Fundamentals of Python Programming
String formatting in Python
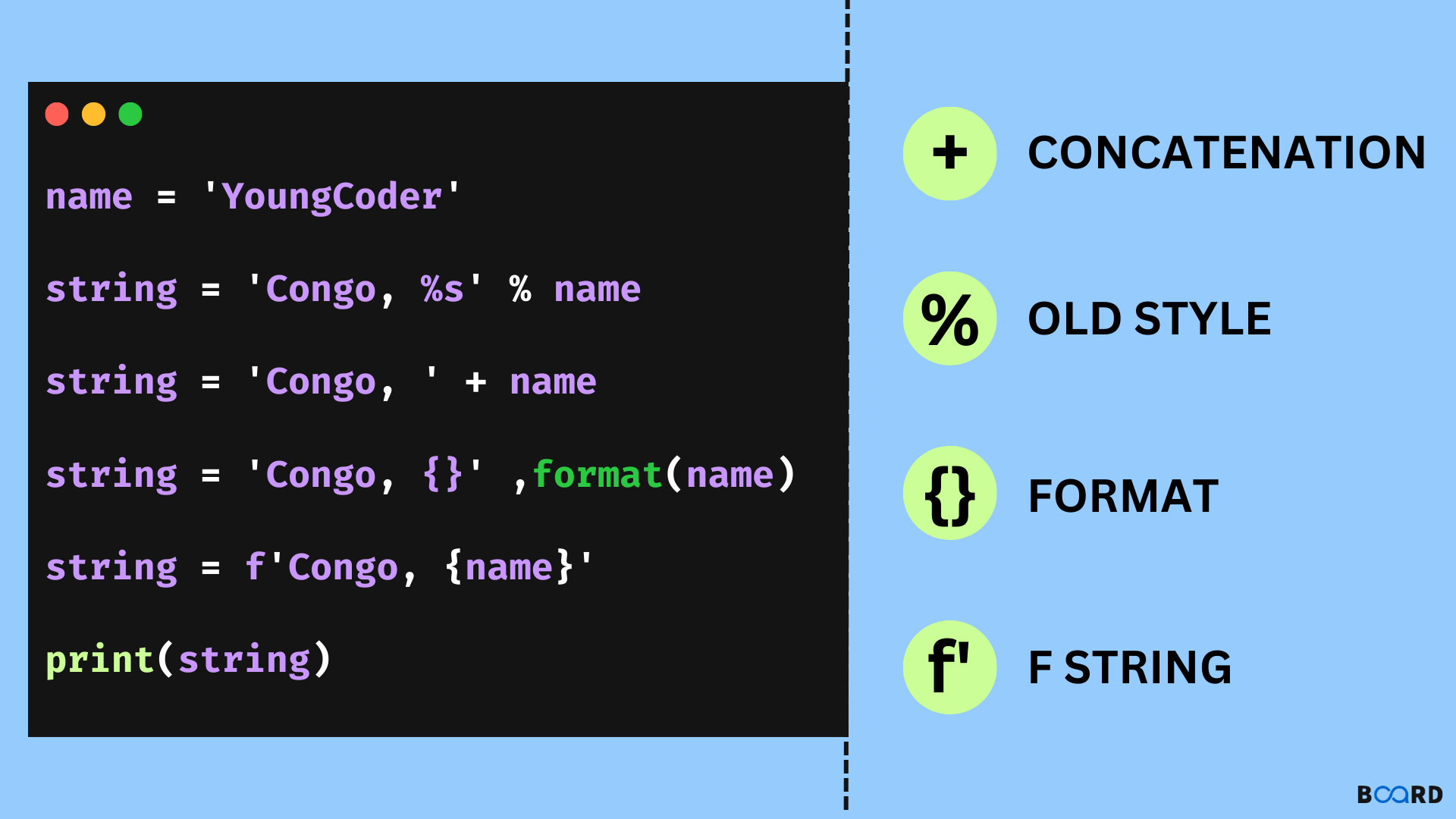
Concatenation's Formatting Rules
print("Hello"+name)
The easiest approach to learn is this one because it is the most basic. Additionally, it's how I like to teach a beginner programmer.
