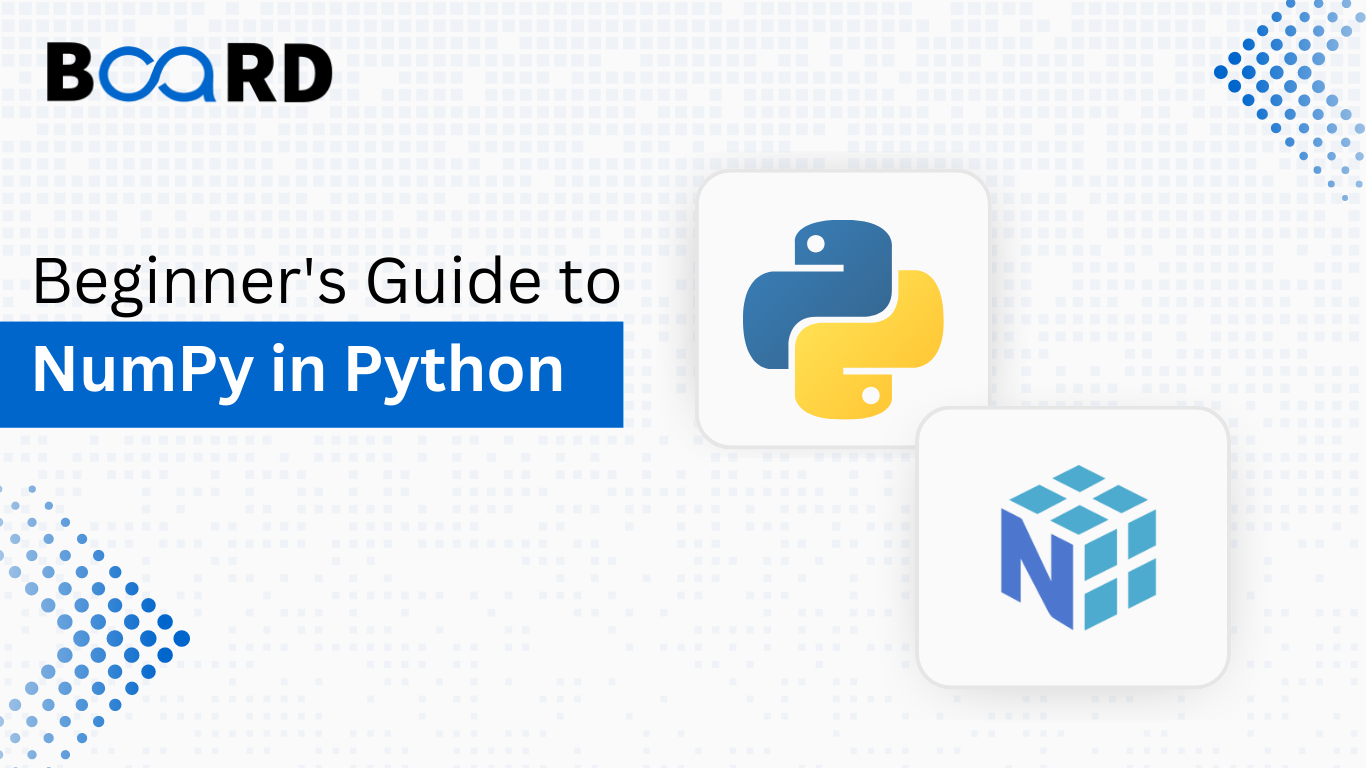
Introduction
NumPy library in Python is a built-in package that comes with a Python interpreter. NumPy is one of the most widely used Python packages for dealing with arrays and statistics formulas related to the collection of values stored in the form of a list or an array. In NumPy, the array data is stored in the form of a multidimensional array object. These array objects are highly optimized for providing high performance. Scientific computation and large data sets are dealt with in the Python NumPy package because it provides numerous highly optimized functions and methods for easy data manipulation. The NumPy library in the Python package has a lot of functions that can perform basic to advance level statistical operations in a very optimized time so that the result of any operation on the large data set can be obtained faster.
Why do we use the NumPy Library in Python?
