Fundamentals of Python Programming
Python numpy.linspace()
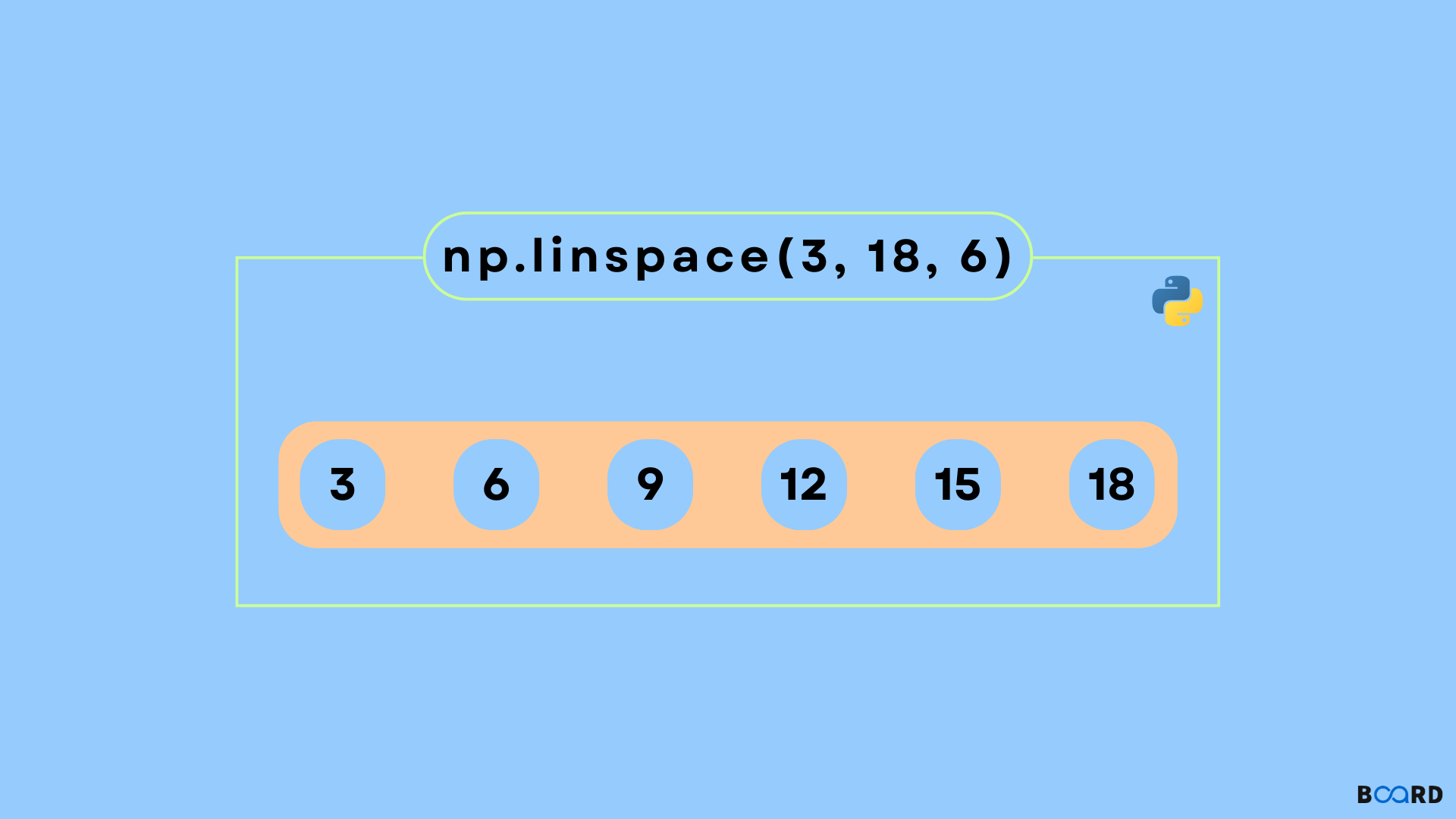
Introduction
In this article, we shall learn about the method “linspace” from the module “numpy” in Python. It is a Python method that returns evenly-spaced numbers over a given interval. linspace() is quite similar to the Python arange() function. But a difference in between the two is that linspace() uses a sample number instead of a step.
Scope
In this article we shall learn about:
- Syntax of using the linspace() method in Python.
- The parameters that the linspace function takes.
- The return value of the linspace method.
- A fine example of using the linspace() method along with code implementation.
Syntax
Parameters
start
It is an optional parameter and denotes the start of the range of the interval. Its default value is zero.
stop
It represents the end of the interval range.
restep
If set to True, returns (samples, step). The default value is False.
num
It’s an optional parameter the value of which must be a number dictating the number of samples to be generated.
dtype
It’s the type of output array.
Return
Implementation and examples
Example 1
Output
Example 2
Output
Example 3
Output