Fundamentals of Python Programming
Bytes to String in Python
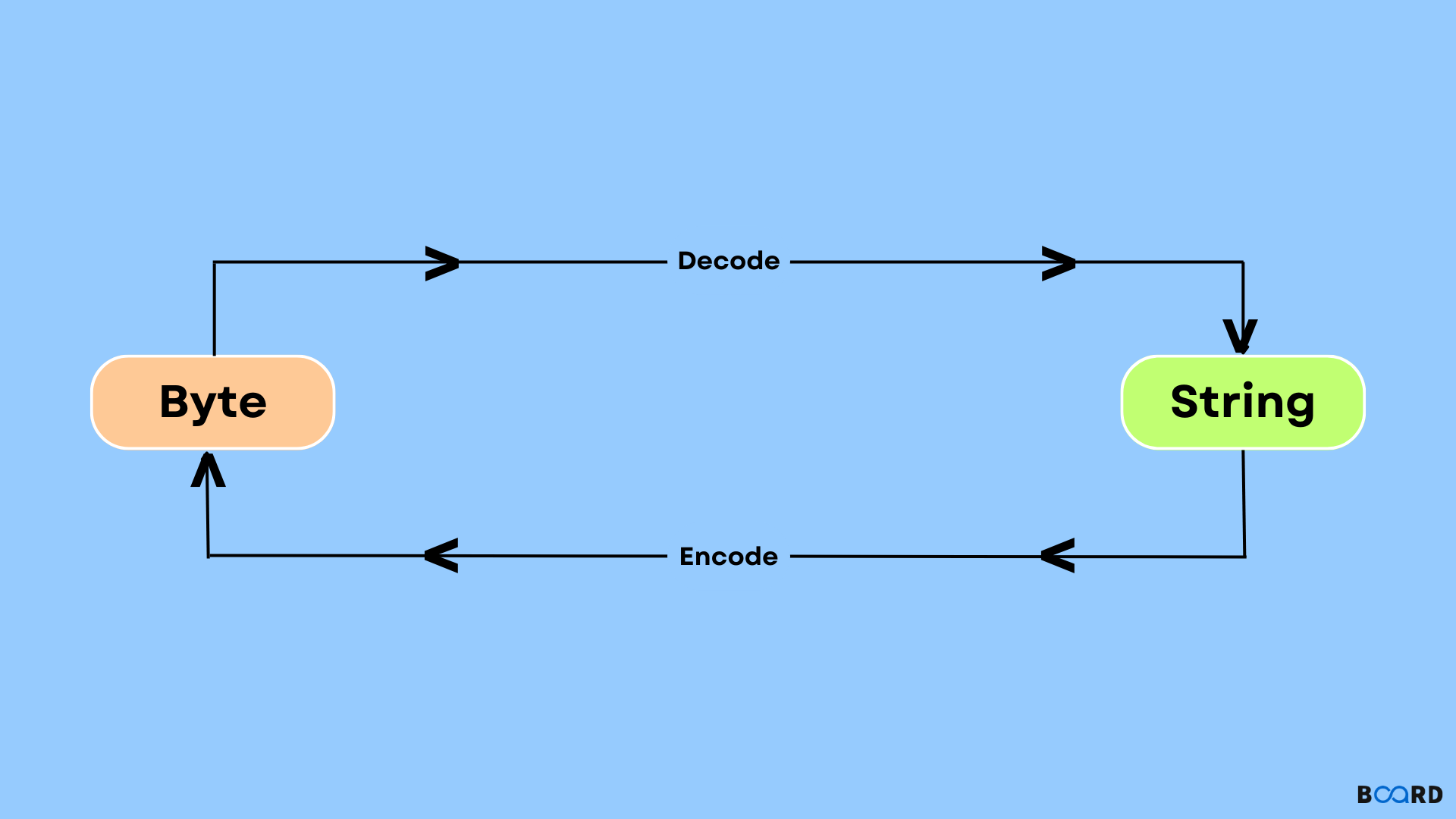
Introduction
Every time we save something in Python, it will be stored as a byte. Strings are forms that can be read by humans, whereas bytes cannot. Any string that is stored will first be encoded into bytes using multiple techniques, such as ASCII and UTF-8, before being stored as a string.
For example, ‘I am a infinity’.encode (‘ASCII’)
In the example above, we use the ASCII encode method to translate the string into bytes. And we will get results like "I am an infinity" when we print it in Python. In this case, we can observe that the string only contains the letter b. In this case, print() decodes the bytes to forms that can be read by humans so that we can read the strings even if we aren't really able to read the bytes.
In reality, however, the output of running this string to display each character in the bytes strings will look like this:
The two strings were made. One string is easy to understand. Bytes make up the other string. The results that we receive while printing both string kinds are seen below. The findings below demonstrate that one string is a string type and another is a bytes type.
To determine the difference between the two strings, we will now print each character from each. The first thing we'll do is use a for loop to print a standard string, called str1.
We will now examine various techniques for converting bytes to strings.
# 1 - Using the map() function
In this approach, the bytes will be transformed into a string format using the map () function. The little programme below will explain the idea.
#2 - Using decode () function
A decode () function is an additional approach. The encode () function's opposite behavior is mimicked by the decode () function.
#3 - Using the codecs.decode () function
We'll use the codecs.decode () function in this approach. The binary string is converted into normal forms using this function. Let's now examine the functionality of this function.
#4 - Using the str () function
The str () function may be used to transform the bytes to regular strings as well. The simple software needed to comprehend this approach is provided below.
Conclusion
As Python programmers, we frequently encounter errors related to bytes when working on various languages. In order to prevent issues while using any methods connected to strings, we are attempting to provide some ways for converting bytes to strings in this article. We have covered every concept needed to translate bytes into strings in this tutorial. You can make a decision based on what is required by your program.