Fundamentals of Python Programming
What are defaultdict in Python?
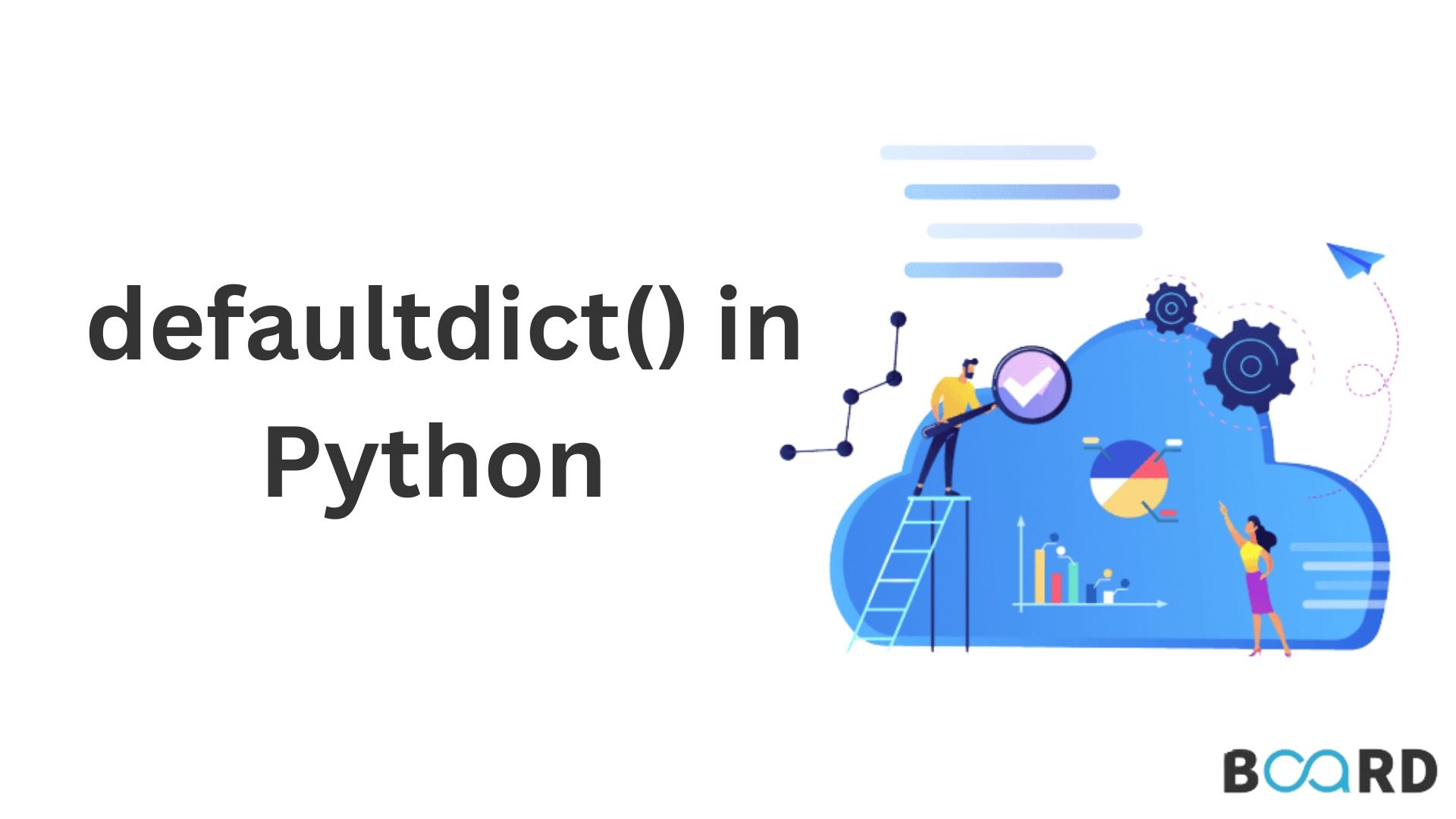
Introduction
The Python dictionary, dict, has key-value pairs of any data type together with terms and their definitions. Another split of the built-in dict class is the defaultdict.
Dictionaries are a practical way to save information for later name-based retrieval. Unique, immutable objects—typically strings—must be used as keys. A dictionary's values could be anything. Values are frequently of straightforward types like strings and integers for many applications. When dictionary values are collections, the situation becomes more interesting. In this situation, initializing the value (in this case, an empty list or dict) is required before using a specific key. Despite the fact that performing these tasks manually is fairly simple, the defaultdict type automates and streamlines them.
How defaultdicts are different from Standard dicts
The functionality of a defaultdict is identical to that of a standard dict, except it is initialized using a function (the "default factory") that accepts no parameters and supplies the default value for any keys that aren't there. A defaultdict never causes a KeyError to occur. The default factory's value is returned for any key that doesn't have a value.
Make sure to supply defaultdict with the function object (). Never invoke the function; for example, use defaultdict(func) rather than defaultdict(func()). In the example that follows, counting is done with a defaultdict. Int is the default factory, and its default value is zero. The value, where the key is the food, is increased by one for each item on the list. We don't need to confirm that the food is already a key because it will accept zero as the default value.
The list of states and cities is the first step in the following example. The state abbreviations will serve as the dictionary's keys, while listings of each state's cities will serve as the dictionary's values. Utilizing a defaultdict with a default list factory, we create this dictionary of lists. For each new key, a fresh list is generated.
In conclusion, use a defaultdict anytime you require a dictionary and each element's value has to begin with a default value.
FAQs for defaultdict
Can a defaultdict and a dict be equal?
-> Yes. By generating one of each with the same things and adding a condition that evaluates whether they are equal, which evaluates to true, one may determine if defaultdict and dict are equivalent if they both hold the exact same items. Also, defaultdict functions just like a dictionary if no arguments are supplied to it.
Without using defaultdict, can we prevent receiving a KeyError in a dict?
-> Yes. By initializing mutable collections before their first use, KeyErrors can be effectively avoided when used as dictionary values. It is preferable to use defaultdict because it simplifies and automates the process.