Fundamentals of Python Programming
Converting String to Float in Python
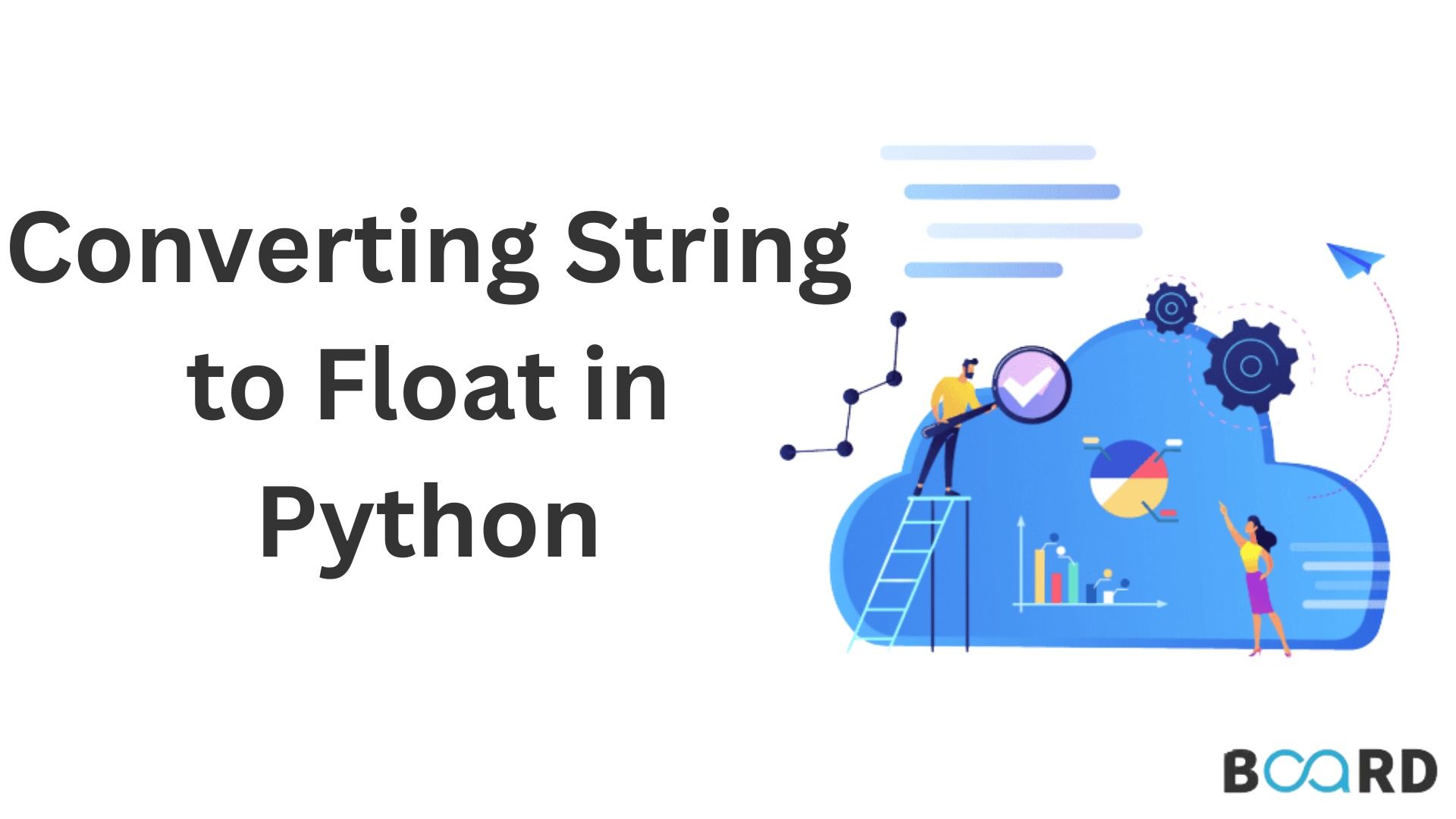
Introduction
We examine how to change a string object into a floating object in this brief lesson. We examine various approaches and talk about the restrictions encountered while attempting to convert a string to a float in Python.
Why would you require a string to be converted to a float?
Python has given us methods to make it easier to change data types, which is a regular activity. The process of changing a Python string object into a floating object is one that is commonly searched for. You would encounter this most frequently while reading and interacting with files. In these situations, you would need to transform a numeric value from a string into a float or an int before taking any action.
Similar to the aforementioned example, there may be times when you need to convert a string in Python to a float.
Solution: Using the float() function
In this solution, we use the most common method to convert any data type including a string to float in Python; float().
Syntax of float()
float(x)
Parameter:
In this case, x may be an int or a string. It might contain signs, but this wouldn't change the value that was given.
Code and Explanation:
The aforementioned strings were transformed into a floating entity, as you can see. The sole requirement for the string to be converted to a float is that it must include a decimal or integer value; otherwise, the conversion will fail. Before and after using the float, you may use the type() method to verify the data types (). The object's data type is returned by this method.
The int(), str(), and float() functions can be used to convert values of different data types into integers and strings ().
Python's method for converting a string to a float has drawbacks and warnings.
- As was previously stated, if the string doesn't include a float value, float() will return a ValueError.
- OverflowError would be returned if the number was outside of a floating variable's acceptable range.
- Finally, it returns 0.0 if no value is provided as an input.