Fundamentals of Python Programming
Converting String to Dictionary in Python
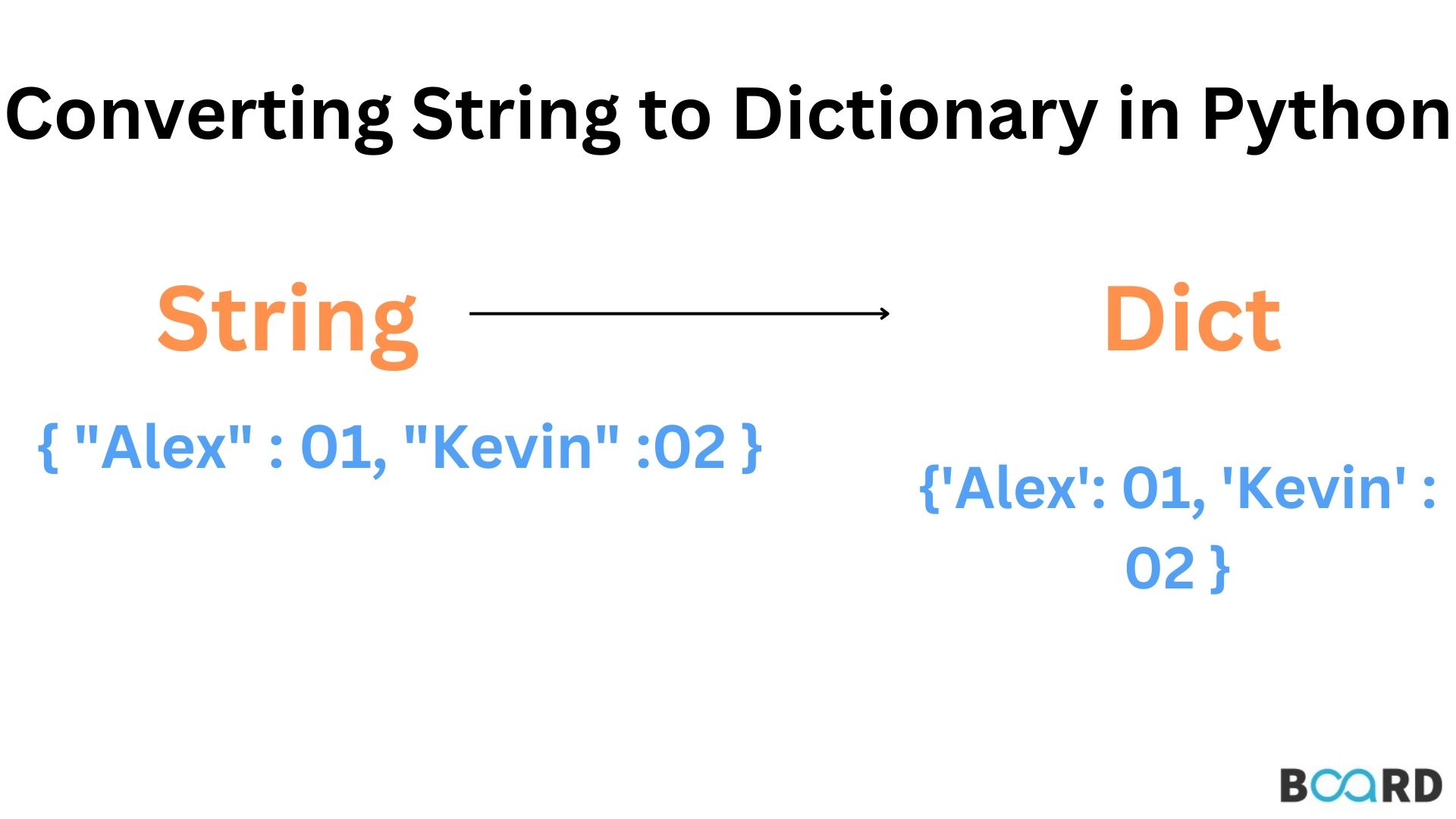
Introduction
A reliable data structure for working with key-value pairs is the Python dictionary. However, json string is employed for sending data back and forth between the client and server. When data is accessible on the client side, we must translate the JSON string into a dictionary because it is a standard for data sharing. Given a dictionary's string representation. How can it be made into a dictionary?
Method 1: eval()
The built-in eval() method accepts a string parameter and parses it to make it look like a code expression before evaluating it. The return value is a standard Python dictionary if the string provides a textual representation of a dictionary. By using the eval() method, you may quickly turn a text into a Python dictionary.
Method 2: ast.literal_eval()
A string literal, such as a dictionary represented by a string, or an expression can be securely evaluated using the ast.literal eval() function. It addresses many of the security issues with the eval() function and is also appropriate for strings that may originate from unreliable sources. By using the expression ast.literal eval, you may transform a dictionary's string representation
Method 3: json.loads() & s.replace()
Use the json.loads() function on a string to transform a dict's representation into a dict. First, use the expression s.replace( to convert all single quote characters to escaped double quote characters "'", """) to make it compatible with Python's JSON module. The command "json.loads(s.replace("'", "" ")) creates a dictionary from the string s.
Unfortunately, this approach is subpar since it will not work for dictionary representations when the keys or values have trailing commas or single quotes. It's not the easiest one either, which is why technique 1's broad strategy is chosen.
Method 4: Iterative Approach
A string may also be turned into a dictionary by breaking it up into a series of (key, value) pairs. After some cleanup, you may add every (key, value) pair to an originally empty dictionary. Here is a simple Python implementation: