Sort Dictionary in Python
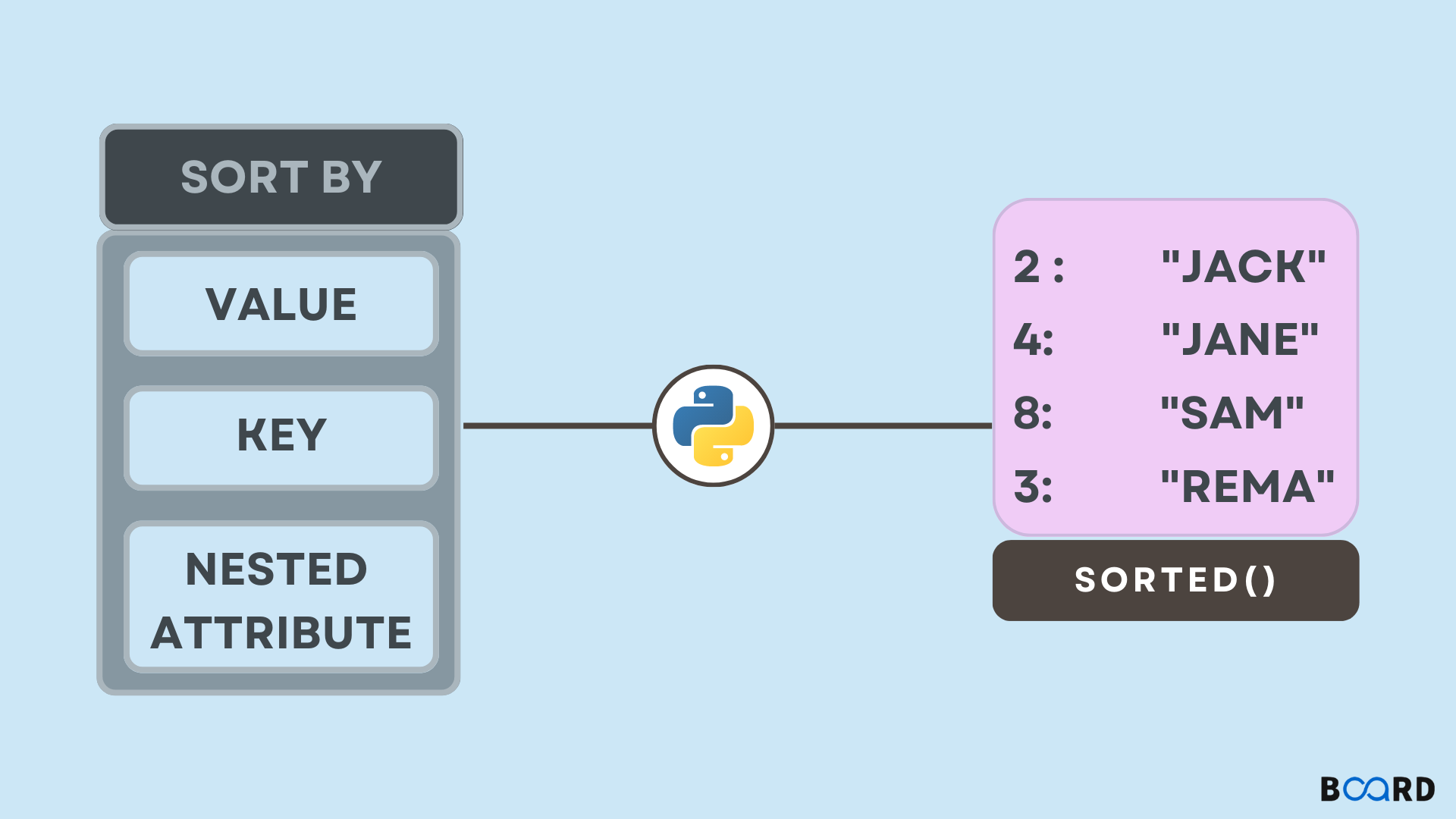
Introduction
Dictionary a Data Type in Python which stores the data in the form of key-value pairs. By default, a dictionary is unordered. All the keys in a Python Dictionary must be unique. However, the values associated with the Dictionary keys can be duplicated. But, while accessing the dictionary with duplicate values and unique keys, only one value associated with each key is printed.
See the code and its output below:
Output:
In this article, we will learn how to sort a Dictionary by value in Python. But, first, let’s create a Python Dictionary. Suppose dictionary keys are of character type and values associated with them are of type string. Then the program will be:
Output:
Sorting a Python Dictionary by Key
There are various ways to sort a dictionary in python. You can sort a Dictionary by value or by key in Python. To sort a dictionary by key, we can directly use the sorted method. In this method, the dictionary is first passed to the sorted() which returns the sorted dictionary.
But, the sorted() method in Python returns a list of tuples that contains the key-value pair of the dictionary. Thus, you need to again convert the list into the tuples using the dict() method in Python.
Following is the code for sorting a dictionary by keys in python:
Output:
Sorting a Python Dictionary by Value
You can also sort a dictionary by value in python. Suppose there is a dictionary that contains keys as numbers and values as strings. The method is the same as the previous one. The difference is that you have to pass another argument in the sorted() method which specifies the value of keys while sorting.
The key argument in the sorted() method specifies what should be considered while sorting the list. Here, we pass the lambda function as a key which specifies that the value of each key should be considered while sorting the list. The code for the above approach is below:
Output:
To reverse the order of sorting by value, pass Reverse=True as argument.
Output:
Need of Sorting a Python Dictionary
Sorting any list makes retrieval of data very quick for us. The value of the data in a Python Dictionary is stored in a key-value pair. Thus, if the dictionary is sorted by key, then the value can be accessed systematically. However, it is sometimes observed that the value field in a dictionary needs to be sorted in order to make the list more systematic and organized. Therefore, if you know how to sort a dictionary by value in Python, it will make it easy for you to manipulate the data in it.