It is simple to understand even without any prior coding experience by using the portions in the literal order and the "+" operators.
CODE:
OUTPUT:
In order to construct a formatted string, the aforementioned example shows how to combine variables using the addition operator. Here, it's important to bear in mind that we must convert each variable to a string. Name is already a string in this case, and year is an integer.
Formatting a String the Conventional Way
'Hello%s'% name in print
When Python2 was still a new and developing language, this strategy was applied more frequently. It's a method that seasoned programmers with a foundation in C programming will find simple to learn.
As you can see in the code below, we are defining the placeholder in this case together with the arguments at the conclusion of the statement and the type of data that is supplied. Similar to the C language, this (and other languages too).
Code:
Output:
The variables that we wish to format into the strings are defined in the aforementioned example. The string is then created with the variable placeholders.
Keep in mind that the types of the placeholders and the related variables should match. Using the % sign at the conclusion of the statement, we can connect the real variables to the placeholders.
Using Format, format a String as follows: ".format ()
Given how much easier it makes things for you, many Python programmers view this technique as a breath of fresh air.
Early Python3 contained its introduction. In essence, the new syntax got rid of the '%' symbols and added a string method called.format() that takes positional parameters for substitutions specified in curly braces.
Although this can feel a little cumbersome at times, we can also specify keyword arguments here. The str.format method allows you to set accuracy, rounding, and zero padding, among other things. We shall simply concentrate on the fundamental usage for the purposes of this paper.
Code:
Output:
In the example above, we create the variables that will be used to format the string. Then, in the simple form, we can substitute for the required variables. The variables are then specified as an ordered collection of parameters and the string is formatted using the.format() method.
In the placeholders in the second section of the example, ordered parameter indices are used.
The final section of the example also demonstrates how to use named parameters and a dictionary in the.format function to format a string.
F String Formatting Instructions: f'hello name
F strings, also known as format strings, are the newest approach that Python3 now offers, and as such, they are gaining popularity quickly.
By prefixing the string with the letter "f," F strings reduce the verbosity of the.format() method and offer a quicker alternative. Of the two strategies, this one appears to be the more effective:
1) The concatenation's literal order
2) The.format() method's modularity
These benefits have led to an increase in its use among developers. I believe it also has the advantage of being simpler for kids to learn and simpler to use if you're still learning how to code.
Code:
Output:
The aforementioned example is comparable to the.format() method, as you may have seen. In this case, we specify the variables within the placeholders while still using the as a placeholder in the strings. When the lowercase letter f appears at the start of the string, this function is activated. Additionally, the approach works with various data types like dictionaries.
Do we really need to understand and employ all of these string formatting techniques?
All of this information is crucial for studying Python. There are certain older forums, Stack Overflow postings, and several older Machine Learning frameworks that still utilise these older methods of string formatting, even though you won't use them frequently.
Because of this, it's beneficial to be familiar with them so you can understand what's happening and perhaps debug a problem. You can format your Python strings using any of the other three techniques we covered here. Simply choose the one that fits the situation and even your current mood best!
When working with short, straightforward phrases, the Plus is helpful. Additionally, you can use it to create debugging states as you work on a straightforward project.
For instance, the + operator could be used to create the following simple number guessing game:
The debug statements used in the aforementioned code will be removed from your finished product. (You wouldn't want to reveal the secret number, would you?) Therefore, while writing your code, you can use the concatenation strategy in your prints.
You might prefer the.format() or f string methods for adding logging statements because they'll make your statements more modular. Additionally, it will be simpler to define formatting and precision for the data type itself.
Do any further Python string formatting methods exist?
Yes! One such method is the use of template strings, which is offered as a class in the Python standard library's string module. The.substitute() method is used to replace placeholders, and it is imported as a module.
Having said that, I have never had to utilize this in my previous coding experiences and I only became aware of it when conducting research for this blog.
Conclusion
A Python coder should be familiar with Python string formatting as it is a useful daily tool. String formatting appears to be consistent across all coding languages. It is also a crucial factor in our use of scripting languages. The f string method, along with.format() and concatenation, appears to be the most durable of these various approaches to formatting strings. As most legacy libraries start to support Python3, the Python2 syntax may start to disappear.