Python Socket Programming
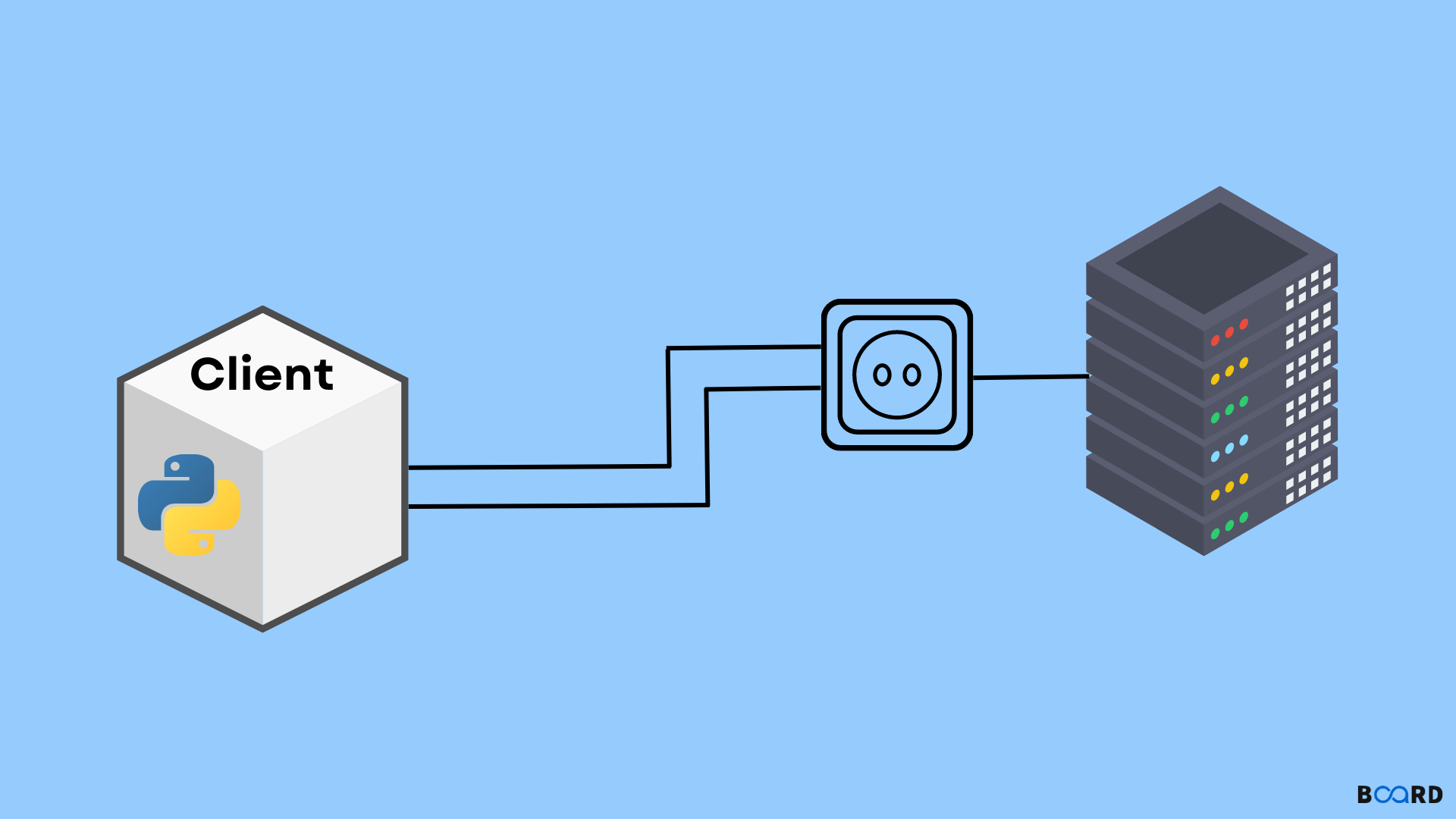
Introduction
Socket Server, Socket Client, and Socket are three fascinating subjects that we need to learn in order to comprehend Python socket programming. A server is what, then? A server, then, is a piece of software that watches for client requests and fulfills or processes them as necessary. A client, on the other hand, requests this service. A client software asks the server for some resources, and the server fulfills the request. The socket is where a server and client's two-way communication link ends. Sockets may communicate between processes on the same machine itself , between processes on separate machines, or within a single process. We must establish a connection through a socket port in order to communicate with any distant software.
Sample Python Socket
As was said before, a socket client requests resources from a socket server, and the server answers. Therefore, we will create a server and client model that can communicate with each other. The steps may be seen in this manner.
1)Initially, the Python socket server software runs and waits for any requests.
2) The initial conversation will be started by a Python socket client programme.
3) The server application will then respond to client queries as necessary.
4) The client programme will end if the user types "bye" in the box. The server programme will also end when the client programme does; however, we can choose to keep the server programme running indefinitely or terminate it at the client's request with a specific command.
Socket server in Python
The Python socket server software will be saved as socket server.py. We must import the socket module in order to use the Python socket connection. After that, we must take some action in order to link the server and client. The function socket.gethostname() can be used to get the host address. It is advised to use port addresses above 1024 because standard internet protocol uses port numbers greater than or equal to 1024. See the Python socket server example code below; the comments will aid in code comprehension.
So, port 5000 is where our Python socket server is running and waiting for client requests. Simply remove the if condition and break statement if you do not want the server to terminate when the client connection is ended. The server software is executed forever while waiting for client requests using a while loop in Python.
Socket client in Python
The Python socket client application will be saved as socket client.py. Except for binding, this programme is comparable to the server programme. The main distinction between a server programme and a client programme is that a server programme must bind a host address and a port address. See the Python socket client example code below; the comment will aid in code comprehension.