Fundamentals of Python Programming
Not Equal Operator in Python
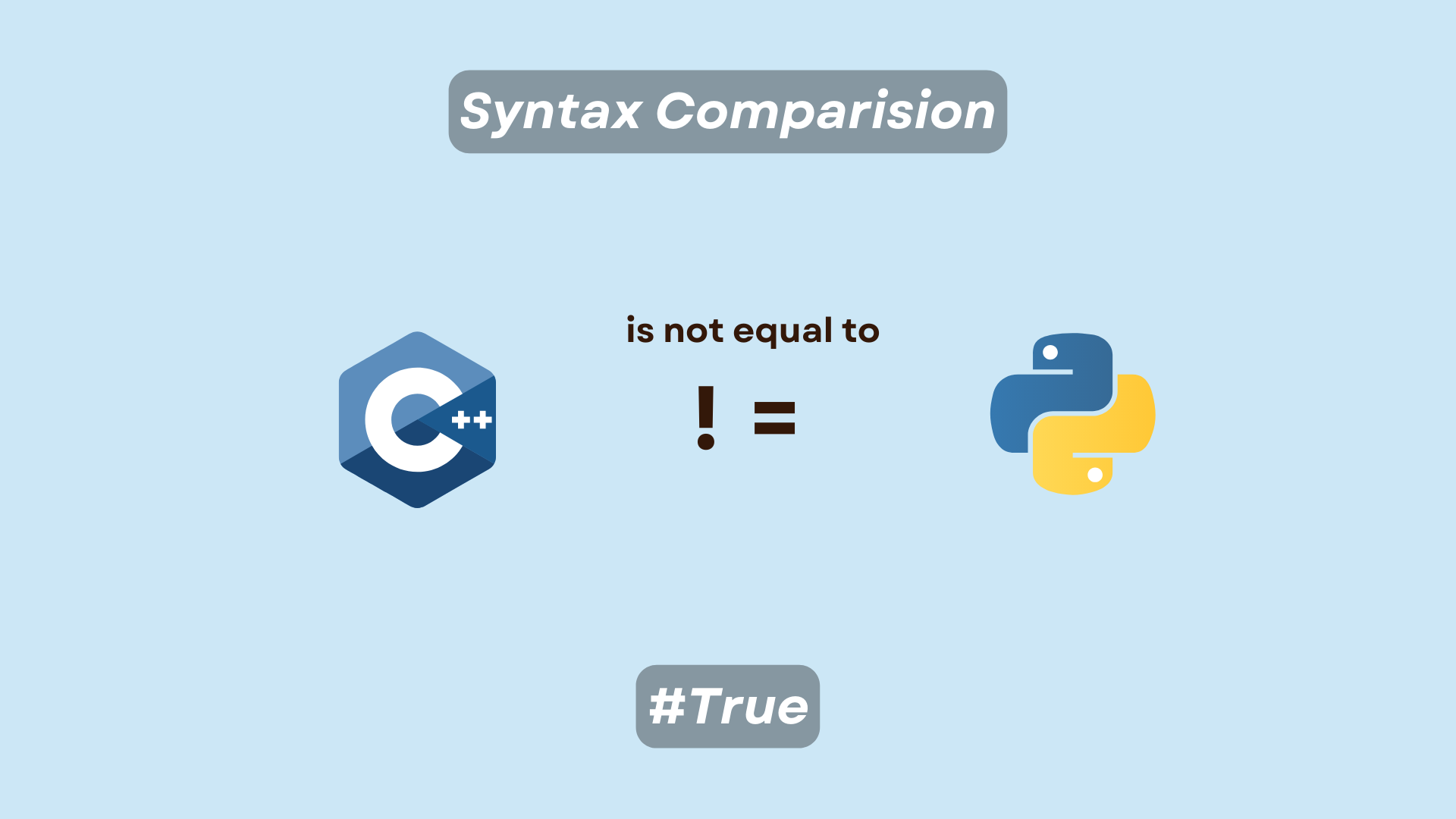
Introduction
Python is known as a very dynamic programming language and is typically thought of as a tightly typed language. By understanding the importance of the not equal operator, this sentence may be clarified. If the two operand values on either side of the not equal operator are not equal, the operator returns true; otherwise, it returns false.
In the not equal operator, a true value is returned if two variables have different types but the same value individually. Python is a particularly dynamic language since few other programming languages can describe it as true if the variable type is of a different kind. Not all equality operators in Python can be classified as comparison operators.
An easy illustration of the not equal operator
To demonstrate the not equal operator, let's look at two instances. Here is an example of using the not equal operator with the same data type but distinct values:
Here is an illustration of a not equal operator with data of a different type but the same values.
Use of the Not Equal Operator in an IF Statement
An if-statement in Python is a statement that evaluates when the entry-level condition is true after checking it. Here is a simple example of how to use the if statement with the not equal to operator: -
The if statement and the not equal to operator (!=) are both used in this case.
How to use the equal to (==) operator with a while loop
While-loops in Python run over blocks of code depending on whether a condition is true or false. Take the following example of outputting odd numbers using the while loop and equals operator:
Here, the if statement and equal to (==) are both used.
Making use of the not equal operator to find even numbers
While loops in Python can also be combined with the not equal to operator. Take the following example of outputting even integers using a while loop and the not equal to operator:
Here, the if statement is used coupled with the not equal to operator (!=).
How to use the "not equal" operator in Python when using a custom object
Users or developers can construct their own unique implementations using custom objects. This gives the developers the ability to alter the actual result from what is often expected. Take the following custom object as an example, which makes use of the not equal to operator: –
Example:
Tips for Using the "Not Equal" Operator
- In formatted strings, you can use the not equal to operator.
- This functionality, which is a part of Python 3.6, is relatively new.
- Because certain fonts or interpreters alter syntax from!= to, the developer should make sure that the syntax is!= and not.