The NumPy library in Python is an open-source package so its source code is easily available to all of us. Some of the numerous features that make it one of the most widely used Python packages are as follows:
- We can create powerful and highly optimized array objects of N-dimensions for example, 1-D array objects, 2-D array objects, etc.
- We have optimized functions that can be used to perform basic to advance level statistical operations.
- We can even integrate other programming languages (C, C++, Fortran) code using tools.
- We can perform some of the advanced level computations such as Fourier transformation, Linear algebra, Random number capabilities, etc.
- NumPy is used in the field of Scientific Data computation. To learn more about the use case of Python in Data Science, please refer to the article
- The Pandas package is developed on top of the NumPy package.
- We can even use the NumPy as a storage area for generic data.
- We can define arbitrary data types in the NumPy package and can also integrate databases.
As we have discussed that the NumPy library in the Python package is a built-in package that comes with the Python interpreter but in any scenario where we do not have the package installed on our system then we can use the following simple command to install the package on our system.
Note: You can access the NumPy code on the GitHub platform (https://github.com/numpy/numpy).
How is NumPy faster than list and other sequential Data Structures?
As we have discussed earlier that the array objects are stored in Numpy. These array objects are known as ndarray. The ndarray is stored sequentially so the data retrieval, access, and manipulation are very fast. On the other hand, the list data is not stored sequentially and hence is slower due to random access.
Example 1:
Output:
[1 3 5 7]
In the above example, we first imported the NumPy array so that it can be used in the function. The functions of the NumPy library in Python cannot be used without importing the library. There is one more way to import the NumPy library, we generally import the NumPy library with an alias np.
Example 2:
Output:
[2 4 6 8 10]
If we want to access the array elements then we can use the indexing method. The array indexing always starts with zero. So, if we look at the above example, element 2 is stored at index 0, element 4 is stored at index 1, and so on.
To randomly access the ndarray element, we can mention the array index in large brackets i.e. [] after mentioning the name of the array.
For example:
array = [45, 48, 51, 54]
array[0] = 45
array[1] = 48
array[2] = 51
array[3] = 54
Important NumPy Function in Python
So far we have discussed a lot about the NumPy library in Python like its use cases, working, etc. Let us now learn about the various important functions that make the NumPy library so popular:
1. __version__:
The __version__ attribute is used to get the version of the NumPy library stored on our system. It returns the version number in the form of a string.
2. ndim:
The ndim attribute is used to get the dimension of the ndarray.
3. dtype:
The dtype attribute is used to get the data type of the array.
4. astype():
The astype() function is used to change the data type of the array element. This method does not change the data type of the original array element but it returns a new array with the provided data type.
5. copy():
The copy() method is used to get the copy of the original array. If we change the data elements present in the copied array then the original array is not affected and vice versa.
6. view():
The view() method is used to get a view of the original array only. If we change the data elements present in the viewed array then the original array is affected as well because the view is just referring to the original array.
7. shape:
The shape attribute is used to get the index showing the number of corresponding elements in the form of a tuple.
8. reshape():
The reshape() function is used to change the dimension of the array elements. For example, if we have a 1-D array having 12 elements and we want to convert it into a 2-D array consisting of 4 arrays with each having 3 elements then we can use the function as reshape(4, 3).
9. nditer():
The nditer() function is a very powerful function that is used to perform basic to advance level iterations on the ndarray.
10. ndenumerate():
The ndenumerate() function is used to perform the iteration when we need to use the index number along with the indexed element
10. concatenate():
The concatenate() function is used to join two or more ndarrays into a single ndarray.
Data Types used in NumPy
We have a lot of extra data types which makes it easy to work with various types of data. The default data types of Python language like string, integer, float, boolean, and complex are still used in NumPy. The newer data types are:
- i - integer
- b - boolean
- u - unsigned integer
- f - float
- c - complex float
- m - time delta
- M - DateTime
- O - object
- S - string
- U - Unicode string
- V - a fixed chunk of memory for another type ( void )
Conclusion
NumPy library in Python is a built-in package that comes with a Python interpreter. NumPy is one of the most widely used Python packages for dealing with arrays and statistics formulas related to the collection of values stored in the form of a list or an array. The NumPy is an open-source package so its source code is easily available to all of us. The NumPy library offers optimized functions that can be used to perform basic to advance level statistical operations.