Fundamentals of Python Programming
List Index Out of Range Error in Python
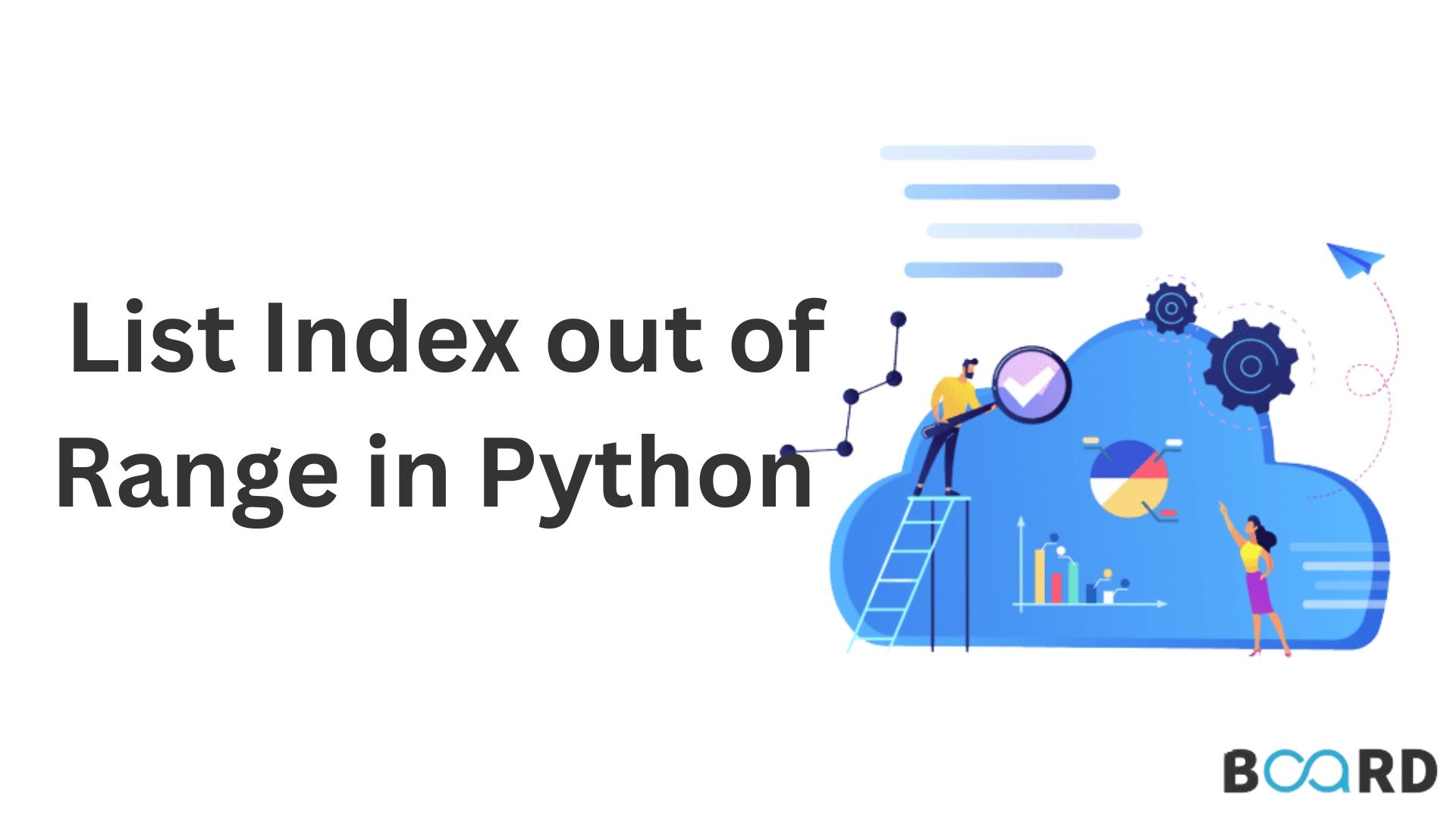
Overview
In this article, we shall learn about what the Python error “List Index Out Of Range Error” is and how to resolve it.
Scope
- Cause of the error.
- Fixing the error by using the range() method.
- Fixing the error by using the index() method.
- Fixing the error by using the “in” operator with range.
What causes “list index out of range”?
“list index out of range” is an error that frequently arises in Python, usually while working with lists. The error occurs when we try to access an index in a list that is not in the range of its size. Following are some prominent examples of this problem occurring:
Code
Output
Code
Output
Using range()
The method “range” returns a specific range with respect to the size of the given list. It returns a sequence of numbers between the defined range.
Syntax
Parameters
start
An obligatory number denotes the start of the sequence. The default value is zero.
stop
A number that denotes the end of the sequence.
step
An obligatory integer is required when increasing the iterator increment by more than 1.
Example
Output
Using index()
index() function finds and returns the index of the first occurrence of the given element in the given array. This index value of the last item of the list we use by adding 1 to it. The resulting value is the exact value of length.
Output
Using in
Output