Fundamentals of Python Programming
Python print() Function
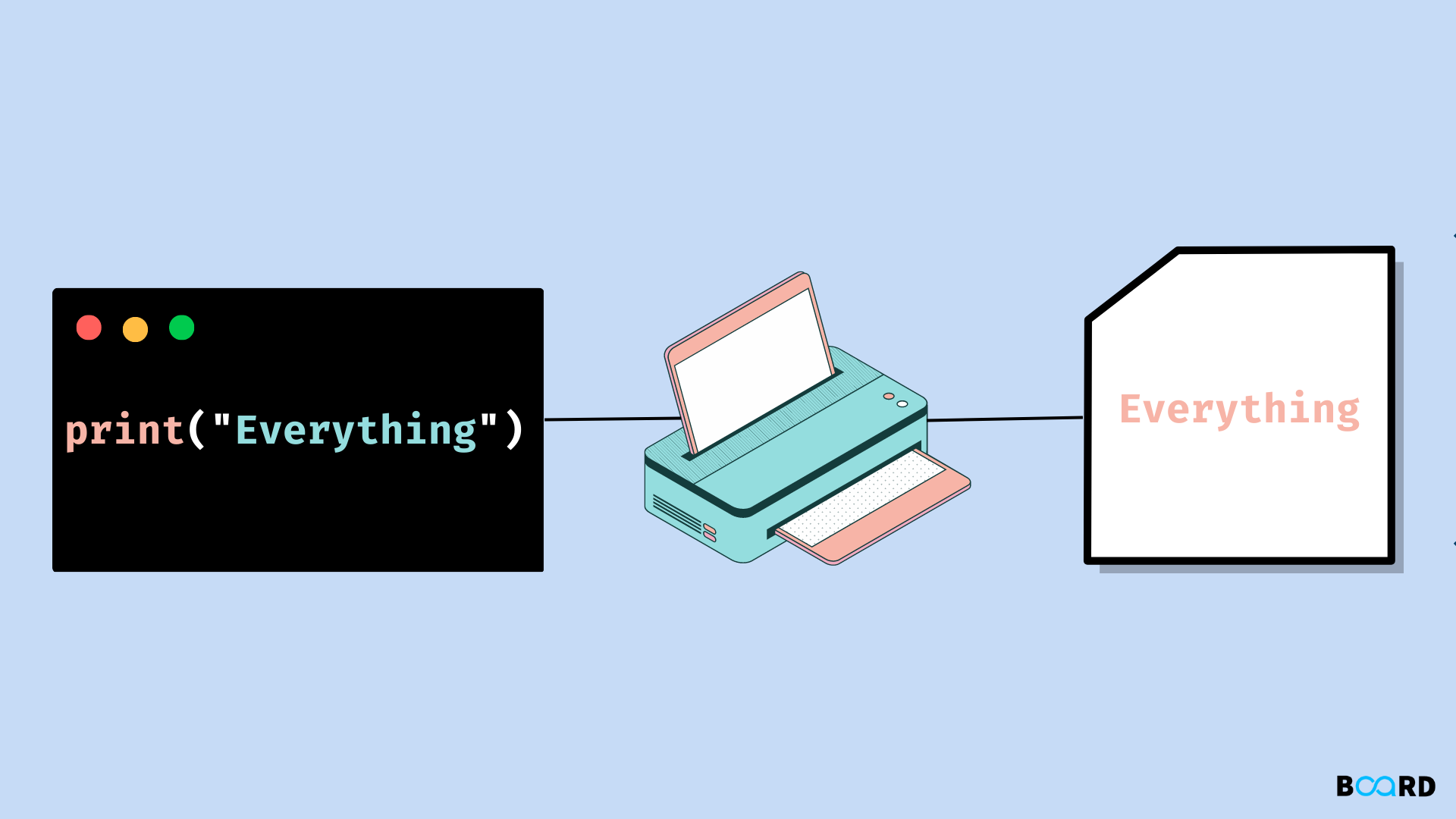
There are 4 ways to use print function in python:
Example 1: Print() with sep Parameter
Suppose you have a list of strings “Hello”, “World”, “I”, “Love”, “Python” . So, you have a comma separated list of strings and you want to print all these strings so let us see what output we get
Output:
You will see that output is in a same line but you can specify that what should be the separator between these words so maybe you can have a separator which is called a comma so
Output:
You will see the list is now separated with a comma and suppose you want to have a separator backslash n
Output:
You will see all the words come in a new line or you can have a backslash double n so all the words are now separated with two backslashes n
Output:
So you will find one empty line between each word right so this is the use of a separator parameter this is a named parameter and you can specify any separator between these words maybe it can be a plus so you will see Hello Plus world plus I plus love plus Python so this is one use case of using this ”sep” keyboard.
Output:
Example 2: Print() with end Parameter
Next way is the “end” keyword, so suppose A is 10 and B is 20 and you want to print A and B so the output is in a different line
Output
but if you specify that the end of this line should be with the space you will see the output is in the same line
Output:
or if the end is with a comma you will see the numbers are separated by a comma.
Output:
Example 3: Print() with format method
Now we are going to learn about the format method. Suppose I have variable a which is equal to Indians and I have variable b which is equal to mangoes and we want to print into some particular format so here we will specify the indices of the arguments which we will be giving to our format function so consider the below code
Output:
Output:
So here {0} love {1} and we call dot format method and then we will supply a word parameter side a and b so you will see that the zeroth argument is replaced by ’a’ which is Indians and the first argument is replaced by ‘b’ which is mangoes. This method comes very handy when you are printing some data or you want to print things in a similar format now consider ‘c’ is Russians and ‘d’ is Pizza and you can just call this method and you have to just change these values and you will see Russians love Pizza.
Example 4: Print() with modulo operator
This print function behaves as a template and one more thing about the print function is that it also supports C language type printing what it means is you can have a print percentage let's say it is again 10 B is 20 and you want to print numbers right so suppose you entered a number ‘a’ which is %d and B it so you entered these numbers and you will give a comma and so here you will give a %d and in the bracket you will specify what does these numbers mean and %d and here you will give a and b see you entered 10 and 20 this is also a template kind of thing where the first %d will be replaced by the first integer and the second %d will be replaced by a second integer.
Output: