JSON file in Python: Read and Write
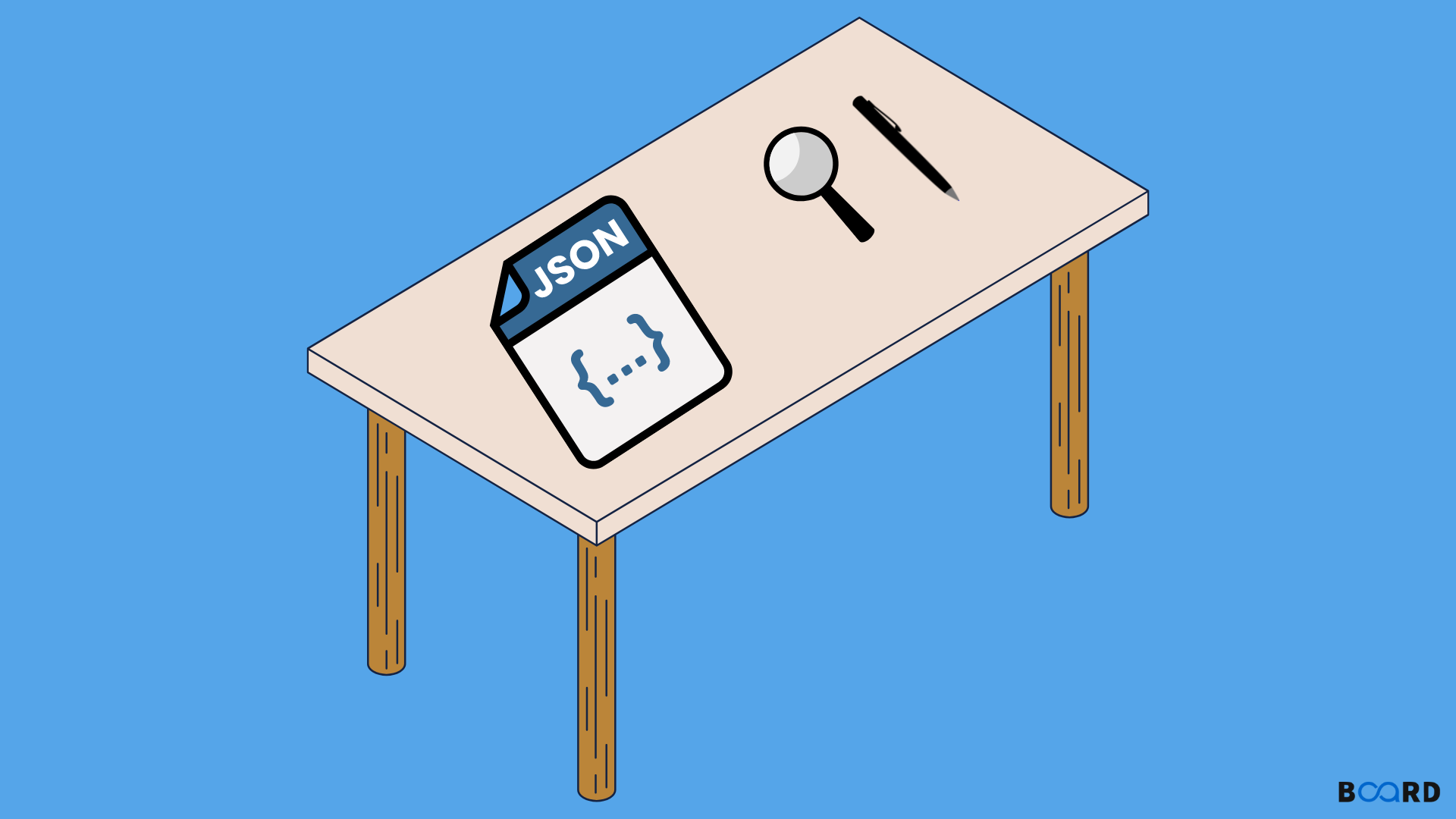
Introduction
JavaScript Object Notation is the Full form for JSON. It indicates that the data is stored and transferred using a script (executable) file that is composed of text in a programming language. JSON is supported by Python through the JSON built-in package. We import the JSON package into the Python script in order to use this feature. The quoted-string used in JSON contains the value in the key-value mapping inside of. It is comparable to Python's dictionary.
Writing JSON to a file in Python
Serializing JSON is the process of converting data into a sequence of bytes (hence serial) for storage or network transmission. It is simple to write data to files because the JSON library in Python uses the dump() or dumps() function to convert Python objects into the appropriate JSON objects.
Method 1: Writing data to JSON using json.dumps method
A function in Python's JSON package called json.dumps() facilitates turning a dictionary into a JSON object. There are two parameters:
- Dictionary: The name of the dictionary that needs to be transformed into a JSON object is dictionary.
- Indent: defines how many units should be indented.
Output:
Method 2: Writing data to JSON using json.dump() method
JSON can also be written to a file using the json.dump() method. Without first converting the dictionary into a JSON object, the "dump" function of the JSON package simply writes the dictionary to a file in the JSON format. There are two parameters accepted by json.dump():
- Dictionary: The name of the dictionary that needs to be transformed into a JSON object is dictionary.
- File Pointer: file pointer is the identifier for a file that has been opened in write or append mode.
Output:
Reading JSON file in Python:
Deserialization is the process of converting JSON objects into their corresponding Python objects and is the opposite of serialisation. It uses the load() method to do so. JSON data can be easily deserialized with load(), which is typically used to load from a string; otherwise, the root object is in a list or Dict. This depends on whether you used JSON data from another programme or obtained it as a string format of JSON.
Reading JSON using json.load() method
The json.load() function in the JSON package loads the contents of a JSON file into a dictionary. There is only one parameter required:
- File Pointer: file pointer is the identifier for the JSON file.
Output