Fundamentals of Python Programming
Learn about range() in Python
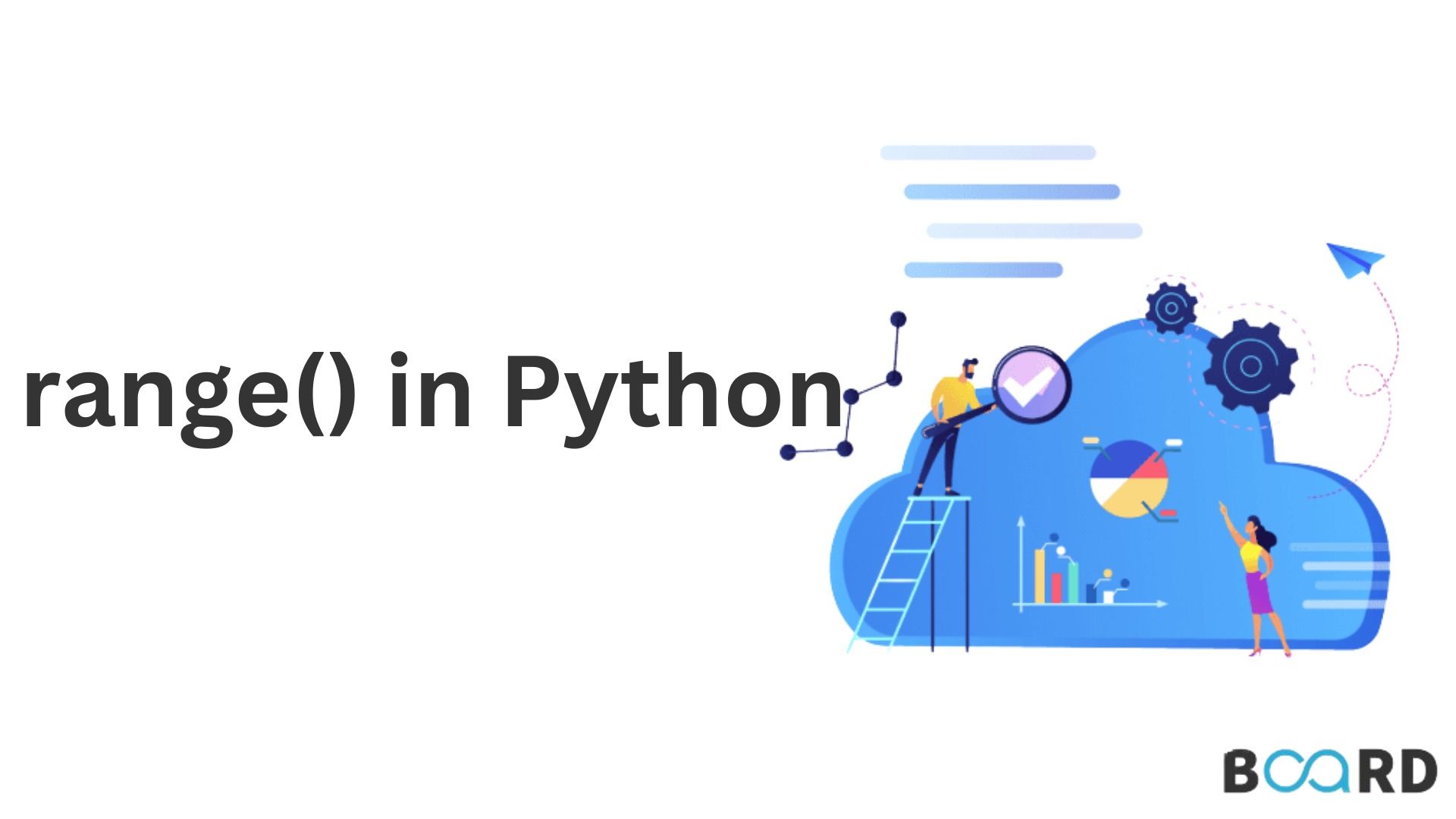
Introduction
Python's built-in function is called range(). When an action must be carried out a certain number of times, we use it. The function xrange() in Python is simply called range() in Python versions 3.x and higher. When creating a series of numbers, the range() function is helpful. We will thoroughly examine the range function.
Knowing how to utilize range function ()—which is frequently used for looping—is essential when working with any kind of Python code. Python's range() function is most frequently used to iterate over sequence types (such as strings and lists) using for and while loops.
Python range() Basics
Simply said, range() enables users to generate a sequence of integers that fall inside a predetermined range. The user may choose the beginning and ending points of the series of integers as well as the amount of difference between each number by varying the number of parameters passed to the function. The three arguments that range() typically accepts are as follows:
- start (1 argument): Integer starting point from which to reverse the series of integers
- stop (2 arguments): Before which the order of the integers should be reversed. The integer range ends at stop minus 1.
- step (3 arguments): An integer value that specifies the amount by which each integer in the order will increase.
range(stop)
When we call range() with just one argument, we will receive a list of numbers that starts at 0 and includes all whole numbers up to but excluding the stop number that we have specified.
range(start, stop)
We don't always have to start at "0" when calling range() with two arguments since we may specify where the series of integers starts and terminates. Making a sequence of numbers from 'X' to 'Y' using range() is possible (X, Y).
range(start, stop, step)
With three arguments, range() allows us to specify not only the beginning and ending points of the number sequence but also the size of the gap between each number.
Using the float Numbers in the Python range()
The float numbers are not supported by the range() method in Python. In other words, we are not allowed to use a floating-point or non-integer value as one of its parameters. We are limited to using only integers.
FAQs related to the range function
1. What actions does a range () function perform?
-> The range() function reverses a set of numbers, starting by default at "0," increasing by "1," and stopping before a specified number.
2. What is the range actually for?
-> Actually, the range is the difference between the lowest and highest numbers. For instance: The lowest value in 4, 5, 9, 3, and 8 is "3," and the highest value is "9." The range will therefore be 9 x 3 = 6.