Fundamentals of Python Programming
Exit Command in Python
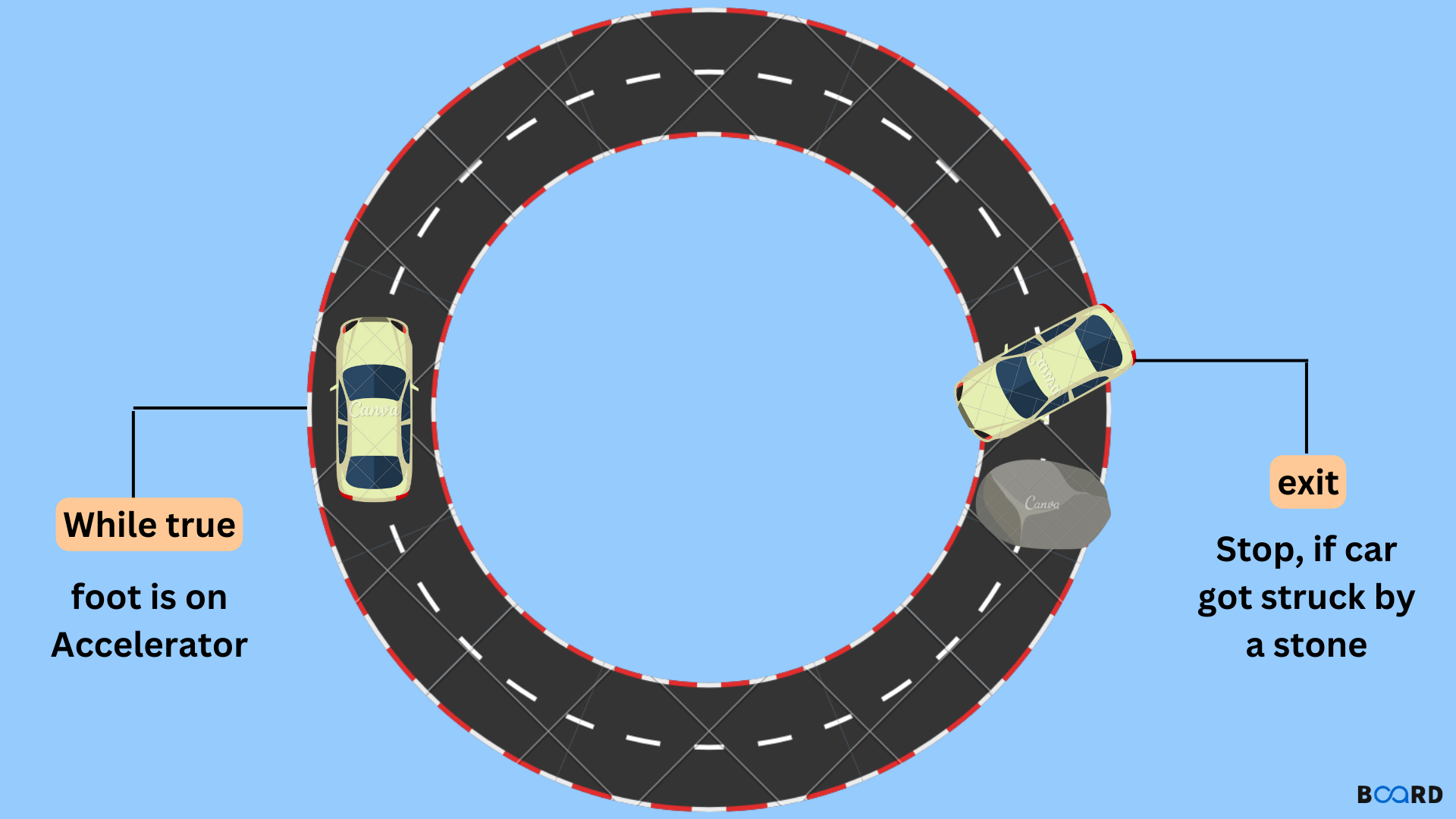
Introduction
Python is a very active programming language. It is one of the most popular programming languages due to its versatility.
When dealing with Python, we frequently need to exit the program before the interpreter. The application will exit automatically if the Python Exit function is used. We don't just have the Python Exit method in Python. Other methods for terminating a program in Python include quit(), sys. exit (), and os. exit ( ).
Simple code execution allows the interpreter to complete the script and then leave the program.
When we run a Python program, the code is contained in a single Python file from start to finish. When it reaches the end of the file, the software terminates. However, we can explicitly terminate code using Python's built-in exit functions.
This post will teach us four distinct approaches for creating Python Exit Functions. For a better understanding, there will be an example code block with output.
Python exit () Function
In Python, the Exit function is exclusively usable with the site.py module. However, we do not need to import the sys module because it is already included in the Python library.
This is why the exit() function cannot be used in a production environment. The production code is the code that the audience will use in a real-world setting.
Only the interpreter is supported by the exit () function.
It outputs a message when used with the print statement since it utilises the System Exit Exception class and no Stack Traceback.
Example
Output
Python quit( ) Function
Quit() and exit() are considered synonym functions since they were designed to make Python more user-friendly.
Quit() is also used in the site.py module, although it does not work in production.
Because of the SystemExit exception, the quit function prints a message.
Example
Output
Python sys.exit( ) Function
This function, which is part of Python's sys module, works similarly to the previous ones. It is not, however, dependent on the site module.
Unlike the other functions, sys.exit() can be used in both real-world and production programs because it is a sys module function that is available everywhere.
When working with large files, we use this function to control the terminal.
In this sys.exit(), we can give an optional argument in the form of an integer or any other type of object.
Example
Output
Python os. _exit( ) Function
os. exit() is a function in the Python os module. We can observe it working in Python's production environment.
os. exit() immediately quits the process with the supplied status.
This function does not use stdio buffers, cleanup routines, handlers, or flushing, among other things.
This line is not a good way to leave the Python program because it is never followed by a return statement.
This function is primarily intended for usage in terminating a running child process.
Example
Output
Conclusion
When we don't want the interpreter to reach the end of the program and wish to stop before it does, we can use the Python Exit routines. The most recommended technique in the following Python Exit functions is logically sys. exit(). The functions exit() and quit() are unsuitable for production use, while os. exit only handles unusual instances for immediate exits. Finally, the decision is yours; so make your choice based on your tastes. I hope the examples help you understand the various Python Exit methods.