Fundamentals of Python Programming
Python reduce() function
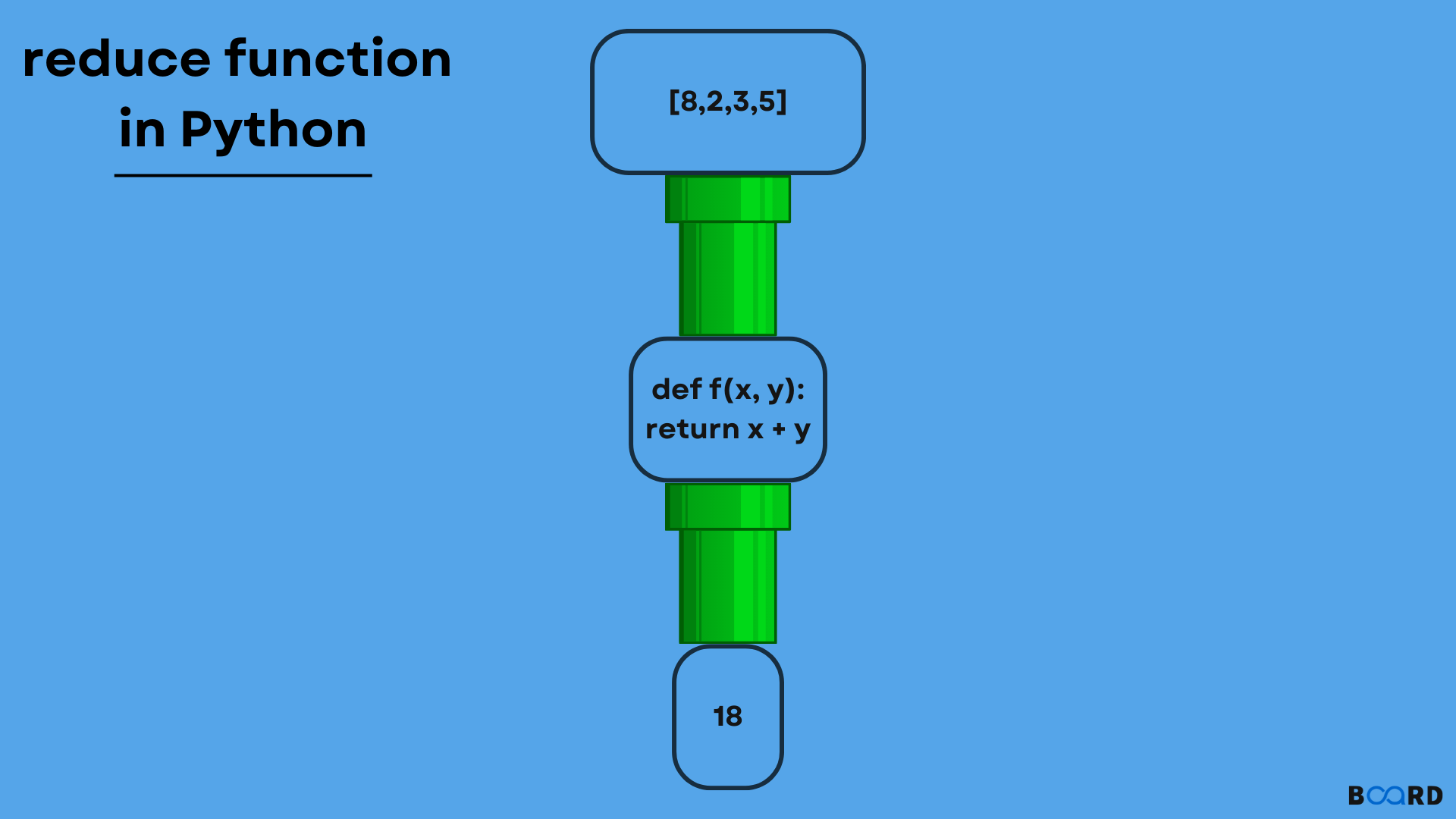
Introduction
A function and an iterable (such as a list, tuple, dictionary, etc.) are sent to Python's reduce() method to perform functional computing. The function returns the result after the calculation is complete (applying the function on the iterable).
The functools module in Python defines the reduce() method, which only delivers a single value as output after reducing the entire iterable to a single integer, string, or boolean. It does not return any iterators or multiple values. Let us understand what a reduce function in Python is.
reduce() Function: What is It?
Python's reduce() function applies a pre-defined function to each element of an iterable (such as a list, tuple, dictionary, etc.) and generates a single-valued result. Applying the reduce function to the iterable passed as an argument yields this single-valued output, which only returns a single integer, string, or boolean.
Without explicitly programming For loops, processing iterables using reduce() is very convenient. Reduce() is a built-in function written in the C programming language, making it much more optimized and faster. It is similar to a for loop in Python.
The functools module, which must be imported before calling the function in our program, contains the reduce() function in Python. This single value output denotes that only one integer, string, or boolean is returned due to applying the reduce function to the iterable. Let us look at the syntax of reduce function in Python.
Syntax
These two parameters in reduce function are
- Reduce() takes a function as its first argument. The result will be computed cumulatively, applying this function to each element in an iterable.
- The second argument can be repeated. Python objects that can be iterated over or looped over are known as iterables. Examples include lists, tuples, sets, dictionaries, generators, iterators, etc.
Let us look at the working of reduce function in Python.
Working of Reduce Function in Python
The reduce() function in the functools module of Python only returns one value and doesn't return any other values. Steps for using Python's reduce function are mentioned below:
- The iterable's first two elements are subject to the function passed as an argument.
- The function is then applied to both the most recent result that was generated and the subsequent element in the iterable.
- The iterable is processed until the end of this process.
- Applying the reduce function to the iterable causes the single value to be returned.
Let us look at the example of reduce function in Python.
Examples
We will discuss different examples by using reduce() function in Python.
Lamba Function with Reduce Function
In the given example, a lambda function has been defined to use the reduce function to iteratively calculate the multiplication of two numbers on the passed list.
Output
Predefined Function with Reduce Function
Now, a pre-defined function called sum is used as an argument in the reduce() function, that is passed a list of numbers and returns the sum of any two numbers that are passed to it.
Output
Operator Function with Reduce Function
Operator functions and the reduce function are sometimes implemented together in Python. Operator functions are the fundamental pre-defined logical, bitwise, and mathematical operations bundled into a single operator module in Python. Let's investigate the relationship between operator functions and the reduce function.
Output
Let us understand how can we use reduce function in Python with three parameters.
reduce() Function with Three Parameters
In Python 3, the reduce function, or reduce(), supports both two and three parameters. To put it simply, reduce(), if it is present, places the third parameter before the value of the second one. The third argument then acts as the default one if the second argument is an empty sequence.
Code
Output
Conclusion
Python's Reduce function enables us to apply reduction operations to iterables using lambda and callable functions. A function called reduce() reduces the elements of an iterable to a single cumulative value. The reduce function in Python solves various straightforward issues, including adding and multiplying iterables of numbers.
Even though many Python functions, such as sum(), any(), and min(), have largely replaced the Reduce function, it is still a very common technique in functional programming.