How to perform Matrix Multiplication with Numpy Python
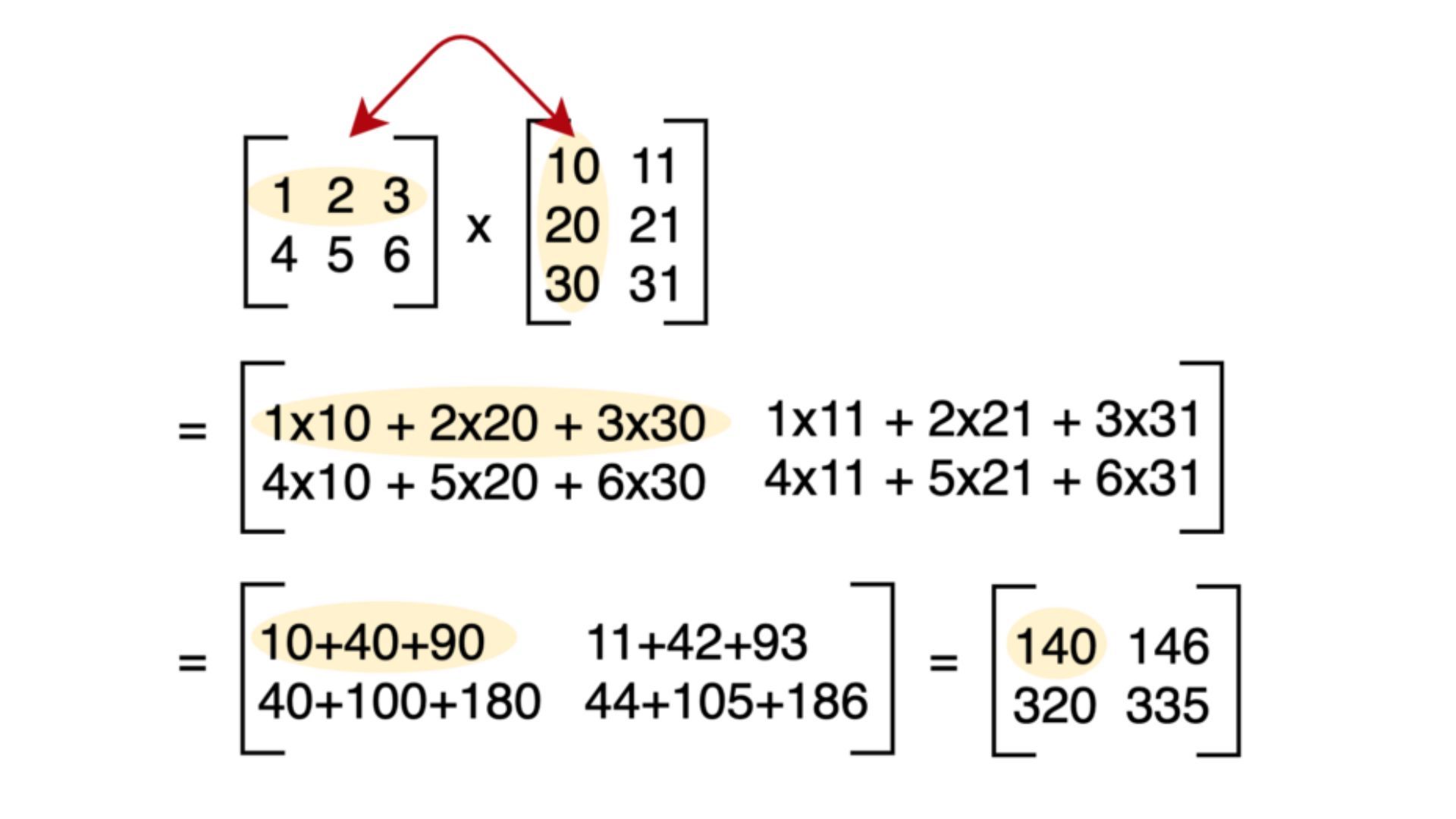
Introduction
A double rectangular array of data represented in rows and columns is known as a Python matrix. A matrix's data might consist of letters, integers, strings, formulas, symbols, etc. One of the crucial data structures that may be employed in calculations in math and science is the matrix.
By multiplying the rows of the first matrix by the columns of the second matrix, NumPy matrix multiplication creates a single matrix from two input matrices. NumPy has three alternative routines for multiplying two matrices.
- numpy.multiply(board_arr1, board_arr2) – Element based multiplication
- numpy.matmul(board_arr1, board_arr2) – Product of two matrices
- numpy.dot(board_arr1, board_arr2) – Scalar/dot product
Ensure that the first matrix's first column and second matrix's second row totals are equal before performing matrix multiplication in NumPy.
Quick Reference
Here are a few brief examples of NumPy matrix multiplication in case you're in a hurry.
1. NumPy.multiply()
Let's create some NumPy arrays and use them to multiply things element by element with the NumPy.multiply() function. This uses element-wise multiplication, also known as the Hadamard product, to multiply each element of the first matrix by its corresponding element in the second matrix. To multiply, make sure that both matrices have the same dimensions.
To supply a set of columns, rows, or submatrices to the numpy. Utilize the multiply() function to multiple certain rows, columns, and submatrices. The sizes of the submatrices, columns, and rows that we supply as our operands should be followed. Take this as an example:
2. NumPy.dot()
Scalar multiplication is a straightforward method of multiplying matrices, and we can accomplish this using the NumPy dot() function. A scalar can be multiplied by a matrix, or a matrix can be multiplied by a scalar, in scalar multiplication. The scalar is multiplied by each member of the matrix to produce an array with the same form as the original array. Order is irrelevant when conducting scalar multiplication.
Using np.dot, we may multiply a two-dimensional matrix by another two-dimensional matrix (). When multiplying two matrices, the operations should be performed in the correct sequence; for example, multiplying matrix X by matrix Y does not yield the same result as multiplying matrix Y by matrix X.
3. matmul()
In order to determine the matrix product of two arrays, use the np.matmul() function. The NumPy arrays' matrix multiplication is returned by the matmul() method, which accepts the inputs board_arr1 and board_arr2. Only when board_arr1 and board_arr2 are both 1-dimensional vectors is a scalar generated.
Conclusion
In this article, we have covered the idea of NumPy matrix multiplication in Python as well as how to use it with examples using the functions numpy.multiply(), numpy.matmul(), and numpy.dot().