Fundamentals of Python Programming
Python 2D Array
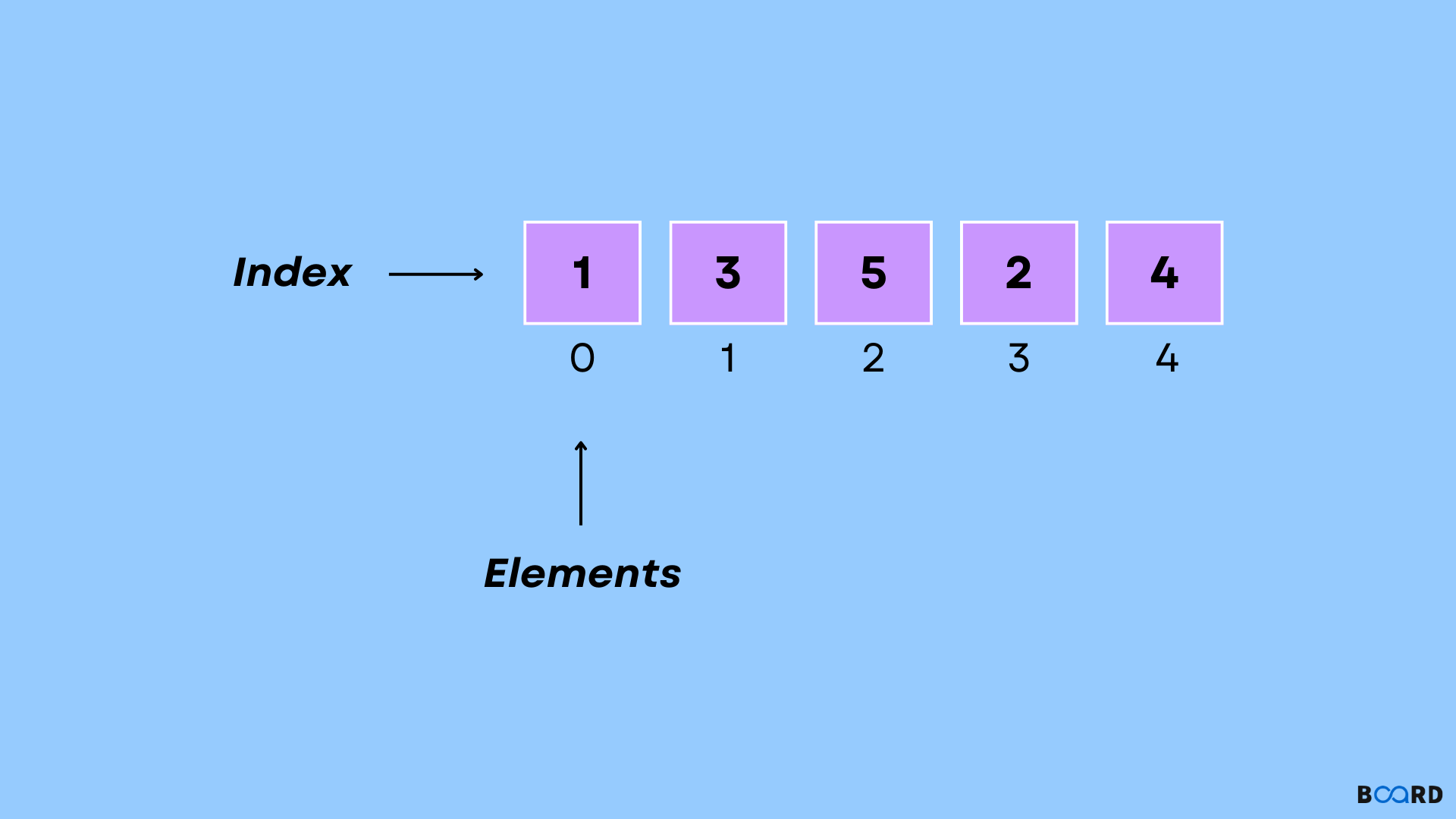
Introduction
In Python, there are multiple ways in which a user can create 2d arrays or lists. But before implementing these ways we need to understand the differences between these ways because it can create complications that can turn out to be hard to trace.
Initializing 1D Array
Let us see some common ways of initializing a 1D array with 0s filled.
Code
Output
Let us create a list using list comprehension.
Code
Output
Creating a 2D array
Method 1: Naive approach
Code
Output
Explanation
In the code above, we multiply the array with the columns ©, now this will result in a 1D list, whose size will be equal to the number of columns. After it, we again multiply the array with the rows ®, again this will result in the construction of a 2D list.
Now sometimes we need to be careful while using this method as it can cause unexpected behaviours. Each row in this 2D array will reference the same column. This means if we try to update only a single element of the array, it will update the same column in our array.
Code
Output
Creating a 2D array using list comprehension
Code
Output
Explanation
In the above code, we are using the list comprehension technique, in this technique we use a loop for a list and inside a list, hence creating a 2D list.
Creating a 2D array using empty list
Code
Output
Explanation
In the code above, we are trying to append zeros as elements for some columns times and after it we are trying to append this 1D list to an empty row list, hence creating a 2D list.