Fundamentals of Python Programming
Learn Different Methods to convert a List into a Tuple in Python
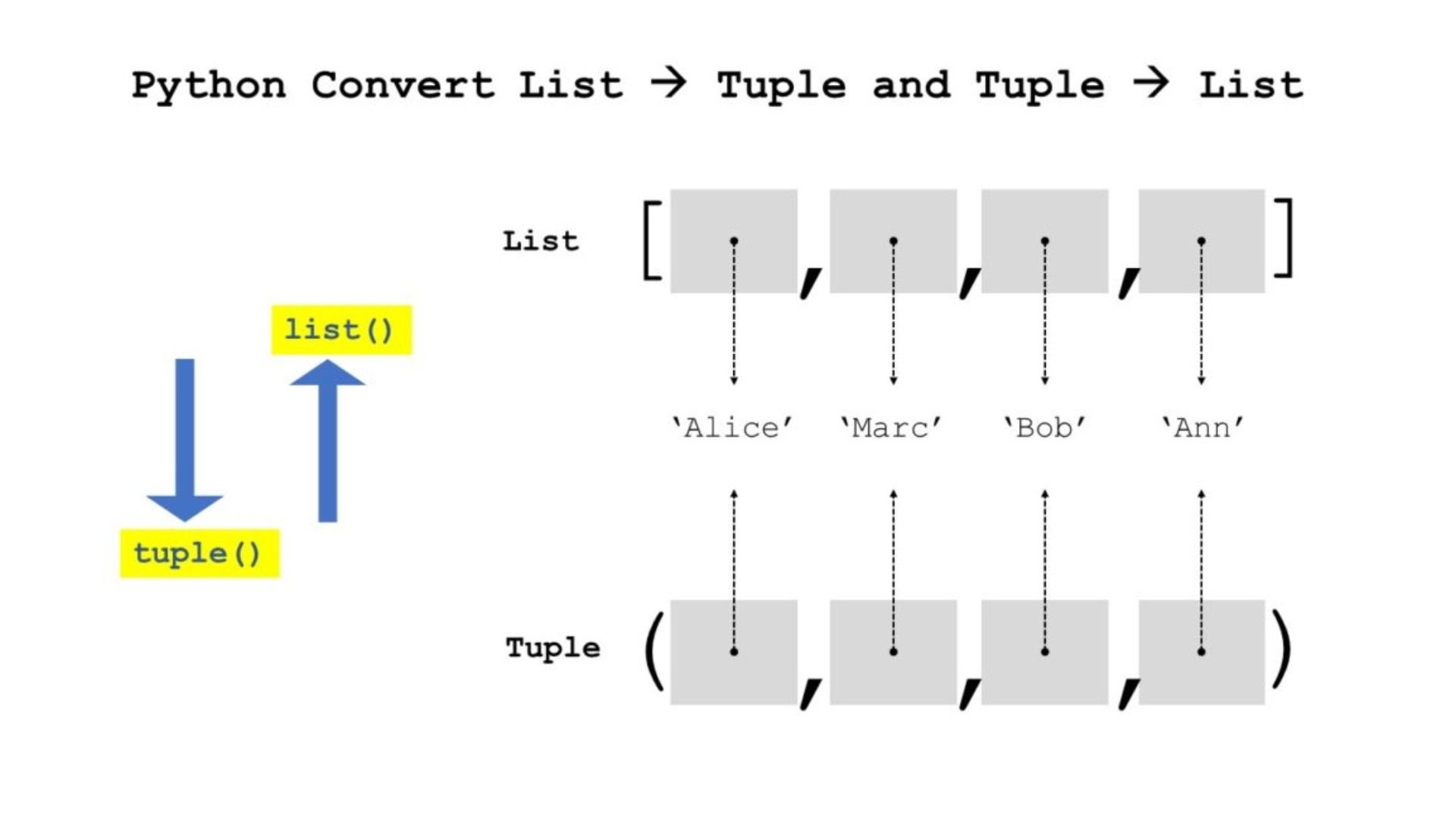
In Python, Tuple and Lists belong to the class of data structure. The list in Python is dynamic on the other hand the tuple in Python is static. Let us first understand each of them and after it, we will see how to convert a list into a tuple.
List
In Python, lists are a data structure that is used widely. It is a mutable data structure which means that the List can be changed after it is created. In the list, elements are in an ordered sequence. Below is the following example of a list.
If we execute this code, the Output will be:
Tuple
In Python, Tuple can be defined as a collection of python objects which is separated by commas and are ordered and also immutable that is it can not be changed after it is created. Tuples are similar to lists, they are sequences. A tuple is different from lists by tuple can not be changed and lists on the other hand can be changed also tuples are enclosed in parentheses, and lists are enclosed in square braces.
Let us look at the example below.
After executing the above code the output will be:
Now let us see how can we convert a list into a tuple.
Method 1: Using the tuple() built-in function
In the tuple() function, an iterator can be passed which we then convert to a tuple object. If we need to convert a list to a tuple, we have to pass this whole list to the tuple() function as an argument, and in return, it will give the tuple data type.
Code
Output
Method 2: Using a loop
This method is like Method 1, only the difference is that we do not have to send the whole list as an argument, we have to traverse at each element and then we have to send that element to the tuple() function as an argument.
Code
Output
Method 3: Using unpack list inside the parenthesis(*list, )
This method is faster when compared to other methods in Python but it is not efficient also it is not easy to understand. In this method inside the parentheses, the list gets unpacked and the elements are unpacked in the tuple literal, which is generated by a single comma (,).
Code
Output